Equity Options
Universes
Create Subscriptions
Follow these steps to subscribe to an Equity Option universe:
- Load the assembly files and data types in their own cell.
- Import the data types.
- Create a
QuantBook
. - Subscribe to the underlying Equity with raw data normalization and save a reference to the Equity
Symbol
. - Call the
AddOption
add_option
method with the underlying EquitySymbol
.
#load "../Initialize.csx"
#load "../QuantConnect.csx" #r "../Microsoft.Data.Analysis.dll" using QuantConnect; using QuantConnect.Data; using QuantConnect.Algorithm; using QuantConnect.Research; using QuantConnect.Indicators; using QuantConnect.Securities.Option; using QuantConnect.Data.UniverseSelection; using Microsoft.Data.Analysis;
var qb = new QuantBook();
qb = QuantBook()
var equitySymbol = qb.AddEquity("SPY", dataNormalizationMode: DataNormalizationMode.Raw).Symbol;
equity_symbol = qb.add_equity("SPY", data_normalization_mode=DataNormalizationMode.RAW).symbol
To view the supported underlying assets in the US Equity Options dataset, see the Data Explorer.
var option = qb.AddOption(equitySymbol);
option = qb.add_option(equity_symbol)
Price History
The contract filter determines which Equity Option contracts are in your universe each trading day. The default filter selects the contracts with the following characteristics:
- Standard type (exclude weeklys)
- Within 1 strike price of the underlying asset price
- Expire within 35 days
To change the filter, call the SetFilter
set_filter
method.
// Set the contract filter to select contracts that have the strike price // within 1 strike level and expire within 90 days. option.SetFilter(-1, 1, 0, 90);
# Set the contract filter to select contracts that have the strike price # within 1 strike level and expire within 90 days. option.set_filter(-1, 1, 0, 90)
To get the prices and volumes for all of the Equity Option contracts that pass your filter during a specific period of time, call the OptionHistory
option_history
method with the underlying Equity Symbol
object, a start DateTime
datetime
, and an end DateTime
datetime
.
option_history = qb.option_history( equity_symbol, datetime(2024, 1, 1), datetime(2024, 1, 5), Resolution.MINUTE, fill_forward=False, extended_market_hours=False )
var optionHistory = qb.OptionHistory( equitySymbol, new DateTime(2024, 1, 1), new DateTime(2024, 1, 5), Resolution.Minute, fillForward: false, extendedMarketHours: false );
To convert the OptionHistory
object to a DataFrame
that contains the trade and quote information of each contract and the underlying, use the data_frame
property.
option_history.data_frame
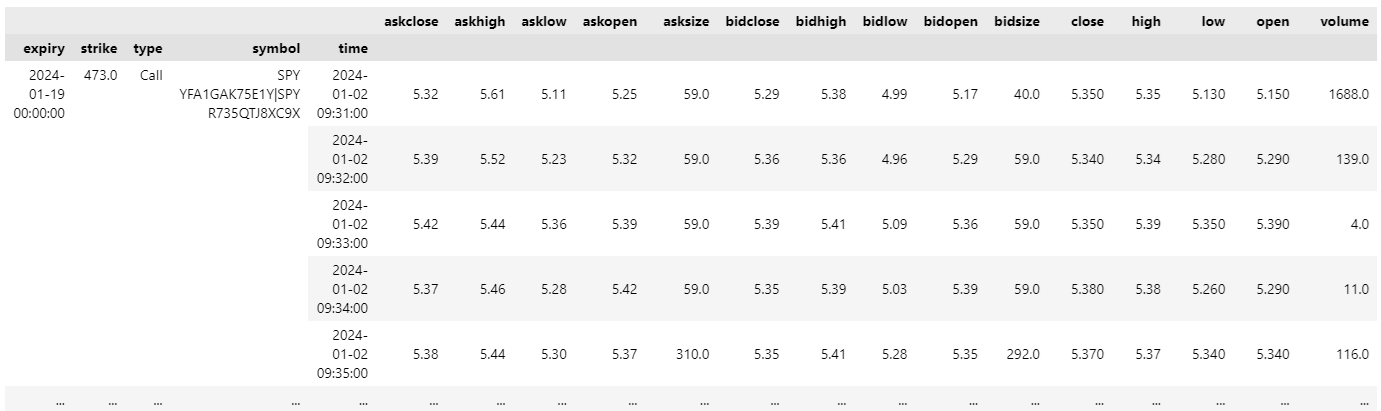
To get the expiration dates of all the contracts in an OptionHistory
object, call the GetExpiryDates
get_expiry_dates
method.
option_history.get_expiry_dates()
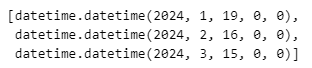
To get the strike prices of all the contracts in an OptionHistory
object, call the GetStrikes
get_strikes
method.
option_history.get_strikes()

Daily Price and Greeks History
To get daily data on all the tradable contracts for a given date, call the History<OptionUniverse>
history
method with the canoncial Option Symbol, a start date, and an end date. This method returns the entire Option chain for each trading day, not the subset of contracts that pass your universe filter. The daily Option chains contain the prices, volume, open interest, implied volaility, and Greeks of each contract.
# DataFrame format history_df = qb.history(option.symbol, datetime(2024, 1, 1), datetime(2024, 1, 5), flatten=True) # OptionUniverse objects history = qb.history[OptionUniverse](option.symbol, datetime(2024, 1, 1), datetime(2024, 1, 5)) for chain in history: end_time = chain.end_time filtered_chain = [contract for contract in chain if contract.greeks.delta > 0.3] for contract in filtered_chain: price = contract.price iv = contract.implied_volatility
var history = qb.History<OptionUniverse>(option.Symbol, new DateTime(2024, 1, 1), new DateTime(2024, 1, 5)); foreach (var chain in history) { var endTime = chain.EndTime; var filteredChain = chain.Data .Select(contract => contract as OptionUniverse) .Where(contract => contract.Greeks.Delta > 0.3m); foreach (var contract in filteredChain) { var price = contract.Price; var iv = contract.ImpliedVolatility; } }
The method represents each contract with an OptionUniverse
object, which have the following properties: