Indicators
Bar Indicators
Create Subscriptions
You need to subscribe to some market data in order to calculate indicator values.
qb = QuantBook() symbol = qb.add_equity("SPY").symbol
Create Indicator Timeseries
You need to subscribe to some market data and create an indicator in order to calculate a timeseries of indicator values. In this example, use a 20-period AverageTrueRange
indicator.
atr = AverageTrueRange(20)
You can create the indicator timeseries with the Indicator
helper method or you can manually create the timeseries.
Indicator Helper Method
To create an indicator timeseries with the helper method, call the Indicator
method.
# Create a dataframe with a date index, and columns are indicator values. atr_dataframe = qb.indicator(atr, symbol, 50, Resolution.DAILY)
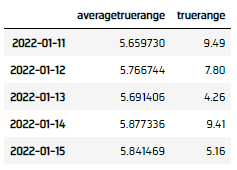
Manually Create the Indicator Timeseries
Follow these steps to manually create the indicator timeseries:
- Get some historical data.
- Set the indicator
window.size
for each attribute of the indicator to hold their values. - Iterate through the historical market data and update the indicator.
- Populate a
DataFrame
with the data in theIndicator
object.
# Request historical trading data with the daily resolution. history = qb.history[TradeBar](symbol, 70, Resolution.DAILY)
# Set the window.size to the desired timeseries length atr.window.size = 50 atr.true_range.window.size = 50
for bar in history: atr.update(bar)
atr_dataframe = pd.DataFrame({ "current": pd.Series({x.end_time: x.value for x in atr}), "truerange": pd.Series({x.end_time: x.value for x in atr.true_range}) }).sort_index()
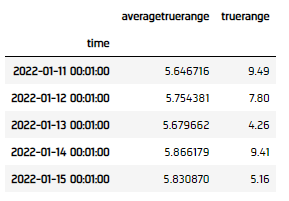
Plot Indicators
You need to create an indicator timeseries to plot the indicator values.
Follow these steps to plot the indicator values:
- Call the
plot
method. - Show the plots.
atr_indicator.plot(title="SPY ATR(20)", figsize=(15, 10))
plt.show()
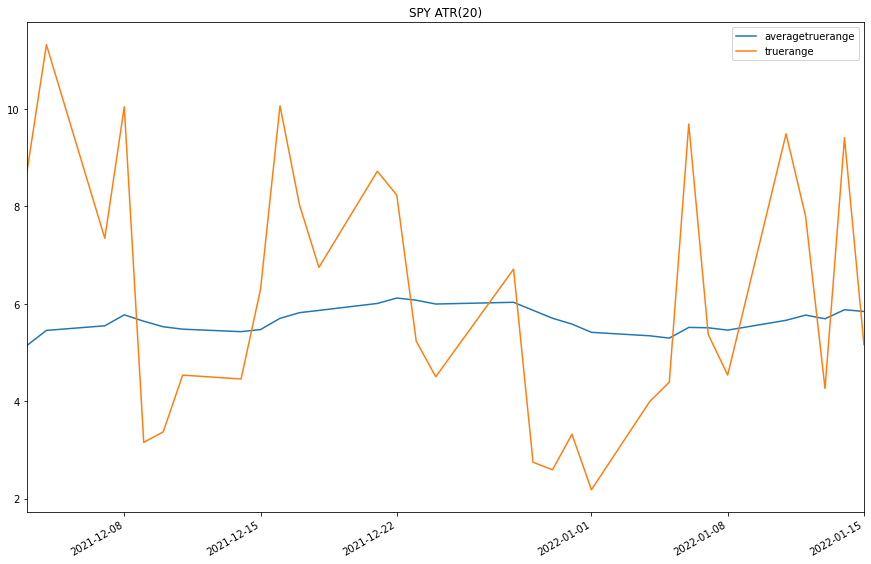
Examples
The following examples demonstrate some common practices for researching with bar indicators.
Example 1: Quick Backtest On William Percent Ratio
The following example demonstrates a quick backtest to testify the effectiveness of a William Percent Ratio mean-reversal under the research enviornment.
# Instantiate the QuantBook instance for researching. qb = QuantBook() # Request SPY data to work with the indicator. symbol = qb.add_equity("SPY").symbol # Get the historical data for trading. history = qb.history(symbol, 252, Resolution.DAILY).close.unstack(0).iloc[:, 0] # Create the William %R indicator with parameters to be studied. indicator = WilliamsPercentR(20) # Get the indicator history of the indicator. indicator_dataframe = qb.indicator(indicator, symbol, 252, Resolution.DAILY) # Create a order record and return column. # Buy if the asset is underprice (below -80), sell if overpriced (above -20) indicator_dataframe["position"] = indicator_dataframe.apply(lambda x: 1 if x.current < -80 else -1 if x.current > -20 else 0, axis=1) # Get the 1-day forward return. indicator_dataframe["return"] = history.pct_change().shift(-1).fillna(0) indicator_dataframe["return"] = indicator_dataframe["position"] * indicator_dataframe["return"] # Obtain the cumulative return curve as a mini-backtest. equity_curve = (indicator_dataframe["return"] + 1).cumprod() equity_curve.plot(title="Equity Curve", ylabel="Equity", xlabel="time")