Option Strategies
Long Iron Butterfly
Introduction
The Long Iron Butterfly is an option strategy which involves four Option contracts. All the contracts have the same underlying stock and expiration, but the order of strike prices for the four contracts is A>B>C. The following table describes the strike price of each contract:
Position | Strike |
---|---|
1 OTM call | A |
-1 ATM call | B |
-1 ATM put | B |
1 OTM put | C=B−(A−B) |
The long iron butterfly consists of selling an OTM call, selling an OTM put, buying an ATM call, and buying an ATM put. This strategy profits from a decrease in price movement (implied volatility).
Implementation
Follow these steps to implement the long iron butterfly strategy:
- In the
initialize
method, set the start date, end date, cash, and Option universe. - In the
on_data
method, select the contracts in the strategy legs. - In the
on_data
method, select the contracts and place the orders.
def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 5, 10) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().iron_butterfly(30, 5));
The iron_butterfly
filter narrows the universe down to just the four contracts you need to form a long iron butterfly.
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select expiry expiry = max([x.expiry for x in chain]) # Separate the call and put contracts calls = [i for i in chain if i.right == OptionRight.CALL and i.expiry == expiry] puts = [i for i in chain if i.right == OptionRight.PUT and i.expiry == expiry] if not calls or not puts: return # Get the ATM and OTM strike prices atm_strike = sorted(calls, key = lambda x: abs(chain.underlying.price - x.strike))[0].strike otm_put_strike = min([x.strike for x in puts]) otm_call_strike = 2 * atm_strike - otm_put_strike
Approach A: Call the OptionStrategies.iron_butterfly
method with the details of each leg and then pass the result to the buy
method.
iron_butterfly = OptionStrategies.iron_butterfly(self._symbol, otm_put_strike, atm_strike, otm_call_strike, expiry) self.buy(iron_butterfly, 2)
Approach B: Create a list of Leg
objects and then call the combo_market_order, combo_limit_order, or combo_leg_limit_order method.
# Select the contracts atm_call = [x for x in calls if x.strike == atm_strike][0] atm_put = [x for x in puts if x.strike == atm_strike][0] otm_call = [x for x in calls if x.strike == otm_call_strike][0] otm_put = [x for x in puts if x.strike == otm_put_strike][0] legs = [ Leg.create(atm_call.symbol, -1), Leg.create(atm_put.symbol, -1), Leg.create(otm_call.symbol, 1), Leg.create(otm_put.symbol, 1) ] self.combo_market_order(legs, 1)
Strategy Payoff
The long call butterfly is a limited-reward-limited-risk strategy. The payoff is
COTMT=(ST−KCOTM)+CATMT=(ST−KCATM)+POTMT=(KPOTM−ST)+PATMT=(KPATM−ST)+PT=(COTMT+POTMT−CATMT−PATMT−COTM0−POTM0+CATM0+PATM0)×m−fee whereCOTMT=OTM call value at time TCATMT=ATM call value at time TPOTMT=OTM put value at time TPATMT=ATM put value at time TST=Underlying asset price at time TKCOTM=OTM call strike priceKCATM=ATM call strike priceKPOTM=OTM put strike priceKPATM=ATM put strike pricePT=Payout total at time TCOTM0=OTM call value at position opening (debit paid)CATM0=ATM call value at position opening (credit received)POTM0=OTM put value at position opening (debit paid)PATM0=ATM put value at position opening (credit received)m=Contract multiplierT=Time of expirationThe following chart shows the payoff at expiration:
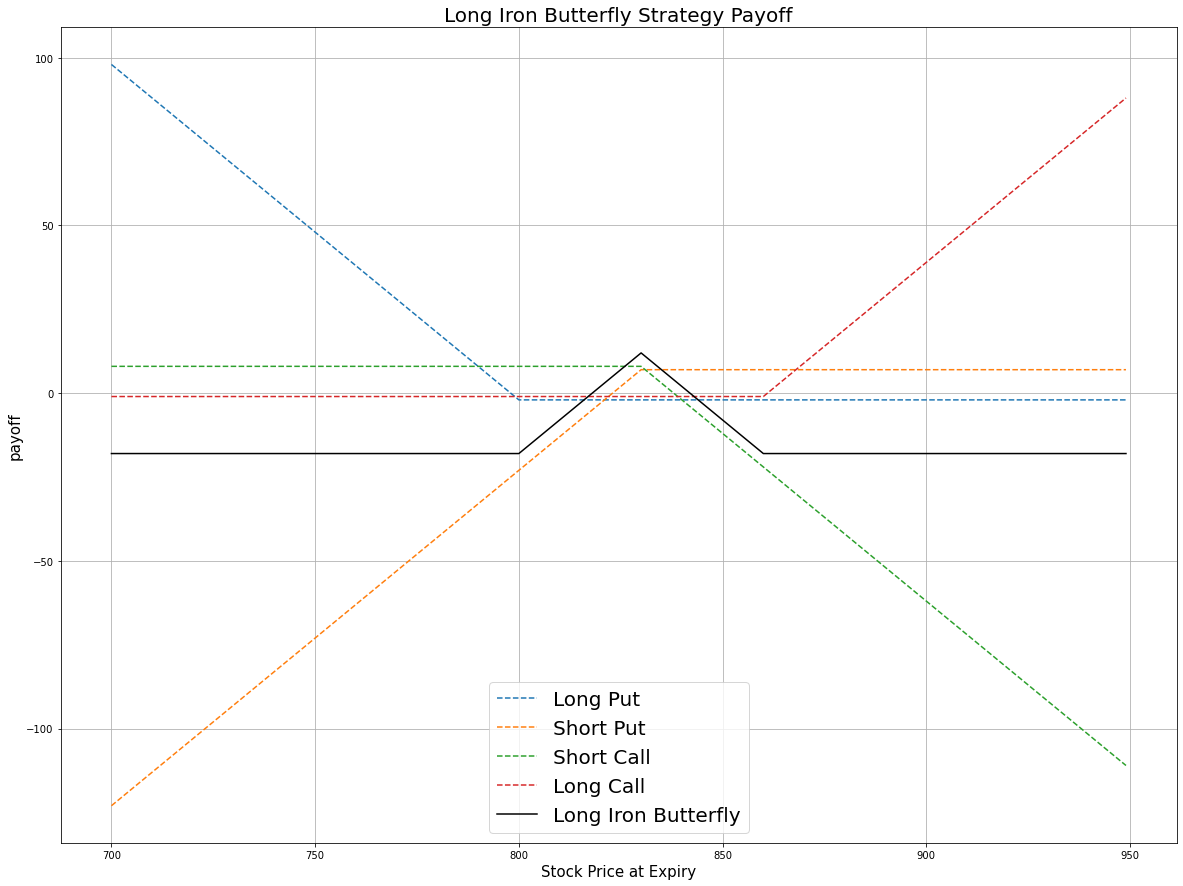
The maximum profit is the net credit received, CATM0+PATM0−COTM0−POTM0. It occurs when the underlying price stays the same as when you opened the trade.
The maximum loss is KCOTM−KCATM−CATM0−PATM0+COTM0+POTM0. It occurs when the underlying price is below the OTM put strike price or above the OTM call strike price at expiration.
If the Option is American Option, there is a risk of early assignment on the contracts you sell.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
OTM call | 1.35 | 855.00 |
OTM put | 1.50 | 810.00 |
ATM call | 10.30 | 832.50 |
ATM put | 9.50 | 832.50 |
Underlying Equity at expiration | 843.25 | - |
Therefore, the payoff is
COTMT=(ST−KCOTM)+=(843.25−855.00)+=0CATMT=(ST−KCATM)+=(843.25−832.50)+=10.75POTMT=(KPOTM−ST)+=(810.00−843.25)+=0PATMT=(KPATM−ST)+=(832.50.00−843.25)+=0PT=(CATMT+PATMT−COTMT−POTMT−CATM0−PATM0+COTM0+POTM0)×m−fee=(10.75+0−0−0−10.30−9.50+1.35+1.50)×100−1×4=−624So, the strategy losses $624.
The following algorithm implements a long iron butterfly Option strategy:
class LongButteflyOptionStrategy(QCAlgorithm): def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 23) self.set_cash(100000) option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol # set our strike/expiry filter for this option chain option.set_filter(lambda x: x.include_weeklys().iron_butterfly(30, 5)) def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select expiry expiry = max([x.expiry for x in chain]) # Separate the call and put contracts calls = [i for i in chain if i.right == OptionRight.CALL and i.expiry == expiry] puts = [i for i in chain if i.right == OptionRight.PUT and i.expiry == expiry] if not calls or not puts: return # Get the ATM and OTM strike prices atm_strike = sorted(calls, key = lambda x: abs(x.strike - chain.underlying.price))[0].strike otm_put_strike = min([x.strike for x in puts]) otm_call_strike = 2 * atm_strike - otm_put_strike iron_butterfly = OptionStrategies.iron_butterfly(self._symbol, otm_put_strike, atm_strike, otm_call_strike, expiry) self.buy(iron_butterfly, 1)