India Equity
Handling Data
Introduction
LEAN passes the data you request to the OnData
on_data
method so you can make trading decisions. The default OnData
on_data
method accepts a Slice
object, but you can define additional OnData
on_data
methods that accept different data types. For example, if you define an OnData
on_data
method that accepts a TradeBar
argument, it only receives TradeBar
objects. The Slice
object that the OnData
on_data
method receives groups all the data together at a single moment in time. To access the Slice
outside of the OnData
on_data
method, use the CurrentSlice
current_slice
property of your algorithm.
All the data formats use DataDictionary
objects to group data by Symbol
and provide easy access to information. The plural of the type denotes the collection of objects. For instance, the TradeBars
DataDictionary
is made up of TradeBar
objects. To access individual data points in the dictionary, you can index the dictionary with the security ticker or Symbol
symbol
, but we recommend you use the Symbol
symbol
.
To view the resolutions that are available for India Equity data, see Resolutions.
Trades
TradeBar
objects are price bars that consolidate individual trades from the exchanges. They contain the open, high, low, close, and volume of trading activity over a period of time.
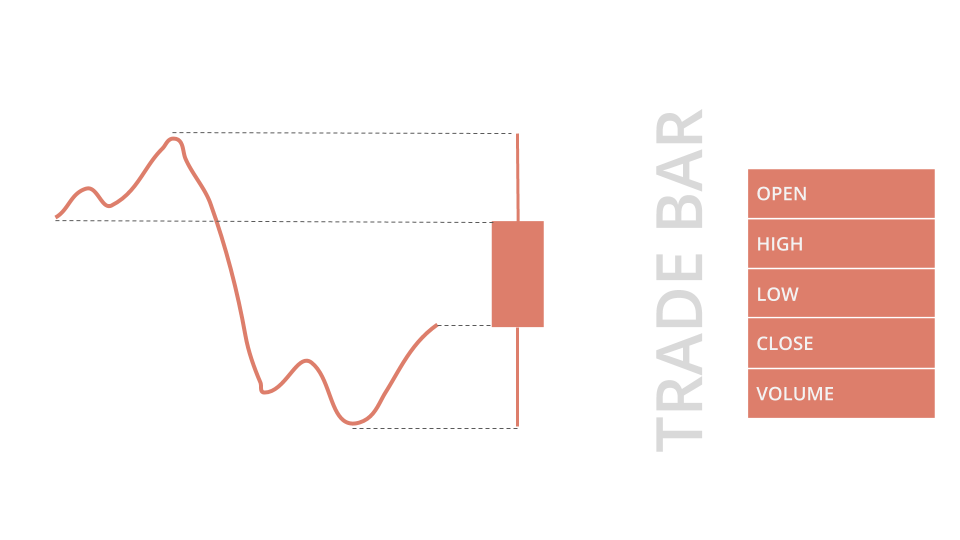
To get the TradeBar
objects in the Slice
, index the Slice
or index the Bars
bars
property of the Slice
with the security Symbol
symbol
. If the security doesn't actively trade or you are in the same time step as when you added the security subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your security before you index the Slice
with the security Symbol
symbol
.
public override void OnData(Slice slice) { // Check if the symbol is contained in TradeBars object if (slice.Bars.ContainsKey(_symbol)) { // Obtain the mapped TradeBar of the symbol var tradeBar = slice.Bars[_symbol]; } }
def on_data(self, slice: Slice) -> None: # Obtain the mapped TradeBar of the symbol if any trade_bar = slice.bars.get(self._symbol) # None if not found
You can also iterate through the TradeBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the TradeBar
objects.
public override void OnData(Slice slice) { // Iterate all received Symbol-TradeBar key-value pairs foreach (var kvp in slice.Bars) { var symbol = kvp.Key; var tradeBar = kvp.Value; var closePrice = tradeBar.Close; } }
def on_data(self, slice: Slice) -> None: # Iterate all received Symbol-TradeBar key-value pairs for symbol, trade_bar in slice.bars.items(): close_price = trade_bar.close
TradeBar
objects have the following properties: