Supported Indicators
Ichimoku Kinko Hyo
Introduction
This indicator computes the Ichimoku Kinko Hyo indicator. It consists of the following main indicators: Tenkan-sen: (Highest High + Lowest Low) / 2 for the specific period (normally 9) Kijun-sen: (Highest High + Lowest Low) / 2 for the specific period (normally 26) Senkou A Span: (Tenkan-sen + Kijun-sen )/ 2 from a specific number of periods ago (normally 26) Senkou B Span: (Highest High + Lowest Low) / 2 for the specific period (normally 52), from a specific number of periods ago (normally 26)
To view the implementation of this indicator, see the LEAN GitHub repository.
Using ICHIMOKU Indicator
To create an automatic indicators for IchimokuKinkoHyo
, call the ICHIMOKU
helper method from the QCAlgorithm
class. The ICHIMOKU
method creates a IchimokuKinkoHyo
object, hooks it up for automatic updates, and returns it so you can used it in your algorithm. In most cases, you should call the helper method in the initialize
method.
class IchimokuKinkoHyoAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._ichimoku = self.ichimoku(self._symbol, 9, 26, 17, 52, 26, 26) def on_data(self, slice: Slice) -> None: if self._ichimoku.is_ready: # The current value of self._ichimoku is represented by self._ichimoku.current.value self.plot("IchimokuKinkoHyo", "ichimoku", self._ichimoku.current.value) # Plot all attributes of self._ichimoku self.plot("IchimokuKinkoHyo", "tenkan", self._ichimoku.tenkan.current.value) self.plot("IchimokuKinkoHyo", "kijun", self._ichimoku.kijun.current.value) self.plot("IchimokuKinkoHyo", "senkou_a", self._ichimoku.senkou_a.current.value) self.plot("IchimokuKinkoHyo", "senkou_b", self._ichimoku.senkou_b.current.value) self.plot("IchimokuKinkoHyo", "chikou", self._ichimoku.chikou.current.value) self.plot("IchimokuKinkoHyo", "tenkan_maximum", self._ichimoku.tenkan_maximum.current.value) self.plot("IchimokuKinkoHyo", "tenkan_minimum", self._ichimoku.tenkan_minimum.current.value) self.plot("IchimokuKinkoHyo", "kijun_maximum", self._ichimoku.kijun_maximum.current.value) self.plot("IchimokuKinkoHyo", "kijun_minimum", self._ichimoku.kijun_minimum.current.value) self.plot("IchimokuKinkoHyo", "senkou_b_maximum", self._ichimoku.senkou_b_maximum.current.value) self.plot("IchimokuKinkoHyo", "senkou_b_minimum", self._ichimoku.senkou_b_minimum.current.value) self.plot("IchimokuKinkoHyo", "delayed_tenkan_senkou_a", self._ichimoku.delayed_tenkan_senkou_a.current.value) self.plot("IchimokuKinkoHyo", "delayed_kijun_senkou_a", self._ichimoku.delayed_kijun_senkou_a.current.value) self.plot("IchimokuKinkoHyo", "delayed_maximum_senkou_b", self._ichimoku.delayed_maximum_senkou_b.current.value) self.plot("IchimokuKinkoHyo", "delayed_minimum_senkou_b", self._ichimoku.delayed_minimum_senkou_b.current.value)
The following reference table describes the ICHIMOKU
method:
ichimoku(symbol, tenkan_period, kijun_period, senkou_a_period, senkou_b_period, senkou_a_delay_period, senkou_b_delay_period, resolution=None, selector=None)
[source]Creates a new IchimokuKinkoHyo indicator for the symbol. The indicator will be automatically updated on the given resolution.
- symbol (Symbol) — The symbol whose ICHIMOKU we want
- tenkan_period (int) — The period to calculate the Tenkan-sen period
- kijun_period (int) — The period to calculate the Kijun-sen period
- senkou_a_period (int) — The period to calculate the Tenkan-sen period
- senkou_b_period (int) — The period to calculate the Tenkan-sen period
- senkou_a_delay_period (int) — The period to calculate the Tenkan-sen period
- senkou_b_delay_period (int) — The period to calculate the Tenkan-sen period
- resolution (Resolution, optional) — The resolution
- selector (Callable[IBaseData, TradeBar], optional) — Selects a value from the BaseData to send into the indicator, if null defaults to casting the input value to a TradeBar
A new IchimokuKinkoHyo indicator with the specified periods and delays
If you don't provide a resolution, it defaults to the security resolution. If you provide a resolution, it must be greater than or equal to the resolution of the security. For instance, if you subscribe to hourly data for a security, you should update its indicator with data that spans 1 hour or longer.
For more information about the selector argument, see Alternative Price Fields.
For more information about plotting indicators, see Plotting Indicators.
You can manually create a IchimokuKinkoHyo
indicator, so it doesn't automatically update. Manual indicators let you update their values with any data you choose.
Updating your indicator manually enables you to control when the indicator is updated and what data you use to update it. To manually update the indicator, call the update
method with a TradeBar
or QuoteBar
. The indicator will only be ready after you prime it with enough data.
class IchimokuKinkoHyoAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._ichimoku = IchimokuKinkoHyo(9, 26, 17, 52, 26, 26) def on_data(self, slice: Slice) -> None: bar = slice.bars.get(self._symbol) if bar: self._ichimoku.update(bar) if self._ichimoku.is_ready: # The current value of self._ichimoku is represented by self._ichimoku.current.value self.plot("IchimokuKinkoHyo", "ichimoku", self._ichimoku.current.value) # Plot all attributes of self._ichimoku self.plot("IchimokuKinkoHyo", "tenkan", self._ichimoku.tenkan.current.value) self.plot("IchimokuKinkoHyo", "kijun", self._ichimoku.kijun.current.value) self.plot("IchimokuKinkoHyo", "senkou_a", self._ichimoku.senkou_a.current.value) self.plot("IchimokuKinkoHyo", "senkou_b", self._ichimoku.senkou_b.current.value) self.plot("IchimokuKinkoHyo", "chikou", self._ichimoku.chikou.current.value) self.plot("IchimokuKinkoHyo", "tenkan_maximum", self._ichimoku.tenkan_maximum.current.value) self.plot("IchimokuKinkoHyo", "tenkan_minimum", self._ichimoku.tenkan_minimum.current.value) self.plot("IchimokuKinkoHyo", "kijun_maximum", self._ichimoku.kijun_maximum.current.value) self.plot("IchimokuKinkoHyo", "kijun_minimum", self._ichimoku.kijun_minimum.current.value) self.plot("IchimokuKinkoHyo", "senkou_b_maximum", self._ichimoku.senkou_b_maximum.current.value) self.plot("IchimokuKinkoHyo", "senkou_b_minimum", self._ichimoku.senkou_b_minimum.current.value) self.plot("IchimokuKinkoHyo", "delayed_tenkan_senkou_a", self._ichimoku.delayed_tenkan_senkou_a.current.value) self.plot("IchimokuKinkoHyo", "delayed_kijun_senkou_a", self._ichimoku.delayed_kijun_senkou_a.current.value) self.plot("IchimokuKinkoHyo", "delayed_maximum_senkou_b", self._ichimoku.delayed_maximum_senkou_b.current.value) self.plot("IchimokuKinkoHyo", "delayed_minimum_senkou_b", self._ichimoku.delayed_minimum_senkou_b.current.value)
To register a manual indicator for automatic updates with the security data, call the register_indicator
method.
class IchimokuKinkoHyoAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol self._ichimoku = IchimokuKinkoHyo(9, 26, 17, 52, 26, 26) self.register_indicator(self._symbol, self._ichimoku, Resolution.DAILY) def on_data(self, slice: Slice) -> None: if self._ichimoku.is_ready: # The current value of self._ichimoku is represented by self._ichimoku.current.value self.plot("IchimokuKinkoHyo", "ichimoku", self._ichimoku.current.value) # Plot all attributes of self._ichimoku self.plot("IchimokuKinkoHyo", "tenkan", self._ichimoku.tenkan.current.value) self.plot("IchimokuKinkoHyo", "kijun", self._ichimoku.kijun.current.value) self.plot("IchimokuKinkoHyo", "senkou_a", self._ichimoku.senkou_a.current.value) self.plot("IchimokuKinkoHyo", "senkou_b", self._ichimoku.senkou_b.current.value) self.plot("IchimokuKinkoHyo", "chikou", self._ichimoku.chikou.current.value) self.plot("IchimokuKinkoHyo", "tenkan_maximum", self._ichimoku.tenkan_maximum.current.value) self.plot("IchimokuKinkoHyo", "tenkan_minimum", self._ichimoku.tenkan_minimum.current.value) self.plot("IchimokuKinkoHyo", "kijun_maximum", self._ichimoku.kijun_maximum.current.value) self.plot("IchimokuKinkoHyo", "kijun_minimum", self._ichimoku.kijun_minimum.current.value) self.plot("IchimokuKinkoHyo", "senkou_b_maximum", self._ichimoku.senkou_b_maximum.current.value) self.plot("IchimokuKinkoHyo", "senkou_b_minimum", self._ichimoku.senkou_b_minimum.current.value) self.plot("IchimokuKinkoHyo", "delayed_tenkan_senkou_a", self._ichimoku.delayed_tenkan_senkou_a.current.value) self.plot("IchimokuKinkoHyo", "delayed_kijun_senkou_a", self._ichimoku.delayed_kijun_senkou_a.current.value) self.plot("IchimokuKinkoHyo", "delayed_maximum_senkou_b", self._ichimoku.delayed_maximum_senkou_b.current.value) self.plot("IchimokuKinkoHyo", "delayed_minimum_senkou_b", self._ichimoku.delayed_minimum_senkou_b.current.value)
The following reference table describes the IchimokuKinkoHyo
constructor:
IchimokuKinkoHyo
This indicator computes the Ichimoku Kinko Hyo indicator. It consists of the following main indicators: Tenkan-sen: (Highest High + Lowest Low) / 2 for the specific period (normally 9) Kijun-sen: (Highest High + Lowest Low) / 2 for the specific period (normally 26) Senkou A Span: (Tenkan-sen + Kijun-sen )/ 2 from a specific number of periods ago (normally 26) Senkou B Span: (Highest High + Lowest Low) / 2 for the specific period (normally 52), from a specific number of periods ago (normally 26)
get_enumerator()
Returns an enumerator that iterates through the history window.
IEnumerator[IndicatorDataPoint]
reset()
Resets this indicator to its initial state
to_detailed_string()
Provides a more detailed string of this indicator in the form of {Name} - {Value}
str
update(time, value)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- time (datetime)
- value (float)
bool
update(input)
Updates the state of this indicator with the given value and returns true if this indicator is ready, false otherwise
- input (IBaseData)
bool
chikou
The Chikou Span component of the Ichimoku indicator
The Chikou Span component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
consolidators
The data consolidators associated with this indicator if any
The data consolidators associated with this indicator if any
ISet[IDataConsolidator]
current
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the current state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
delayed_kijun_senkou_a
The Delayed Kijun Senkou A component of the Ichimoku indicator
The Delayed Kijun Senkou A component of the Ichimoku indicator
WindowIndicator[IndicatorDataPoint]
delayed_maximum_senkou_b
The Delayed Maximum Senkou B component of the Ichimoku indicator
The Delayed Maximum Senkou B component of the Ichimoku indicator
WindowIndicator[IndicatorDataPoint]
delayed_minimum_senkou_b
The Delayed Minimum Senkou B component of the Ichimoku indicator
The Delayed Minimum Senkou B component of the Ichimoku indicator
WindowIndicator[IndicatorDataPoint]
delayed_tenkan_senkou_a
The Delayed Tenkan Senkou A component of the Ichimoku indicator
The Delayed Tenkan Senkou A component of the Ichimoku indicator
WindowIndicator[IndicatorDataPoint]
is_ready
Returns true if all of the sub-components of the Ichimoku indicator is ready
Returns true if all of the sub-components of the Ichimoku indicator is ready
bool
item
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
Indexes the history windows, where index 0 is the most recent indicator value. If index is greater or equal than the current count, it returns null. If the index is greater or equal than the window size, it returns null and resizes the windows to i + 1.
IndicatorDataPoint
kijun
The Kijun-sen component of the Ichimoku indicator
The Kijun-sen component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
kijun_maximum
The Kijun-sen Maximum component of the Ichimoku indicator
The Kijun-sen Maximum component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
kijun_minimum
The Kijun-sen Minimum component of the Ichimoku indicator
The Kijun-sen Minimum component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
name
Gets a name for this indicator
Gets a name for this indicator
str
previous
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
Gets the previous state of this indicator. If the state has not been updated then the time on the value will equal DateTime.MinValue.
IndicatorDataPoint
samples
Gets the number of samples processed by this indicator
Gets the number of samples processed by this indicator
int
senkou_a
The Senkou A Span component of the Ichimoku indicator
The Senkou A Span component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
senkou_b
The Senkou B Span component of the Ichimoku indicator
The Senkou B Span component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
senkou_b_maximum
The Senkou B Maximum component of the Ichimoku indicator
The Senkou B Maximum component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
senkou_b_minimum
The Senkou B Minimum component of the Ichimoku indicator
The Senkou B Minimum component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
tenkan
The Tenkan-sen component of the Ichimoku indicator
The Tenkan-sen component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
tenkan_maximum
The Tenkan-sen Maximum component of the Ichimoku indicator
The Tenkan-sen Maximum component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
tenkan_minimum
The Tenkan-sen Minimum component of the Ichimoku indicator
The Tenkan-sen Minimum component of the Ichimoku indicator
IndicatorBase[IndicatorDataPoint]
warm_up_period
Required period, in data points, for the indicator to be ready and fully initialized.
Required period, in data points, for the indicator to be ready and fully initialized.
int
window
A rolling window keeping a history of the indicator values of a given period
A rolling window keeping a history of the indicator values of a given period
RollingWindow[IndicatorDataPoint]
Visualization
The following image shows plot values of selected properties of IchimokuKinkoHyo
using the plotly library.
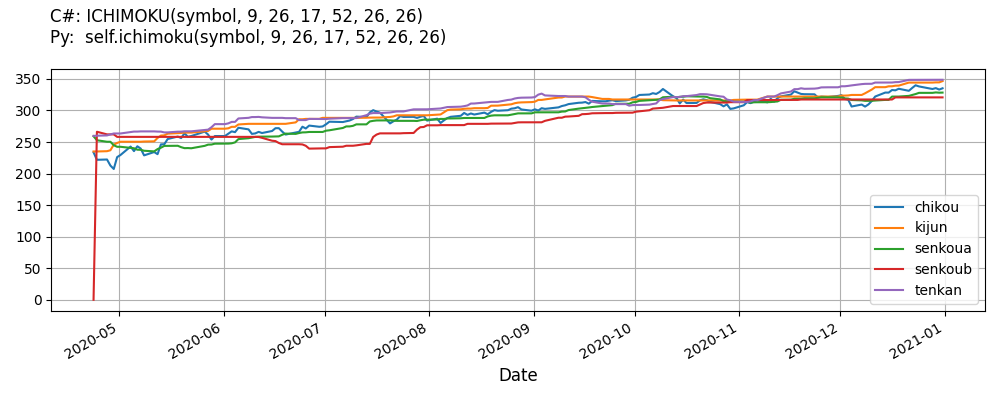
Indicator History
To get the historical data of the IchimokuKinkoHyo
indicator, call the self.indicator_history
method.
This method resets your indicator, makes a history request, and updates the indicator with the historical data.
Just like with regular history requests, the indicator_history
method supports time periods based on a trailing number of bars, a trailing period of time, or a defined period of time.
If you don't provide a resolution
argument, it defaults to match the resolution of the security subscription.
class IchimokuKinkoHyoAlgorithm(QCAlgorithm): def initialize(self) -> None: self._symbol = self.add_equity("SPY", Resolution.DAILY).symbol ichimoku = self.ichimoku(self._symbol, 9, 26, 17, 52, 26, 26) count_indicator_history = self.indicator_history(ichimoku, self._symbol, 100, Resolution.MINUTE) timedelta_indicator_history = self.indicator_history(ichimoku, self._symbol, timedelta(days=10), Resolution.MINUTE) time_period_indicator_history = self.indicator_history(ichimoku, self._symbol, datetime(2024, 7, 1), datetime(2024, 7, 5), Resolution.MINUTE)
To make the indicator_history
method update the indicator with an alternative price field instead of the close (or mid-price) of each bar, pass a selector
argument.
indicator_history = self.indicator_history(ichimoku, 100, Resolution.MINUTE, lambda bar: bar.high) indicator_history_df = indicator_history.data_frame
To access the properties of the indicator history, index the DataFrame with the property name.
tenkan = indicator_history_df["tenkan"] kijun = indicator_history_df["kijun"] senkou_a = indicator_history_df["senkou_a"] senkou_b = indicator_history_df["senkou_b"] chikou = indicator_history_df["chikou"] tenkan_maximum = indicator_history_df["tenkan_maximum"] tenkan_minimum = indicator_history_df["tenkan_minimum"] kijun_maximum = indicator_history_df["kijun_maximum"] kijun_minimum = indicator_history_df["kijun_minimum"] senkou_b_maximum = indicator_history_df["senkou_b_maximum"] senkou_b_minimum = indicator_history_df["senkou_b_minimum"] delayed_tenkan_senkou_a = indicator_history_df["delayed_tenkan_senkou_a"] delayed_kijun_senkou_a = indicator_history_df["delayed_kijun_senkou_a"] delayed_maximum_senkou_b = indicator_history_df["delayed_maximum_senkou_b"] delayed_minimum_senkou_b = indicator_history_df["delayed_minimum_senkou_b"] # Alternative way # tenkan = indicator_history_df.tenkan # kijun = indicator_history_df.kijun # senkou_a = indicator_history_df.senkou_a # senkou_b = indicator_history_df.senkou_b # chikou = indicator_history_df.chikou # tenkan_maximum = indicator_history_df.tenkan_maximum # tenkan_minimum = indicator_history_df.tenkan_minimum # kijun_maximum = indicator_history_df.kijun_maximum # kijun_minimum = indicator_history_df.kijun_minimum # senkou_b_maximum = indicator_history_df.senkou_b_maximum # senkou_b_minimum = indicator_history_df.senkou_b_minimum # delayed_tenkan_senkou_a = indicator_history_df.delayed_tenkan_senkou_a # delayed_kijun_senkou_a = indicator_history_df.delayed_kijun_senkou_a # delayed_maximum_senkou_b = indicator_history_df.delayed_maximum_senkou_b # delayed_minimum_senkou_b = indicator_history_df.delayed_minimum_senkou_b