Option Strategies
Protective Collar
Introduction
A Protective Collar is an Options strategy that consists of a covered call and a long put (protective put) with a lower strike price than the short call contract. In contrast to the covered call, the protective put component limits the drawdown of the strategy when the underlying price decreases too much.
Implementation
Follow these steps to implement the protective collar strategy:
- In the
initialize
method, set the start date, set the end date, subscribe to the underlying Equity, and create an Option universe. - In the
on_data
method, select the Option contracts. - In the
on_data
method, select the contracts and place the orders.
def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 30) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().protective_collar(30, -1, -10))
The protective_collar
filter narrows the universe down to just the two contracts you need to form a protective collar.
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select an expiry date expiry = max([x.expiry for x in chain]) # Select the call and put contracts that expire on the selected date calls = [x for x in chain if x.right == OptionRight.CALL and x.expiry == expiry] puts = [x for x in chain if x.right == OptionRight.PUT and x.expiry == expiry] if not calls or not puts: return # Select the OTM contracts call = sorted(calls, key = lambda x: x.strike)[-1] put = sorted(puts, key = lambda x: x.strike)[0]
Approach A: Call the OptionStrategies.protective_collar
method with the details of each leg and then pass the result to the buy
method.
protective_collar = OptionStrategies.protective_collar(self._symbol, call.strike, put.strike, expiry) self.buy(protective_collar, 1)
Approach B: Create a list of Leg
objects and then call the combo_market_order, combo_limit_order, or combo_leg_limit_order method.
legs = [ Leg.create(call.symbol, -1), Leg.create(put.symbol, 1), Leg.create(chain.underlying.symbol, chain.underlying.symbol_properties.contract_multiplier) ] self.combo_market_order(legs, 1)
Strategy Payoff
This is a limited-profit-limited-loss strategy. The payoff is
CT=(ST−KC)+PT=(KP−ST)+PayoffT=(ST−S0−CT+PT+C0−P0)×m−fee whereCT=Call value at time TPT=Put value at time TST=Underlying asset price at time TKC=Call strike priceKP=Put strike pricePayoffT=Payout total at time TS0=Underlying asset price when the trade openedC0=Call price when the trade opened (credit received)P0=Put price when the trade opened (debit paid)m=Contract multiplierT=Time of expirationThe following chart shows the payoff at expiration:
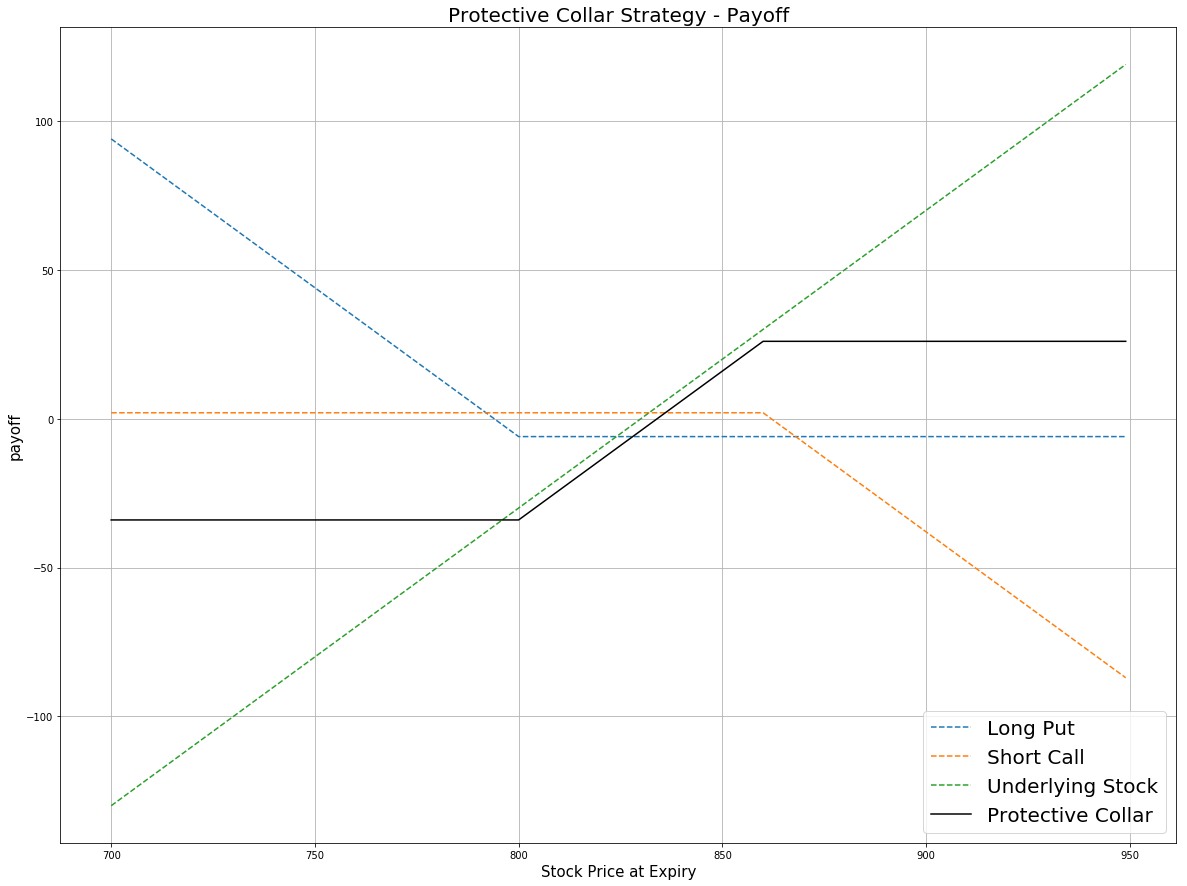
The maximum profit is KC−ST+C0−P0. It occurs when the underlying price is at or above the strike price of the call at expiration.
The maximum profit is ST−KP+C0−P0. It occurs when the underlying price is at or below the strike price of the put at expiration.
If the Option is American Option, there is a risk of early assignment on the contract you sell.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
Call | 2.85 | 845.00 |
Put | 6.00 | 822.50 |
Underlying Equity at position opens | 833.17 | - |
Underlying Equity at expiration | 843.25 | - |
Therefore, the payoff is
CT=(ST−KC)+=(843.365−845.00)+=0PT=(KP−ST)+=(822.50−843.365)+=0PayoffT=(ST−S0−CT+PT+C0−P0)×m−fee=(843.25−833.17−0+0+2.85−6.00)×100−1.00×3=690So, the strategy gains $690.
The following algorithm implements a protective collar Option strategy:
class ProtectiveCollarOptionStrategy(QCAlgorithm): def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 23) self.set_cash(100000) equity = self.add_equity("GOOG", Resolution.MINUTE) option = self.add_option("GOOG", Resolution.MINUTE) self.symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().protective_collar(30, -1, -10)) def on_data(self, data: Slice) -> None: # avoid extra orders if self.portfolio.invested: return # Get the OptionChain of the self.symbol chain = data.option_chains.get(self.symbol, None) if not chain: return # choose the furthest expiration date within 30 days from now on expiry = sorted(chain, key = lambda x: x.expiry)[-1].expiry # filter the call options contracts call = [x for x in chain if x.right == OptionRight.CALL and x.expiry == expiry] # filter the put options contracts put = [x for x in chain if x.right == OptionRight.PUT and x.expiry == expiry] if not call or not put: return # select the strike prices of call and put contracts call_strike = sorted(call, key = lambda x: x.strike)[-1].strike put_strike = sorted(put, key = lambda x: x.strike)[0].strike protective_collar = OptionStrategies.protective_collar(self.symbol, call_strike, put_strike, expiry) self.buy(protective_collar, 1) def on_end_of_day(self, symbol): if symbol.value == "GOOG": self.log(f"{self.time}::{symbol}::{self.securities[symbol].price}")