US Equity
Handling Data
Introduction
LEAN passes the data you request to the OnData
on_data
method so you can make trading decisions. The default OnData
on_data
method accepts a Slice
object, but you can define additional OnData
on_data
methods that accept different data types. For example, if you define an OnData
on_data
method that accepts a TradeBar
argument, it only receives TradeBar
objects. The Slice
object that the OnData
on_data
method receives groups all the data together at a single moment in time. To access the Slice
outside of the OnData
on_data
method, use the CurrentSlice
current_slice
property of your algorithm.
All the data formats use DataDictionary
objects to group data by Symbol
and provide easy access to information. The plural of the type denotes the collection of objects. For instance, the TradeBars
DataDictionary
is made up of TradeBar
objects. To access individual data points in the dictionary, you can index the dictionary with the security ticker or Symbol
symbol
, but we recommend you use the Symbol
symbol
.
To view the resolutions that are available for US Equity data, see Resolutions.
Trades
TradeBar
objects are price bars that consolidate individual trades from the exchanges. They contain the open, high, low, close, and volume of trading activity over a period of time.
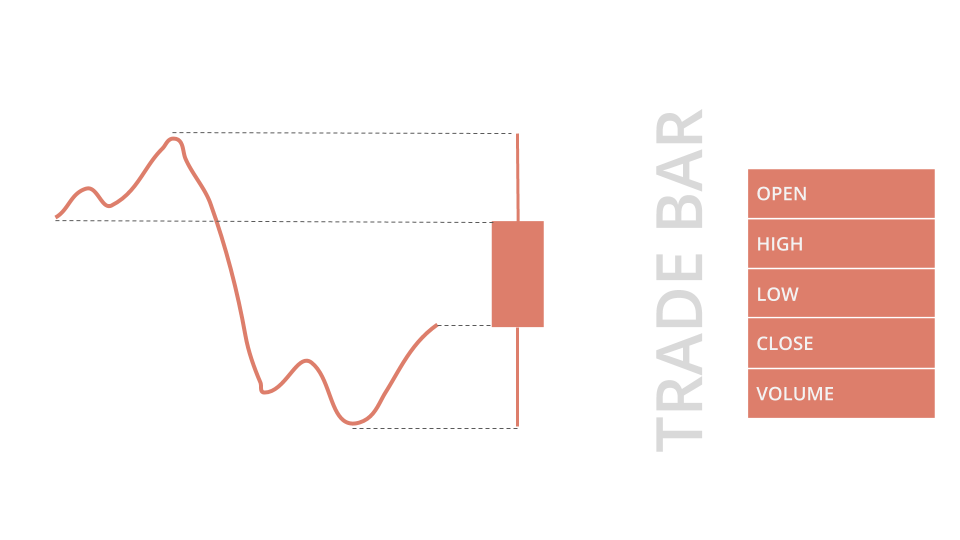
To get the TradeBar
objects in the Slice
, index the Slice
or index the Bars
bars
property of the Slice
with the security Symbol
symbol
. If the security doesn't actively trade or you are in the same time step as when you added the security subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your security before you index the Slice
with the security Symbol
symbol
.
public override void OnData(Slice slice) { // Check if the symbol is contained in TradeBars object if (slice.Bars.ContainsKey(_symbol)) { // Obtain the mapped TradeBar of the symbol var tradeBar = slice.Bars[_symbol]; } }
def on_data(self, slice: Slice) -> None: # Obtain the mapped TradeBar of the symbol if any trade_bar = slice.bars.get(self._symbol) # None if not found
You can also iterate through the TradeBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the TradeBar
objects.
public override void OnData(Slice slice) { // Iterate all received Symbol-TradeBar key-value pairs foreach (var kvp in slice.Bars) { var symbol = kvp.Key; var tradeBar = kvp.Value; var closePrice = tradeBar.Close; } }
def on_data(self, slice: Slice) -> None: # Iterate all received Symbol-TradeBar key-value pairs for symbol, trade_bar in slice.bars.items(): close_price = trade_bar.close
TradeBar
objects have the following properties:
We adjust the daily open and close price of bars to reflect the official auction prices.
Quotes
QuoteBar
objects are bars that consolidate NBBO quotes from the exchanges. They contain the open, high, low, and close prices of the bid and ask. The Open
open
, High
high
, Low
low
, and Close
close
properties of the QuoteBar
object are the mean of the respective bid and ask prices. If the bid or ask portion of the QuoteBar
has no data, the Open
open
, High
high
, Low
low
, and Close
close
properties of the QuoteBar
copy the values of either the Bid
bid
or Ask
ask
instead of taking their mean.
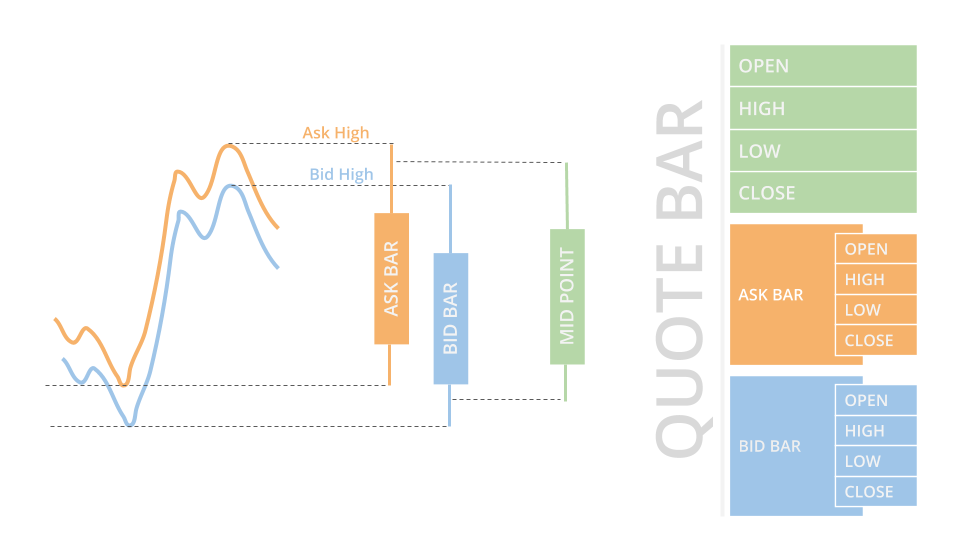
To get the QuoteBar
objects in the Slice
, index the QuoteBars
property of the Slice
with the security Symbol
symbol
. If the security doesn't actively get quotes or you are in the same time step as when you added the security subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your security before you index the Slice
with the security Symbol
symbol
.
public override void OnData(Slice slice) { // Check if the symbol is contained in QuoteBars object if (slice.QuoteBars.ContainsKey(_symbol)) { // Obtain the mapped QuoteBar of the symbol var quoteBar = slice.QuoteBars[_symbol]; } }
def on_data(self, slice: Slice) -> None: # Obtain the mapped QuoteBar of the symbol if any quote_bar = slice.quote_bars.get(self._symbol) # None if not found
You can also iterate through the QuoteBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the QuoteBar
objects.
public override void OnData(Slice slice) { // Iterate all received Symbol-QuoteBar key-value pairs foreach (var kvp in slice.QuoteBars) { var symbol = kvp.Key; var quoteBar = kvp.Value; var askPrice = quoteBar.Ask.Close; } }
def on_data(self, slice: Slice) -> None: # Iterate all received Symbol-QuoteBar key-value pairs for symbol, quote_bar in slice.quote_bars.items(): ask_price = quote_bar.ask.close
QuoteBar
objects let LEAN incorporate spread costs into your simulated trade fills to make backtest results more realistic.
QuoteBar
objects have the following properties:
Ticks
Tick
objects represent a single trade or quote at a moment in time. A trade tick is a record of a transaction for the security. A quote tick is an offer to buy or sell the security at a specific price.
Trade ticks have a non-zero value for the Quantity
quantity
and Price
price
properties, but they have a zero value for the BidPrice
bid_price
, BidSize
bid_size
, AskPrice
ask_price
, and AskSize
ask_size
properties. Quote ticks have non-zero values for BidPrice
bid_price
and BidSize
bid_size
properties or have non-zero values for AskPrice
ask_price
and AskSize
ask_size
properties. To check if a tick is a trade or a quote, use the TickType
ticktype
property.
In backtests, LEAN groups ticks into one millisecond buckets. In live trading, LEAN groups ticks into ~70-millisecond buckets. To get the Tick
objects in the Slice
, index the Ticks
property of the Slice
with a Symbol
symbol
. If the security doesn't actively trade or you are in the same time step as when you added the security subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your security before you index the Slice
with the security Symbol
symbol
.
public override void OnData(Slice slice) { if (slice.Ticks.ContainsKey(_symbol)) { var ticks = slice.Ticks[_symbol]; foreach (var tick in ticks) { var price = tick.Price; } } }
def on_data(self, slice: Slice) -> None: ticks = slice.ticks.get(self._symbol, []) # Empty if not found for tick in ticks: price = tick.price
You can also iterate through the Ticks
dictionary. The keys of the dictionary are the Symbol
objects and the values are the List<Tick>
list[Tick]
objects.
public override void OnData(Slice slice) { foreach (var kvp in slice.Ticks) { var symbol = kvp.Key; var ticks = kvp.Value; foreach (var tick in ticks) { var price = tick.Price; } } }
def on_data(self, slice: Slice) -> None: for symbol, ticks in slice.ticks.items(): for tick in ticks: price = tick.price
Tick data is raw and unfiltered, so it can contain bad ticks that skew your trade results. For example, some ticks come from dark pools, which aren't tradable. We recommend you only use tick data if you understand the risks and are able to perform your own online tick filtering.
Tick
objects have the following properties:
If we detect a tick that may be suspicious, we set its Suspicious
suspicious
flag to true.
Other Data Formats
For more information about data formats available for US Equities, see Corporate Actions.
Examples
The following examples demonstrate some common practices for handling US Equity data.
Example 1: Various Data Resolutions and Formats
The following algorithm handles three data resolutions (Daily, Minute, and Tick) and three data formats (TradeBar, QuoteBar, and Tick). The Equity subscriptions provide the ticks and daily TradeBar objects. To create the 5-minute QuoteBar objects, it consolidates the AAPL ticks.
The algorithm enters a long position in AAPL when the following conditions are met:
- The 16-day Fractal Adaptive Moving Average (FRAMA) of QQQ is increasing, which indicates the trend is up.
- The Relative Strength Index (RSI) of AAPL is above 70, which indicates AAPL has been rising from large buying pressure.
The algorithm enters a short position in AAPL when the following conditions are met:
- The 16-day FRAMA of QQQ is rising, which indicates the trend is down.
- The RSI of AAPL is above, which indicates AAPL has been declining from large selling pressure.
The algorithm liquidates the portfolio when the FRAMA changes direction.
public class USEquityExampleAlgorithm : QCAlgorithm { private dynamic _qqq, _aapl; public override void Initialize() { SetStartDate(2020, 1, 1); SetEndDate(2020, 2, 1); // Add QQQ and AAPL data. _qqq = AddEquity("QQQ", Resolution.Daily); _aapl = AddEquity("AAPL", Resolution.Tick); // Create the indicators. _qqq.Frama = FRAMA(_qqq.Symbol, 16); _aapl.Rsi = new RelativeStrengthIndex(10); // Create a 5-minute consolidator to aggregate AAPL tick data into quote bars. var consolidator = new TickQuoteBarConsolidator(TimeSpan.FromMinutes(5)); // Subscribe the consolidator for automatic updates. SubscriptionManager.AddConsolidator(_aapl.Symbol, consolidator); // Attach an event handler to update the RSI indicator with the consolidated bars. consolidator.DataConsolidated += (sender, bar) => _aapl.Rsi.Update(bar.EndTime, bar.Close); // Warm up the indicators. WarmUpIndicator(_qqq.Symbol, _qqq.Frama); var history = History<QuoteBar>(_aapl.Symbol, 10, Resolution.Minute); foreach (var bar in history) { _aapl.Rsi.Update(bar.EndTime, bar.Close); } } public override void OnData(Slice slice) { // Ensure AAPL ticks are in the current slice. if (!slice.Ticks.ContainsKey(_aapl.Symbol)) { return; } // Get the indicator values. var frama = _qqq.Frama.Current.Value; var prevFrama = _qqq.Frama.Previous.Value; var rsi = _aapl.Rsi.Current.Value; // Check if we have an AAPL position. if (_aapl.Holdings.Invested) { // If there is divergence between our position and the Frama direction, exit the position. if ((_aapl.Holdings.IsLong && frama < prevFrama) || (_aapl.Holdings.IsShort && frama > prevFrama)) { Liquidate(_aapl.Symbol); } } // Look for a long entry. else if (frama > prevFrama && rsi >= 70) { SetHoldings(_aapl.Symbol, 0.5); } // Look for a short entry. else if (frama < prevFrama && rsi <= 30) { SetHoldings(_aapl.Symbol, -0.5); } } }
class USEquityExampleAlgorithm(QCAlgorithm): def initialize(self) -> None: self.set_start_date(2020, 1, 1) self.set_end_date(2020, 2, 1) # Add QQQ and AAPL data. self._qqq = self.add_equity("QQQ", Resolution.DAILY) self._aapl = self.add_equity("AAPL", Resolution.TICK) # Create the indicators. self._qqq.frama = self.frama(self._qqq.symbol, 16) self._aapl.rsi = RelativeStrengthIndex(10) # Create a 5-minute consolidator to aggregate AAPL tick data into quote bars. consolidator = TickQuoteBarConsolidator(timedelta(minutes=5)) # Subscribe the consolidator for automatic updates. self.subscription_manager.add_consolidator(self._aapl.symbol, consolidator) # Attach an event handler handler to update the RSI indicator with the consolidated bars. consolidator.data_consolidated += lambda _, bar: self._aapl.rsi.update(bar.end_time, bar.close) # Warm up the indicators. self.warm_up_indicator(self._qqq.symbol, self._qqq.frama) for quote_bar in self.history[QuoteBar](self._aapl.symbol, 10, Resolution.MINUTE): self._aapl.rsi.update(quote_bar.end_time, quote_bar.close) def on_data(self, slice: Slice) -> None: # Ensure AAPL ticks are in the current slice. if self._aapl.symbol not in slice.ticks: return # Get the indicator values. frama = self._qqq.frama.current.value prev_frama = self._qqq.frama.previous.value rsi = self._aapl.rsi.current.value # Check if we have an AAPL position. if self._aapl.holdings.invested: # If there is divergence between our position and the Frama direction, exit the position. if (self._aapl.holdings.is_long and frama < prev_frama or self._aapl.holdings.is_short and frama > prev_frama): self.liquidate(self._aapl.symbol) # Look for a long entry. elif frama > prev_frama and rsi >= 70: self.set_holdings(self._aapl.symbol, 0.5) # Look for a short entry. elif frama < prev_frama and rsi <= 30: self.set_holdings(self._aapl.symbol, -0.5)