Charting
Bokeh
Import Libraries
Follow these steps to import the libraries that you need:
- Import the
bokeh
library. - Call the
output_notebook
method. - Import the
numpy
library.
from bokeh.plotting import figure, show from bokeh.models import BasicTicker, ColorBar, ColumnDataSource, LinearColorMapper from bokeh.palettes import Category20c from bokeh.transform import cumsum, transform from bokeh.io import output_notebook
output_notebook()
import numpy as np
Get Historical Data
Get some historical market data to produce the plots. For example, to get data for a bank sector ETF and some banking companies over 2021, run:
qb = QuantBook() tickers = ["XLF", # Financial Select Sector SPDR Fund "COF", # Capital One Financial Corporation "GS", # Goldman Sachs Group, Inc. "JPM", # J P Morgan Chase & Co "WFC"] # Wells Fargo & Company symbols = [qb.add_equity(ticker, Resolution.DAILY).symbol for ticker in tickers] history = qb.history(symbols, datetime(2021, 1, 1), datetime(2022, 1, 1))
Create Candlestick Chart
You must import the plotting libraries and get some historical data to create candlestick charts.
In this example, you create a candlestick chart that shows the open, high, low, and close prices of one of the banking securities. Follow these steps to create the candlestick chart:
- Select a
Symbol
. - Slice the
history
DataFrame
with thesymbol
. - Divide the
data
into days with positive returns and days with negative returns. - Call the
figure
function with a title, axis labels and x-axis type. - Call the
segment
method with thedata
timestamps, high prices, low prices, and a color. - Call the
vbar
method for the up and down days with thedata
timestamps, open prices, close prices, and a color. - Call the
show
function.
symbol = symbols[0]
data = history.loc[symbol]
up_days = data[data['close'] > data['open']] down_days = data[data['open'] > data['close']]
plot = figure(title=f"{symbol} OHLC", x_axis_label='Date', y_axis_label='Price', x_axis_type='datetime')
plot.segment(data.index, data['high'], data.index, data['low'], color="black")
This method call plots the candlestick wicks.
width = 12*60*60*1000 plot.vbar(up_days.index, width, up_days['open'], up_days['close'],
fill_color="green", line_color="green") plot.vbar(down_days.index, width, down_days['open'], down_days['close'],
fill_color="red", line_color="red")
This method call plots the candlestick bodies.
show(plot)
The Jupyter Notebook displays the candlestick chart.
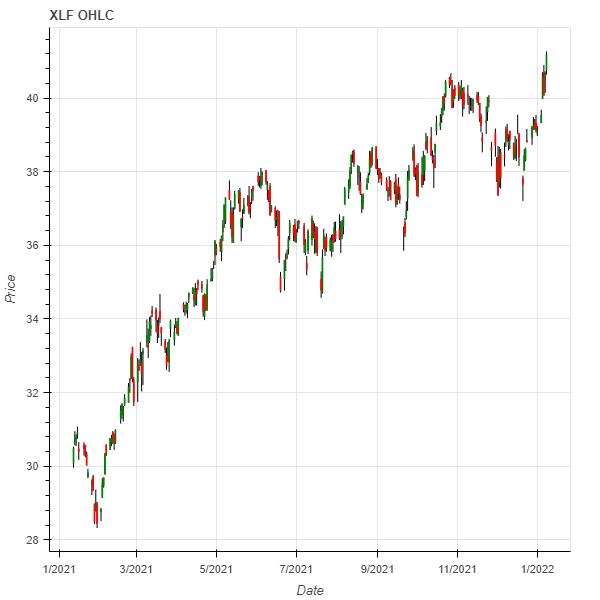
Create Line Plot
You must import the plotting libraries and get some historical data to create line charts.
In this example, you create a line chart that shows the closing price for one of the banking securities. Follow these steps to create the line chart:
- Select a
Symbol
. - Slice the
history
DataFrame with thesymbol
and then select the close column. - Call the
figure
function with title, axis labels and x-axis type.. - Call the
line
method with the timestamps,close_prices
, and some design settings. - Call the
show
function.
symbol = symbols[0]
close_prices = history.loc[symbol]['close']
plot = figure(title=f"{symbol} Close Price", x_axis_label='Date', y_axis_label='Price', x_axis_type='datetime')
plot.line(close_prices.index, close_prices, legend_label=symbol.value, color="blue", line_width=2)
show(plot)
The Jupyter Notebook displays the line plot.
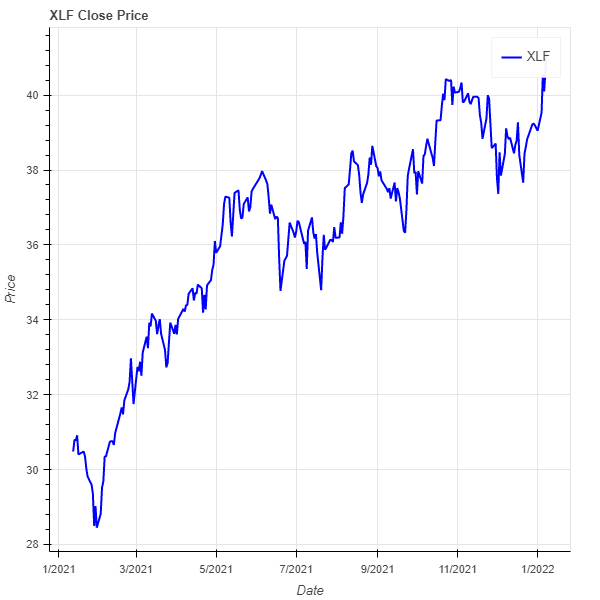
Create Scatter Plot
You must import the plotting libraries and get some historical data to create scatter plots.
In this example, you create a scatter plot that shows the relationship between the daily returns of two banking securities. Follow these steps to create the scatter plot:
- Select 2
Symbol
s. - Slice the
history
DataFrame with eachSymbol
and then select the close column. - Call the
pct_change
anddropna
methods on eachSeries
. - Call the
polyfit
method with thedaily_returns1
,daily_returns2
, and a degree. - Call the
linspace
method with the minimum and maximum values on the x-axis. - Calculate the y-axis coordinates of the regression line.
- Call the
figure
function with a title and axis labels. - Call the
line
method with x- and y-axis values, a color, and a line width. - Call the
dot
method with thedaily_returns1
,daily_returns2
, and some design settings. - Call the
show
function.
For example, to select the Symbol
s of the first 2 bank stocks, run:
symbol1 = symbols[1] symbol2 = symbols[2]
close_price1 = history.loc[symbol1]['close'] close_price2 = history.loc[symbol2]['close']
daily_return1 = close_price1.pct_change().dropna() daily_return2 = close_price2.pct_change().dropna()
m, b = np.polyfit(daily_returns1, daily_returns2, deg=1)
This method call returns the slope and intercept of the ordinary least squares regression line.
x = np.linspace(daily_returns1.min(), daily_returns1.max())
y = m*x + b
plot = figure(title=f"{symbol1} vs {symbol2} Daily Return", x_axis_label=symbol1.value, y_axis_label=symbol2.value)
plot.line(x, y, color="red", line_width=2)
This method call plots the regression line.
plot.dot(daily_returns1, daily_returns2, size=20, color="navy", alpha=0.5)
This method call plots the scatter plot dots.
show(plot)
The Jupyter Notebook displays the scatter plot.
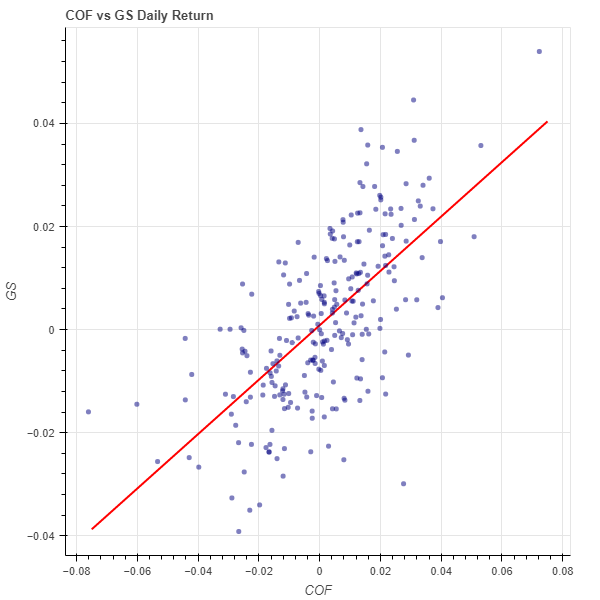
Create Histogram
You must import the plotting libraries and get some historical data to create histograms.
In this example, you create a histogram that shows the distribution of the daily percent returns of the bank sector ETF. In addition to the bins in the histogram, you overlay a normal distribution curve for comparison. Follow these steps to create the histogram:
- Select the
Symbol
. - Slice the
history
DataFrame with thesymbol
and then select the close column. - Call the
pct_change
method and then call thedropna
method. - Call the
histogram
method with thedaily_returns
, the density argument enabled, and a number of bins. hist
: The value of the probability density function at each bin, normalized such that the integral over the range is 1.edges
: The x-axis value of the edges of each bin.- Call the
figure
method with a title and axis labels. - Call the
quad
method with the coordinates of the bins and some design settings. - Call the
mean
andstd
methods. - Call the
linspace
method with the lower limit, upper limit, and number data points for the x-axis of the normal distribution curve. - Calculate the y-axis values of the normal distribution curve.
- Call the
line
method with the data and style of the normal distribution PDF curve. - Call
show
to show the plot.
symbol = symbols[0]
close_prices = history.loc[symbol]['close']
daily_returns = close_prices.pct_change().dropna()
hist, edges = np.histogram(daily_returns, density=True, bins=20)
This method call returns the following objects:
plot = figure(title=f"{symbol} Daily Return Distribution", x_axis_label='Return', y_axis_label='Frequency')
plot.quad(top=hist, bottom=0, left=edges[:-1], right=edges[1:], fill_color="navy", line_color="white", alpha=0.5)
This method call plots the histogram bins.
mean = daily_returns.mean() std = daily_returns.std()
x = np.linspace(-3*std, 3*std, 1000)
pdf = 1/(std * np.sqrt(2*np.pi)) * np.exp(-(x-mean)**2 / (2*std**2))
plot.line(x, pdf, color="red", line_width=4, alpha=0.7, legend_label="Normal Distribution PDF")
This method call plots the normal distribution PDF curve.
show(plot)
The Jupyter Notebook displays the histogram.
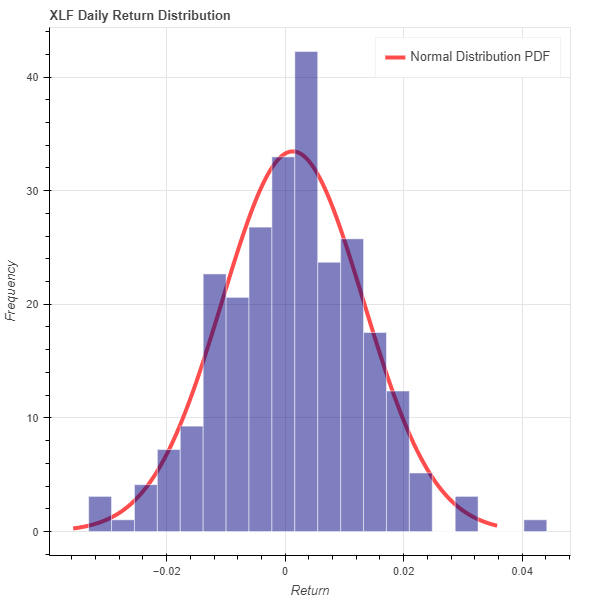
Create Bar Chart
You must import the plotting libraries and get some historical data to create bar charts.
In this example, you create a bar chart that shows the average daily percent return of the banking securities. Follow these steps to create the bar chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method and then multiply by 100. - Call the
mean
method. - Call the
DataFrame
constructor with thedata Series
and then call thereset_index
method. - Call the
figure
function with a title, x-axis values, and axis labels. - Call the
vbar
method with theavg_daily_returns
, x- and y-axis column names, and a bar width. - Rotate the x-axis label and then call the
show
function.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change() * 100
avg_daily_returns = daily_returns.mean()
avg_daily_returns = pd.DataFrame(avg_daily_returns, columns=['avg_return']).reset_index()
plot = figure(title='Banking Stocks Average Daily % Returns', x_range=avg_daily_returns['symbol'], x_axis_label='%', y_axis_label='Stocks')
plot.vbar(source=avg_daily_returns, x='symbol', top='avg_return', width=0.8)
plot.xaxis.major_label_orientation = 0.6 show(plot)
The Jupyter Notebook displays the bar chart.
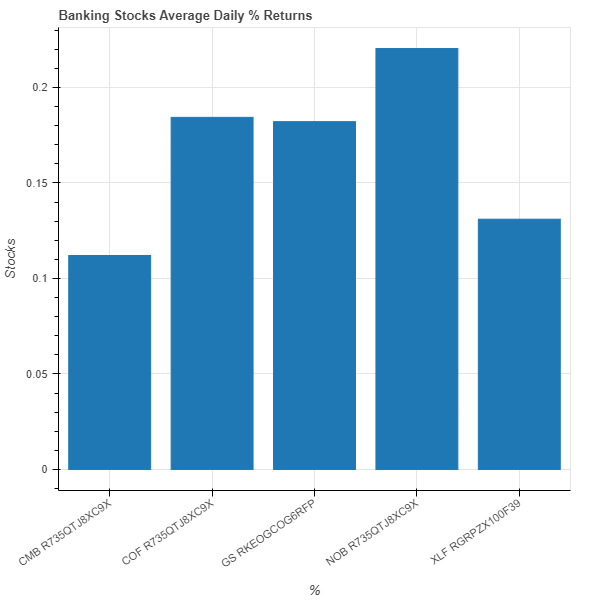
Create Heat Map
You must import the plotting libraries and get some historical data to create heat maps.
In this example, you create a heat map that shows the correlation between the daily returns of the banking securities. Follow these steps to create the heat map:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call the
corr
method. - Set the index and columns of the
corr_matrix
to the ticker of each security and then set the name of the column and row indices. - Call the
stack
,rename
, andreset_index
methods. - Call the
figure
function with a title, axis ticks, and some design settings. - Select a color palette and then call the
LinearColorMapper
constructor with the color pallet, the minimum correlation, and the maximum correlation. - Call the
rect
method with the correlation plot data and design setting. - Call the
ColorBar
constructor with themapper
, a location, and aBaseTicker
. - Call the
add_layout
method with thecolor_bar
and a location. - Call the
show
function.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
corr_matrix = daily_returns.corr()
corr_matrix.index = corr_matrix.columns = [symbol.value for symbol in symbols] corr_matrix.index.name = 'symbol' corr_matrix.columns.name = "stocks"
corr_matrix = corr_matrix.stack().rename("value").reset_index()
plot = figure(title=f"Banking Stocks and Bank Sector ETF Correlation Heat Map", x_range=list(corr_matrix.symbol.drop_duplicates()), y_range=list(corr_matrix.stocks.drop_duplicates()), toolbar_location=None, tools="", x_axis_location="above")
colors = Category20c[len(corr_matrix.columns)] mapper = LinearColorMapper(palette=colors, low=corr_matrix.value.min(), high=corr_matrix.value.max())
plot.rect(source=ColumnDataSource(corr_matrix), x="stocks", y="symbol", width=1, height=1, line_color=None, fill_color=transform('value', mapper))
color_bar = ColorBar(color_mapper=mapper, location=(0, 0), ticker=BasicTicker(desired_num_ticks=len(colors)))
This snippet creates a color bar to represent the correlation coefficients of the heat map cells.
plot.add_layout(color_bar, 'right')
This method call plots the color bar to the right of the heat map.
show(plot)
The Jupyter Notebook displays the heat map.
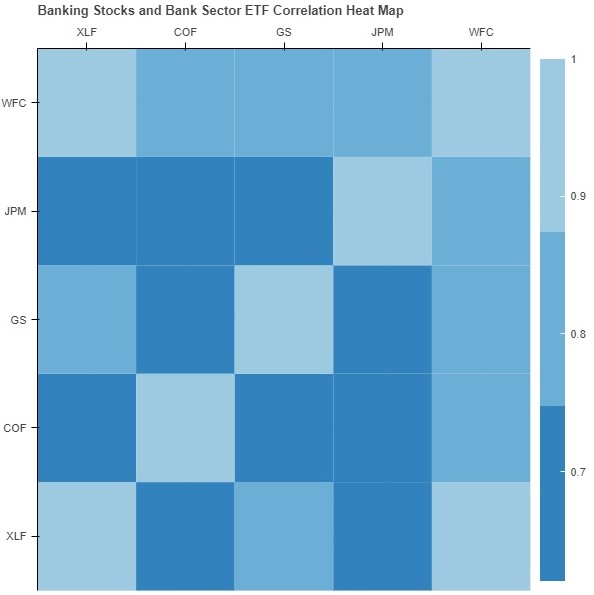
Create Pie Chart
You must import the plotting libraries and get some historical data to create pie charts.
In this example, you create a pie chart that shows the weights of the banking securities in a portfolio if you allocate to them based on their inverse volatility. Follow these steps to create the pie chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call the
var
method, take the inverse, and then normalize the result. - Call the
DataFrame
constructor with theinverse_variance Series
and then call thereset_index
method. - Add a color column to the
inverse_variance DataFrame
. - Call the
figure
function with a title. - Call the
wedge
method with design settings and theinverse_variance DataFrame
. - Call the
show
function.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
inverse_variance = 1 / daily_returns.var() inverse_variance /= np.sum(inverse_variance) # Normalization inverse_variance *= np.pi*2 # For a full circle circumference in radian
inverse_variance = pd.DataFrame(inverse_variance, columns=["inverse variance"]).reset_index()
inverse_variance['color'] = Category20c[len(inverse_variance.index)]
plot = figure(title=f"Banking Stocks and Bank Sector ETF Allocation")
plot.wedge(x=0, y=1, radius=0.6, start_angle=cumsum('inverse variance', include_zero=True), end_angle=cumsum('inverse variance'), line_color="white", fill_color='color', legend_field='symbol', source=inverse_variance)
show(plot)
The Jupyter Notebook displays the pie chart.
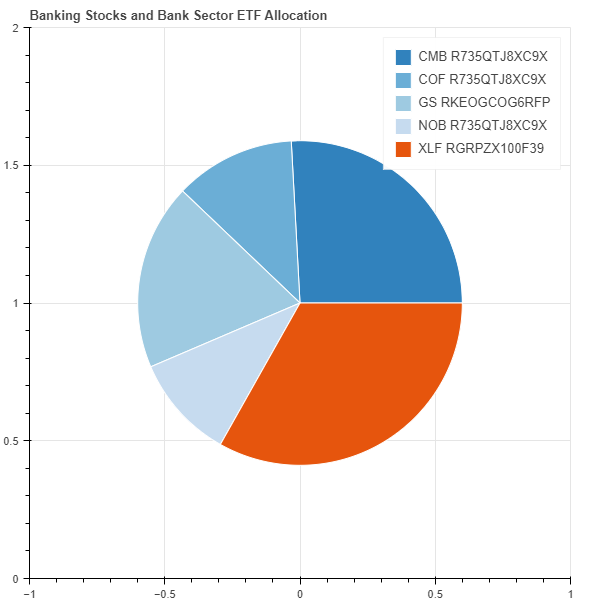