Option Strategies
Short Put Calendar Spread
Introduction
Put calendar spread, also known as put horizontal spread, is a combination of a longer-term (far-leg/front-month) put and a shorter-term (near-leg/back-month) put, where both puts have the same underlying stock and the same strike price. The short put calendar spread consists of selling a longer-term put and buying a shorter-term put. This strategy profits from an increase in price movement.
Implementation
Follow these steps to implement the short put calendar spread strategy:
- In the
initialize
method, set the start date, end date, cash, and Option universe. - In the
on_data
method, select the strike price and expiration dates of the contracts in the strategy legs. - In the
on_data
method, select the contracts and place the orders.
def initialize(self) -> None: self.set_start_date(2017, 2, 1) self.set_end_date(2017, 2, 19) self.set_cash(500000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().put_calendar_spread(0, 30, 60))
The put_calendar_spread
filter narrows the universe down to just the two contracts you need to form a short put calendar spread.
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Get the ATM strike atm_strike = sorted(chain, key=lambda x: abs(x.strike - chain.underlying.price))[0].strike # Select the ATM put Option contracts puts = [i for i in chain if i.strike == atm_strike and i.right == OptionRight.PUT] if len(puts) == 0: return # Select the near and far expiry dates expiries = sorted([x.expiry for x in puts]) near_expiry = expiries[0] far_expiry = expiries[-1]
Approach A: Call the OptionStrategies.short_put_calendar_spread
method with the details of each leg and then pass the result to the buy
method.
option_strategy = OptionStrategies.short_put_calendar_spread(self._symbol, atm_strike, near_expiry, far_expiry) self.buy(option_strategy, 1)
Approach B: Create a list of Leg
objects and then call the combo_market_order, combo_limit_order, or combo_leg_limit_order method.
near_expiry_put = [x for x in puts if x.expiry == near_expiry][0] far_expiry_put = [x for x in puts if x.expiry == far_expiry][0] legs = [ Leg.create(near_expiry_put.symbol, 1), Leg.create(far_expiry_put.symbol, -1) ] self.combo_market_order(legs, 1)
Strategy Payoff
The short put calendar spread is a limited-reward-limited-risk strategy. The payoff is taken at the shorter-term expiration. The payoff is
Pshort-termT=(K−ST)+PT=(Pshort-termT−Plong-termT+Plong-term0−Pshort-term0)×m−fee wherePshort-termT=Shorter term put value at time TPlong-termT=Longer term put value at time TST=Underlying asset price at time TK=Strike pricePT=Payout total at time TPshort-term0=Shorter term put value at position opening (debit paid)Plong-term0=Longer term put value at position opening (credit received)m=Contract multiplierT=Time of shorter term put expirationThe following chart shows the payoff at expiration:
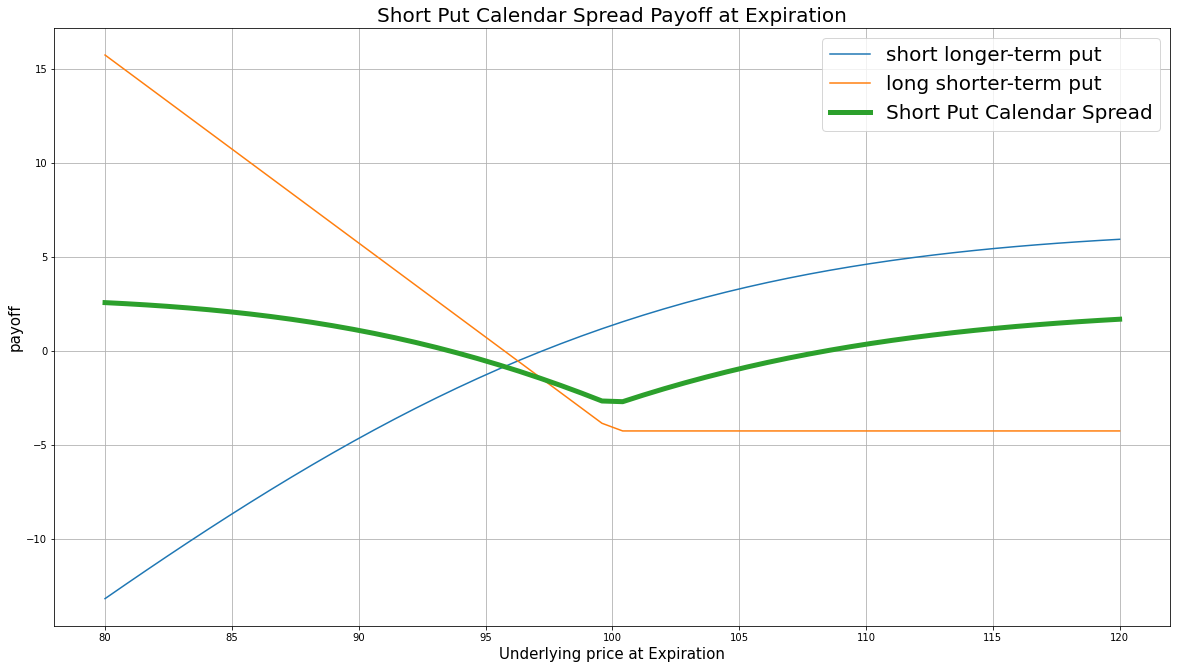
The maximum profit is the net credit received, Plong-term0−Pshort-term0. It occurs when the underlying price moves very deep ITM or OTM so the values of both puts are close to zero.
The maximum loss is undetermined because it depends on the underlying volatility. It occurs when ST=S0 and the spread of the 2 puts are at their maximum.
If the Option is American Option, there is a risk of early assignment on the contract you sell. Additionally, if you don't close the put positions together, the naked short put will have unlimited drawdown risk after the long put expires.
Example
The following table shows the price details of the assets in the short put calendar spread algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
Shorter-term put at position opening | 11.30 | 800.00 |
Longer-term put at position opening | 19.30 | 800.00 |
Longer-term put at shorter-term expiration | 3.50 | 800.00 |
Underlying Equity at shorter-term expiration | 828.07 | - |
Therefore, the payoff is
Pshort-termT=(K−ST)+=(800.00−828.07)+=0PT=(−Plong-termT+Pshort-termT−Pshort-term0+Plong-term0)×m−fee=(−3.50+0−11.30+19.30)×100−1.00×2=448So, the strategy gains $448.
The following algorithm implements a short put calendar spread Option strategy:
class PutCalendarSpreadStrategy(QCAlgorithm): def initialize(self) -> None: self.set_start_date(2017, 2, 1) self.set_end_date(2017, 2, 20) self.set_cash(500000) option = self.add_option("GOOG", Resolution.MINUTE) self.symbol = option.symbol option.set_filter(self.universe_func) def universe_func(self, universe: OptionFilterUniverse) -> OptionFilterUniverse: return universe.include_weeklys().put_calendar_spread(0, 30, 60) def on_data(self, data) -> None: # avoid extra orders if self.portfolio.invested: return # Get the OptionChain of the self.symbol chain = data.option_chains.get(self.symbol, None) if not chain: return # get at-the-money strike atm_strike = sorted(chain, key=lambda x: abs(x.strike - chain.underlying.price))[0].strike # filter the put options from the contracts which is ATM in the option chain. puts = [i for i in chain if i.strike == atm_strike and i.right == OptionRight.PUT] if len(puts) == 0: return # sorted the optionchain by expiration date expiries = sorted([x.expiry for x in puts], key = lambda x: x) # select the farest expiry as far-leg expiry, and the nearest expiry as near-leg expiry near_expiry = expiries[0] far_expiry = expiries[-1] option_strategy = OptionStrategies.short_put_calendar_spread(self.symbol, atm_strike, near_expiry, far_expiry) # We open a position with 1 unit of the option strategy self.buy(option_strategy, 1) def on_end_of_algorithm(self) -> None: for symbol, sec in self.securities.items(): self.log(f"{symbol} :: {sec.price}")
Other Examples
For more examples, see the following algorithms: