Charting
Plotly
Get Historical Data
Get some historical market data to produce the plots. For example, to get data for a bank sector ETF and some banking companies over 2021, run:
qb = QuantBook() tickers = ["XLF", # Financial Select Sector SPDR Fund "COF", # Capital One Financial Corporation "GS", # Goldman Sachs Group, Inc. "JPM", # J P Morgan Chase & Co "WFC"] # Wells Fargo & Company symbols = [qb.add_equity(ticker, Resolution.DAILY).symbol for ticker in tickers] history = qb.history(symbols, datetime(2021, 1, 1), datetime(2022, 1, 1))
Create Candlestick Chart
You must import the plotting libraries and get some historical data to create candlestick charts.
In this example, you create a candlestick chart that shows the open, high, low, and close prices of one of the banking securities. Follow these steps to create the candlestick chart:
- Select a
Symbol
. - Slice the
history
DataFrame
with thesymbol
. - Call the
Candlestick
constructor with the time and open, high, low, and close priceSeries
. - Call the
Layout
constructor with a title and axes labels. - Call the
Figure
constructor with thecandlestick
andlayout
. - Call the
show
method.
symbol = symbols[0]
data = history.loc[symbol]
candlestick = go.Candlestick(x=data.index, open=data['open'], high=data['high'], low=data['low'], close=data['close'])
layout = go.Layout(title=go.layout.Title(text=f'{symbol.value} OHLC'), xaxis_title='Date', yaxis_title='Price', xaxis_rangeslider_visible=False)
fig = go.Figure(data=[candlestick], layout=layout)
fig.show()
The Jupyter Notebook displays the candlestick chart.
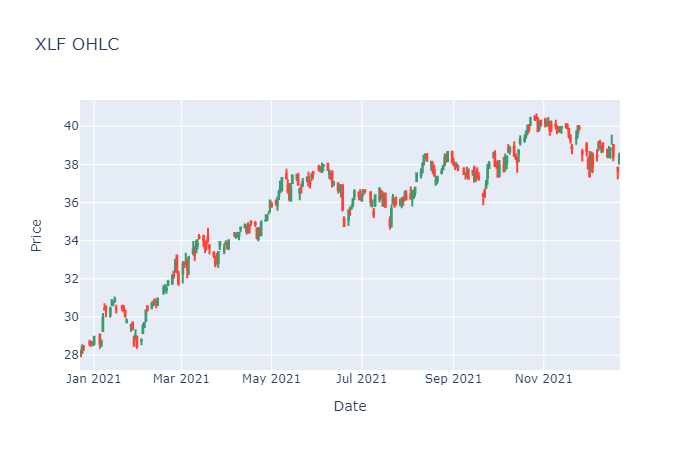
Create Line Chart
You must import the plotting libraries and get some historical data to create line charts.
In this example, you create a line chart that shows the closing price for one of the banking securities. Follow these steps to create the line chart:
- Select a
Symbol
. - Slice the
history
DataFrame with thesymbol
and then select the close column. - Call the
DataFrame
constructor with thedata Series
and then call thereset_index
method. - Call the
line
method withdata
, the column names of the x- and y-axis indata
, and the plot title. - Call the
show
method.
symbol = symbols[0]
data = history.loc[symbol]['close']
data = pd.DataFrame(data).reset_index()
fig = px.line(data, x='time', y='close', title=f'{symbol} Close price')
fig.show()
The Jupyter Notebook displays the line chart.
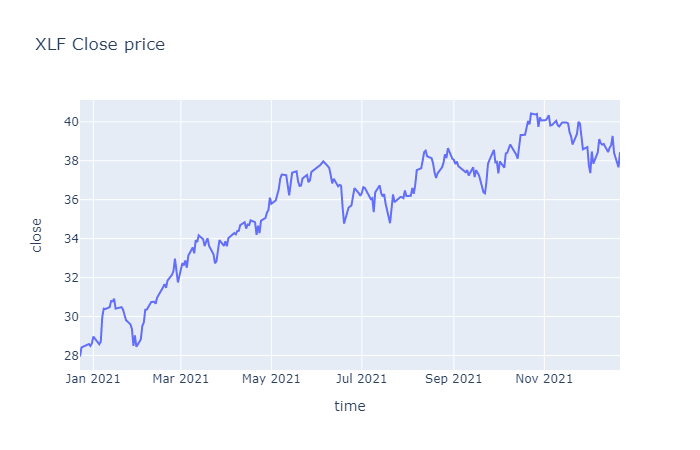
Create Scatter Plot
You must import the plotting libraries and get some historical data to create scatter plots.
In this example, you create a scatter plot that shows the relationship between the daily returns of two banking securities. Follow these steps to create the scatter plot:
- Select 2
Symbol
s. - Slice the
history
DataFrame with eachSymbol
and then select the close column. - Call the
pct_change
anddropna
methods on eachSeries
. - Call the
scatter
method with the 2 returnSeries
, the trendline option, and axes labels. - Call the
update_layout
method with a title. - Call the
show
method.
For example, to select the Symbol
s of the first 2 bank stocks, run:
symbol1 = symbols[1] symbol2 = symbols[2]
close_price1 = history.loc[symbol1]['close'] close_price2 = history.loc[symbol2]['close']
daily_return1 = close_price1.pct_change().dropna() daily_return2 = close_price2.pct_change().dropna()
fig = px.scatter(x=daily_return1, y=daily_return2, trendline='ols', labels={'x': symbol1.value, 'y': symbol2.value})
fig.update_layout(title=f'{symbol1.value} vs {symbol2.value} Daily % Returns');
fig.show()
The Jupyter Notebook displays the scatter plot.
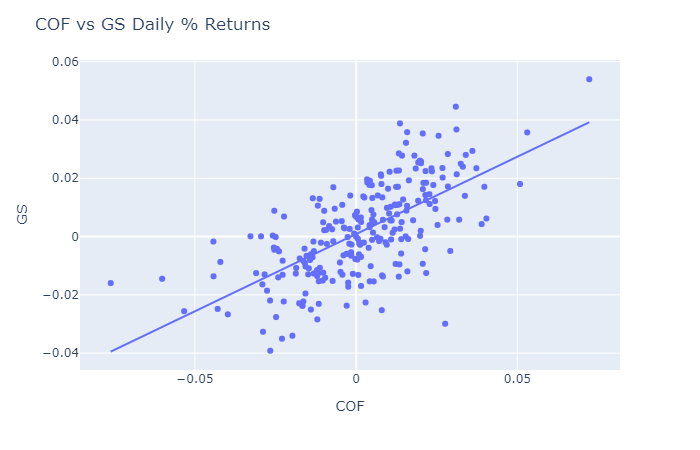
Create Histogram
You must import the plotting libraries and get some historical data to create histograms.
In this example, you create a histogram that shows the distribution of the daily percent returns of the bank sector ETF. Follow these steps to create the histogram:
- Select the
Symbol
. - Slice the
history
DataFrame with thesymbol
and then select the close column. - Call the
pct_change
method and then call thedropna
method. - Call the
DataFrame
constructor with thedata Series
and then call thereset_index
method. - Call the
histogram
method with thedaily_returns
DataFrame, the x-axis label, a title, and the number of bins. - Call the
show
method.
symbol = symbols[0]
data = history.loc[symbol]['close']
daily_returns = data.pct_change().dropna()
daily_returns = pd.DataFrame(daily_returns).reset_index()
fig = px.histogram(daily_returns, x='close', title=f'{symbol} Daily Return of Close Price Distribution', nbins=20)
fig.show()
The Jupyter Notebook displays the histogram.
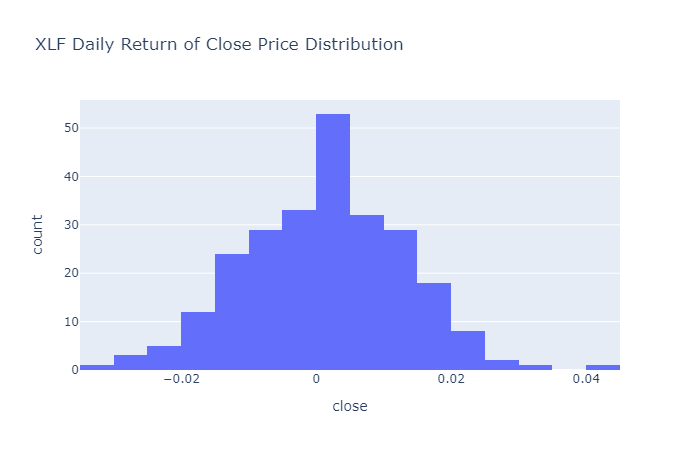
Create Bar Chart
You must import the plotting libraries and get some historical data to create bar charts.
In this example, you create a bar chart that shows the average daily percent return of the banking securities. Follow these steps to create the bar chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method and then multiply by 100. - Call the
mean
method. - Call the
DataFrame
constructor with theavg_daily_returns
Series
and then call thereset_index
method. - Call the
bar
method with theavg_daily_returns
and the axes column names. - Call the
update_layout
method with a title. - Call the
show
method.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change() * 100
avg_daily_returns = daily_returns.mean()
avg_daily_returns = pd.DataFrame(avg_daily_returns, columns=["avg_daily_ret"]).reset_index()
fig = px.bar(avg_daily_returns, x='symbol', y='avg_daily_ret')
fig.update_layout(title='Banking Stocks Average Daily % Returns');
fig.show()
The Jupyter Notebook displays the bar plot.
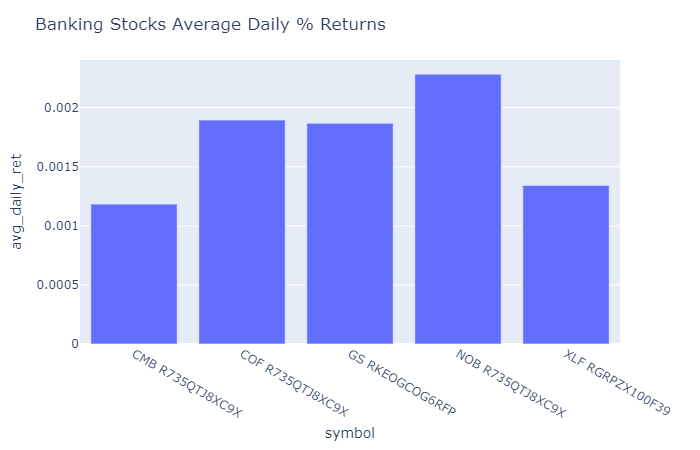
Create Heat Map
You must import the plotting libraries and get some historical data to create heat maps.
In this example, you create a heat map that shows the correlation between the daily returns of the banking securities. Follow these steps to create the heat map:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call the
corr
method. - Call the
imshow
method with thecorr_matrix
and the axes labels. - Call the
update_layout
method with a title. - Call the
show
method.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
corr_matrix = daily_returns.corr()
fig = px.imshow(corr_matrix, x=tickers, y=tickers)
fig.update_layout(title='Banking Stocks and bank sector ETF Correlation Heat Map');
fig.show()
The Jupyter Notebook displays the heat map.
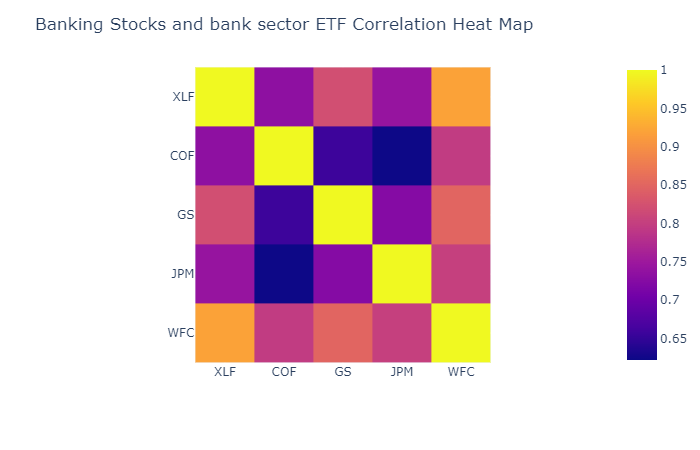
Create Pie Chart
You must import the plotting libraries and get some historical data to create pie charts.
In this example, you create a pie chart that shows the weights of the banking securities in a portfolio if you allocate to them based on their inverse volatility. Follow these steps to create the pie chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call the
var
method and then take the inverse. - Call the
DataFrame
constructor with theinverse_variance Series
and then call thereset_index
method. - Call the
pie
method with theinverse_variance DataFrame
, the column name of the values, and the column name of the names. - Call the
update_layout
method with a title. - Call the
show
method.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
inverse_variance = 1 / daily_returns.var()
inverse_variance = pd.DataFrame(inverse_variance, columns=["inverse variance"]).reset_index()
fig = px.pie(inverse_variance, values='inverse variance', names='symbol')
fig.update_layout(title='Asset Allocation of bank stocks and bank sector ETF');
fig.show()
The Jupyter Notebook displays the pie chart.
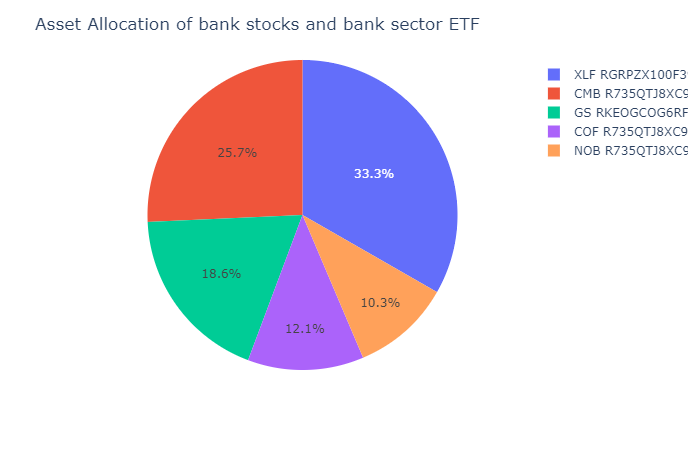
Create 3D Chart
You must import the plotting libraries and get some historical data to create 3D charts.
In this example, you create a 3D chart that shows the price of an asset on each dimension. Follow these steps to create the 3D chart:
- Select the asset to plot on each dimension.
- Call the
Scatter3d
constructor with the data for the x, y, and z axes. - Call the
Layout
constructor with the axes titles and chart dimensions. - Call the
Figure
constructor with thescatter
andlayout
variables. - Call the
show
method.
x, y, z = symbols[:3]
scatter = go.Scatter3d( x=history.loc[x].close, y=history.loc[y].close, z=history.loc[z].close, mode='markers', marker=dict( size=2, opacity=0.8 ) )
layout = go.Layout( scene=dict( xaxis_title=f'{x.value} Price', yaxis_title=f'{y.value} Price', zaxis_title=f'{z.value} Price' ), width=700, height=700 )
fig = go.Figure(scatter, layout)
fig.show()
The Jupyter Notebook displays the 3D chart.
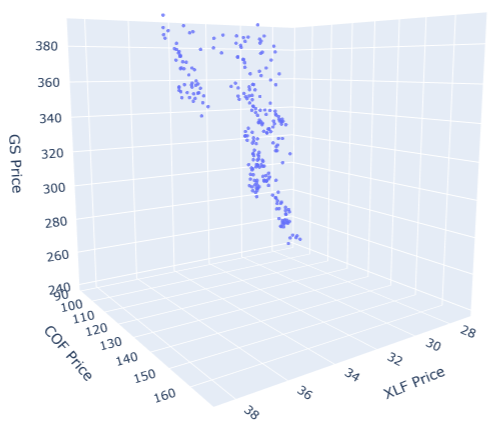