Futures
Handling Data
Introduction
LEAN passes the data you request to the on_data
method so you can make trading decisions. The Slice
object that the on_data
method receives groups all the data together at a single moment in time. To access the Slice
outside of the on_data
method, use the current_slice
property of your algorithm.
All the data formats use DataDictionary
objects to group data by Symbol
and provide easy access to information. The plural of the type denotes the collection of objects. For instance, the TradeBars
DataDictionary
is made up of TradeBar
objects. To access individual data points in the dictionary, you can index the dictionary with the contract ticker or symbol
, but we recommend you use the symbol
.
To view the resolutions that are available for Futures data, see Resolutions.
Trades
TradeBar
objects are price bars that consolidate individual trades from the exchanges. They contain the open, high, low, close, and volume of trading activity over a period of time.
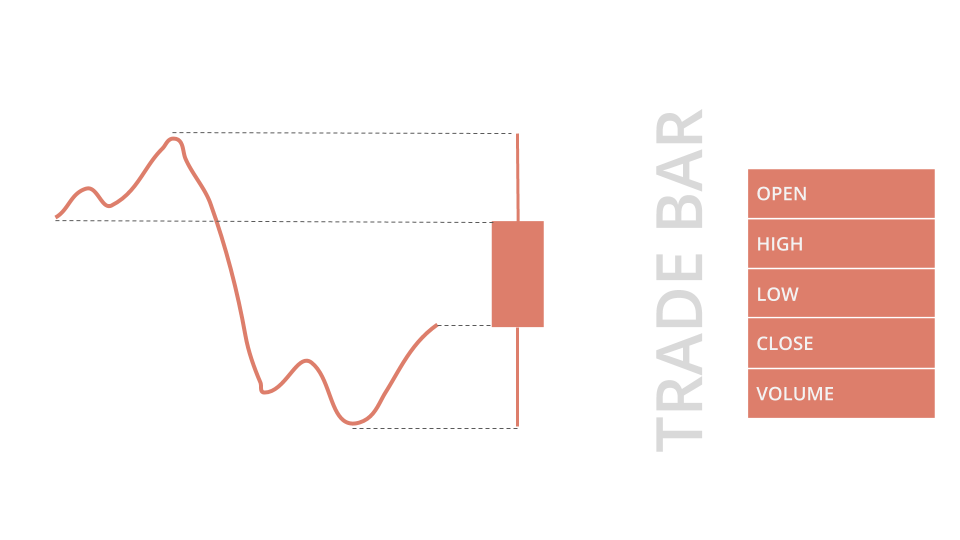
To get the TradeBar
objects in the Slice
, index the Slice
or index the bars
property of the Slice
with the contract symbol
. If the contract doesn't actively trade or you are in the same time step as when you added the contract subscription, the Slice
may not contain data for your symbol
. To avoid issues, check if the Slice
contains data for your contract before you index the Slice
with the contract symbol
.
def on_data(self, slice: Slice) -> None: # Obtain the mapped TradeBar of the symbol if any trade_bar = slice.bars.get(self._contract_symbol) # None if not found
You can also iterate through the TradeBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the TradeBar
objects.
def on_data(self, slice: Slice) -> None: # Iterate all received Symbol-TradeBar key-value pairs for symbol, trade_bar in slice.bars.items(): close_price = trade_bar.close
TradeBar
objects have the following properties:
Quotes
QuoteBar
objects are bars that consolidate NBBO quotes from the exchanges. They contain the open, high, low, and close prices of the bid and ask. The open
, high
, low
, and close
properties of the QuoteBar
object are the mean of the respective bid and ask prices. If the bid or ask portion of the QuoteBar
has no data, the open
, high
, low
, and close
properties of the QuoteBar
copy the values of either the bid
or ask
instead of taking their mean.
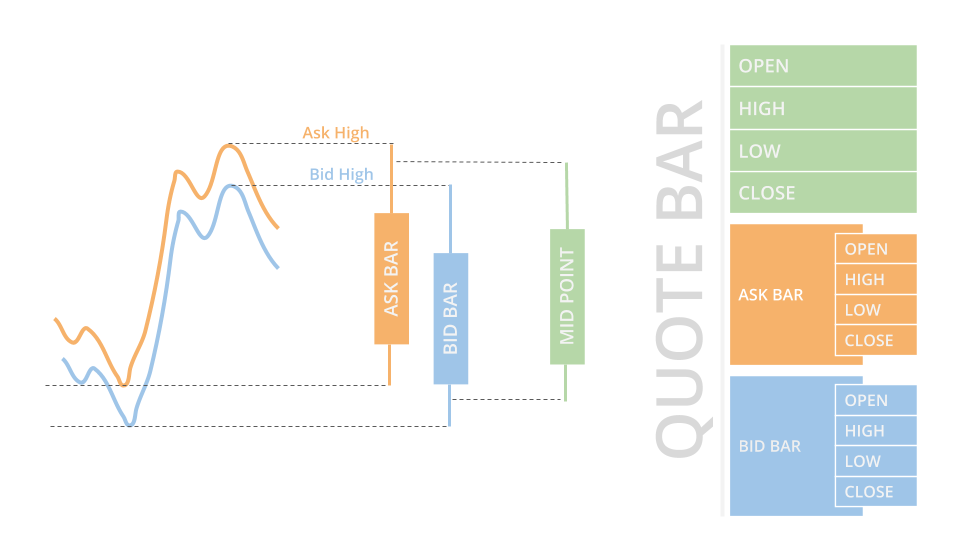
To get the QuoteBar
objects in the Slice
, index the QuoteBars
property of the Slice
with the contract symbol
. If the contract doesn't actively get quotes or you are in the same time step as when you added the contract subscription, the Slice
may not contain data for your symbol
. To avoid issues, check if the Slice
contains data for your contract before you index the Slice
with the contract symbol
.
def on_data(self, slice: Slice) -> None: # Obtain the mapped QuoteBar of the symbol if any quote_bar = slice.quote_bars.get(self._contract_symbol) # None if not found
You can also iterate through the QuoteBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the QuoteBar
objects.
def on_data(self, slice: Slice) -> None: # Iterate all received Symbol-QuoteBar key-value pairs for symbol, quote_bar in slice.quote_bars.items(): ask_price = quote_bar.ask.close
QuoteBar
objects let LEAN incorporate spread costs into your simulated trade fills to make backtest results more realistic.
QuoteBar
objects have the following properties:
Ticks
Tick
objects represent a single trade or quote at a moment in time. A trade tick is a record of a transaction for the contract. A quote tick is an offer to buy or sell the contract at a specific price.
Trade ticks have a non-zero value for the quantity
and price
properties, but they have a zero value for the bid_price
, bid_size
, ask_price
, and ask_size
properties. Quote ticks have non-zero values for bid_price
and bid_size
properties or have non-zero values for ask_price
and ask_size
properties. To check if a tick is a trade or a quote, use the ticktype
property.
In backtests, LEAN groups ticks into one millisecond buckets. In live trading, LEAN groups ticks into ~70-millisecond buckets. To get the Tick
objects in the Slice
, index the Ticks
property of the Slice
with a symbol
. If the contract doesn't actively trade or you are in the same time step as when you added the contract subscription, the Slice
may not contain data for your symbol
. To avoid issues, check if the Slice
contains data for your contract before you index the Slice
with the contract symbol
.
def on_data(self, slice: Slice) -> None: ticks = slice.ticks.get(self._contract_symbol, []) # Empty if not found for tick in ticks: price = tick.price
You can also iterate through the Ticks
dictionary. The keys of the dictionary are the Symbol
objects and the values are the list[Tick]
objects.
def on_data(self, slice: Slice) -> None: for symbol, ticks in slice.ticks.items(): for tick in ticks: price = tick.price
Tick data is raw and unfiltered, so it can contain bad ticks that skew your trade results. For example, some ticks come from dark pools, which aren't tradable. We recommend you only use tick data if you understand the risks and are able to perform your own online tick filtering.
Tick
objects have the following properties:
Futures Chains
FuturesChain
objects represent an entire chain of contracts for a single underlying Future.
To get the FuturesChain
, index the futures_chains
property of the Slice
with the continuous contract Symbol
.
def on_data(self, slice: Slice) -> None: # Try to get the FutureChain using the canonical symbol (None if no FutureChain return) chain = slice.futures_chains.get(self._contract_symbol.canonical) if chain: # Get all contracts if the FutureChain contains any member contracts = chain.contracts
You can also loop through the futures_chains
property to get each FuturesChain
.
def on_data(self, slice: Slice) -> None: # Iterate all received Canonical Symbol-FutureChain key-value pairs for continuous_contract_symbol, chain in slice.futures_chains.items(): contracts = chain.contracts
FuturesChain
objects have the following properties:
Futures Contracts
FuturesContract
objects represent the data of a single Futures contract in the market.
To get the Futures contracts in the Slice
, use the contracts
property of the FuturesChain
.
def on_data(self, slice: Slice) -> None: # Try to get the FutureChain using the canonical symbol chain = slice.future_chains.get(self._contract_symbol.canonical) if chain: # Get individual contract data (None if not contained) contract = chain.contracts.get(self._contract_symbol) if contract: price = contract.last_price
Open interest is the number of outstanding contracts that haven't been settled. It provides a measure of investor interest and the market liquidity, so it's a popular metric to use for contract selection. Open interest is calculated once per day. To get the latest open interest value, use the open_interest
property of the Future
or future_contract
.
def on_data(self, slice: Slice) -> None: # Try to get the futures_chains using the canonical symbol chain = slice.futures_chains.get(self._contract_symbol.canonical) if chain: # Get individual contract data contract = chain.contracts.get(self._contract_symbol) if contract: # Get the open interest of the selected contracts open_interest = contract.open_interest
FuturesContract
objects have the following properties:
Symbol Changes
When the continuous contract rolls over, LEAN passes a SymbolChangedEvent
to your on_data
method, which contains the old contract Symbol
and the new contract Symbol
.
To get the SymbolChangedEvent
, use the symbol_changed_events
property of the Slice
.
You can use the SymbolChangedEvent
to roll over contracts.
def on_data(self, slice: Slice) -> None: for symbol, changed_event in slice.symbol_changed_events.items(): old_symbol = changed_event.old_symbol new_symbol = changed_event.new_symbol tag = f"Rollover - Symbol changed at {self.time}: {old_symbol} -> {new_symbol}" quantity = self.portfolio[old_symbol].quantity # Rolling over: to liquidate any position of the old mapped contract and switch to the newly mapped contract self.liquidate(old_symbol, tag=tag) if quantity: self.market_order(new_symbol, quantity, tag=tag) self.log(tag)
In backtesting, the SymbolChangedEvent
occurs at midnight Eastern Time (ET). In live trading, the live data for continuous contract mapping arrives at 6/7 AM ET, so that's when it occurs.
SymbolChangedEvent
objects have the following properties:
Examples
The following examples demonstrate some common practices for handling Futures data.
Example 1: Rollovers
Spot Future is referred to as the continuous Future contract, which is usually mapped by the front month contract or the contract with the most open interest. When a contract expires or is very close to expiring, traders usually rollover from the current contract to the next contract to avoid price settlement and remain invested. The following algorithm demonstrates rolling over with limit orders .
class FutureExampleAlgorithm(QCAlgorithm): def initialize(self): # Add the E-mini Futures and set the continuous contract mapping criteria for the rollovers. self._future = self.add_future( Futures.Indices.SP_500_E_MINI, extended_market_hours=True, data_mapping_mode=DataMappingMode.OPEN_INTEREST, data_normalization_mode=DataNormalizationMode.BACKWARDS_RATIO, contract_depth_offset=0 ) self._future.set_filter(0, 182) def on_data(self, data): # Place the initial order so you can start rolling over contracts later. if not self.portfolio.invested and not self.transactions.get_open_orders(): # Buy the contract that's currently selected in the continous contract series. self.market_order(self._future.mapped, 1) # Track rollover events. def on_symbol_changed_events(self, symbol_changed_events): for symbol, changed_event in symbol_changed_events.items(): old_symbol = changed_event.old_symbol new_symbol = changed_event.new_symbol # The quantity to roll over should be consistent. quantity = self.portfolio[old_symbol].quantity # Rolling over: Liquidate the old mapped contract and switch to the newly mapped contract. tag = f"Rollover: {old_symbol} -> {new_symbol}" self.liquidate(old_symbol, tag=tag) if quantity: # Place a limit order to avoid extreme quote filling. new_contract = self.securities[new_symbol] self.limit_order( new_symbol, quantity, # To avoid warnings, round the target limit price to a price that respects # the minimum price variation for the Future. self._get_limit_price(new_contract, new_contract.price), tag=tag ) def _get_limit_price(self, security, target_limit_price, round_up=True): parameters = GetMinimumPriceVariationParameters(security, target_limit_price) pip = security.price_variation_model.get_minimum_price_variation(parameters) return (int(target_limit_price / pip) + int(round_up)) * pip