Index Options
Individual Contracts
Introduction
This page explains how to request historical data for individual Index Option contracts. The history requests on this page only return the prices and open interest of the Option contracts, not their implied volatility or Greeks. For information about history requests that return the daily implied volatility and Greeks, see Universes.
Create Subscriptions
Follow these steps to subscribe to individual Index Option contracts:
- Create a
QuantBook
. - Add the underlying Index.
- Set the start date to a date in the past that you want to use as the analysis date.
- Call the
option_chain
method with the underlyingIndex
Symbol
. - Sort and filter the data to select the specific contract(s) you want to analyze.
- Call the
add_index_option_contract
method with anOptionContract
Symbol
and disable fill-forward.
qb = QuantBook()
underlying_symbol = qb.add_index("SPX", Resolution.MINUTE).symbol
To view the available Indices, see Supported Assets.
If you do not pass a resolution argument, Resolution.MINUTE
is used by default.
qb.set_start_date(2024, 1, 1)
The method that you call in the next step returns data on all the contracts that were tradable on this date.
# Get the Option contracts that were tradable on January 1st, 2024. # Option A: Standard contracts. chain = qb.option_chain( Symbol.create_canonical_option(underlying_symbol, Market.USA, "?SPX"), flatten=True ).data_frame # Option B: Weekly contracts. #chain = qb.option_chain( # Symbol.create_canonical_option(underlying_symbol, "SPXW", Market.USA, "?SPXW"), flatten=True #).data_frame
This method returns an OptionChain
object, which represent an entire chain of Option contracts for a single underlying security.
You can even format the chain data into a DataFrame where each row in the DataFrame represents a single contract.
# Select a contract. expiry = chain.expiry.min() contract_symbol = chain[ # Select call contracts with the closest expiry. (chain.expiry == expiry) & (chain.right == OptionRight.CALL) & # Select contracts with a 0.3-0.7 delta. (chain.delta > 0.3) & (chain.delta < 0.7) # Select the contract with the largest open interest. ].sort_values('openinterest').index[-1]
option_contract = qb.add_index_option_contract(contract_symbol, fill_forward=False)
Disable fill-forward because there are only a few OpenInterest
data points per day.
Trade History
TradeBar
objects are price bars that consolidate individual trades from the exchanges. They contain the open, high, low, close, and volume of trading activity over a period of time.
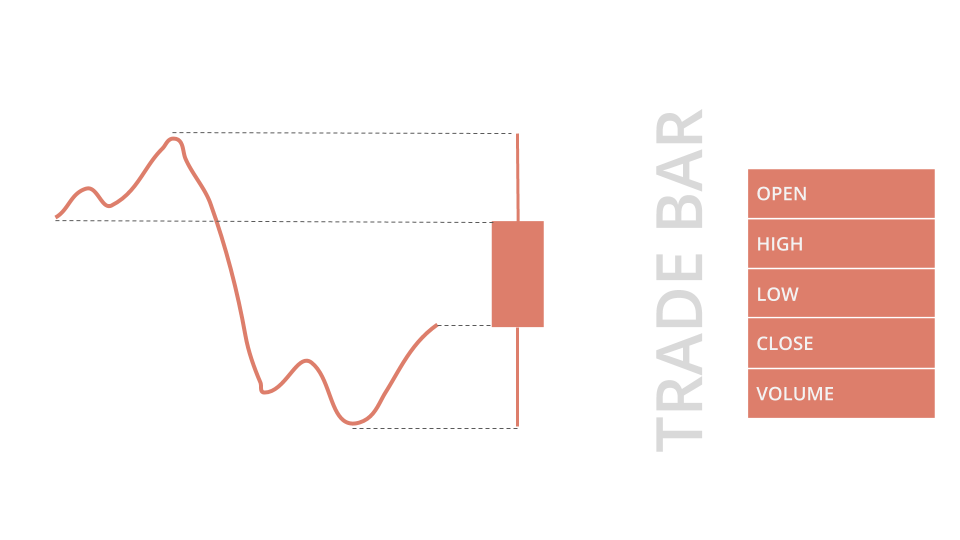
To get trade data, call the history
or history[TradeBar]
method with the contract Symbol
object(s).
# DataFrame format history_df = qb.history(TradeBar, contract_symbol, timedelta(3)) display(history_df) # TradeBar objects history = qb.history[TradeBar](contract_symbol, timedelta(3)) for trade_bar in history: print(trade_bar)
TradeBar
objects have the following properties:
Quote History
QuoteBar
objects are bars that consolidate NBBO quotes from the exchanges. They contain the open, high, low, and close prices of the bid and ask. The open
, high
, low
, and close
properties of the QuoteBar
object are the mean of the respective bid and ask prices. If the bid or ask portion of the QuoteBar
has no data, the open
, high
, low
, and close
properties of the QuoteBar
copy the values of either the bid
or ask
instead of taking their mean.
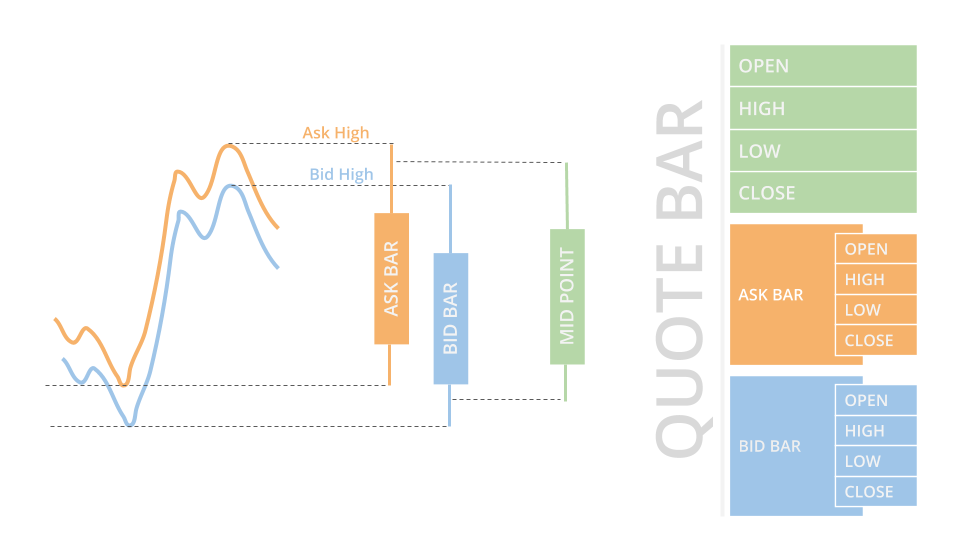
To get quote data, call the history
or history[QuoteBar]
method with the contract Symbol
object(s).
# DataFrame format history_df = qb.history(QuoteBar, contract_symbol, timedelta(3)) display(history_df) # QuoteBar objects history = qb.history[QuoteBar](contract_symbol, timedelta(3)) for quote_bar in history: print(quote_bar)
QuoteBar
objects have the following properties:
Open Interest History
Open interest is the number of outstanding contracts that haven't been settled. It provides a measure of investor interest and the market liquidity, so it's a popular metric to use for contract selection. Open interest is calculated once per day.
To get open interest data, call the history
or history[OpenInterest]
method with the contract Symbol
object(s).
# DataFrame format history_df = qb.history(OpenInterest, contract_symbol, timedelta(3)) display(history_df) # OpenInterest objects history = qb.history[OpenInterest](contract_symbol, timedelta(3)) for open_interest in history: print(open_interest)
OpenInterest
objects have the following properties:
Greeks and IV History
The Greeks are measures that describe the sensitivity of an Option's price to various factors like underlying price changes (Delta), time decay (Theta), volatility (Vega), and interest rates (Rho), while Implied Volatility (IV) represents the market's expectation of the underlying asset's volatility over the life of the Option.
Follow these steps to get the Greeks and IV data:
- Create the mirror contract
Symbol
. - Set up the risk free interest rate, dividend yield, and Option pricing models.
- Define a method to return the IV & Greeks indicator values for each contract.
- Call the preceding method and display the results.
mirror_contract_symbol = Symbol.create_option( option_contract.underlying.symbol, contract_symbol.id.market, option_contract.style, OptionRight.Call if option_contract.right == OptionRight.PUT else OptionRight.PUT, option_contract.strike_price, option_contract.expiry )
In our research, we found the Forward Tree model to be the best pricing model for indicators.
risk_free_rate_model = qb.risk_free_interest_rate_model dividend_yield_model = DividendYieldProvider(underlying_symbol) option_model = OptionPricingModelType.FORWARD_TREE
def greeks_and_iv(contracts, period, risk_free_rate_model, dividend_yield_model, option_model): # Get the call and put contract. call, put = sorted(contracts, key=lambda s: s.id.option_right) def get_values(indicator_class, contract, mirror_contract): return qb.indicator_history( indicator_class(contract, risk_free_rate_model, dividend_yield_model, mirror_contract, option_model), [contract, mirror_contract, contract.underlying], period ).data_frame.current return pd.DataFrame({ 'iv_call': get_values(ImpliedVolatility, call, put), 'iv_put': get_values(ImpliedVolatility, put, call), 'delta_call': get_values(Delta, call, put), 'delta_put': get_values(Delta, put, call), 'gamma_call': get_values(Gamma, call, put), 'gamma_put': get_values(Gamma, put, call), 'rho_call': get_values(Rho, call, put), 'rho_put': get_values(Rho, put, call), 'vega_call': get_values(Vega, call, put), 'vega_put': get_values(Vega, put, call), 'theta_call': get_values(Theta, call, put), 'theta_put': get_values(Theta, put, call), })
greeks_and_iv([contract_symbol, mirror_contract_symbol], 15, risk_free_rate_model, dividend_yield_model, option_model)
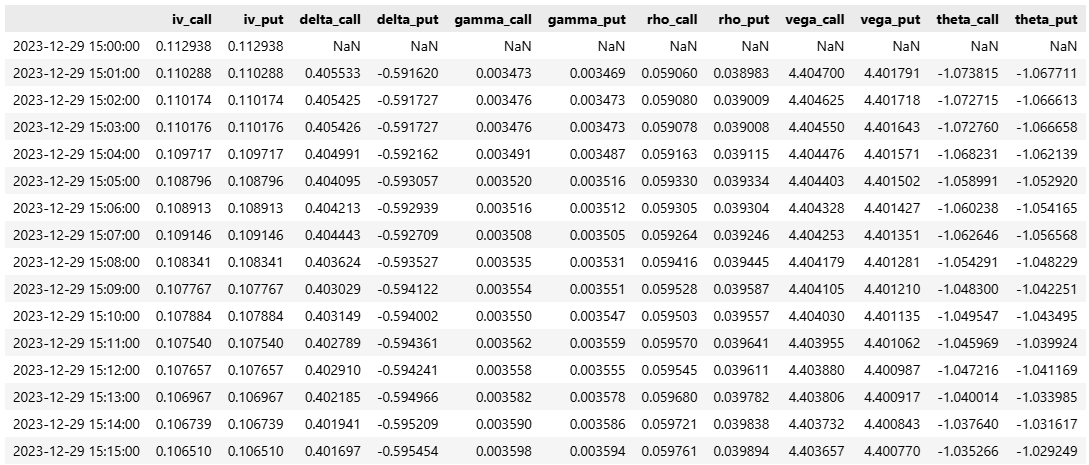
The DataFrame can have NaN entries if there is no data for the contracts or the underlying asset at a moment in time.
Examples
The following examples demonstrate some common practices for analyzing individual Index Option contracts in the Research Environment.
Example 1: Contract Trade History
The following notebook plots the historical prices of an SPX Index Option contract using Plotly :
import plotly.graph_objects as go # Get the SPX Option chain for January 1, 2024. qb = QuantBook() underlying_symbol = qb.add_index("SPX").symbol qb.set_start_date(2024, 1, 1) chain = qb.option_chain( Symbol.create_canonical_option(underlying_symbol, Market.USA, "?SPX"), flatten=True ).data_frame # Select a contract from the chain. expiry = chain.expiry.min() contract_symbol = chain[ (chain.expiry == expiry) & (chain.right == OptionRight.CALL) & (chain.delta > 0.3) & (chain.delta < 0.7) ].sort_values('openinterest').index[-1] # Add the target contract. qb.add_index_option_contract(contract_symbol) # Get the contract history. history = qb.history(contract_symbol, timedelta(3)) # Plot the price history. go.Figure( data=go.Candlestick( x=history.index.levels[4], open=history['open'], high=history['high'], low=history['low'], close=history['close'] ), layout=go.Layout( title=go.layout.Title(text=f'{contract_symbol.value} OHLC'), xaxis_title='Date', yaxis_title='Price', xaxis_rangeslider_visible=False ) ).show()
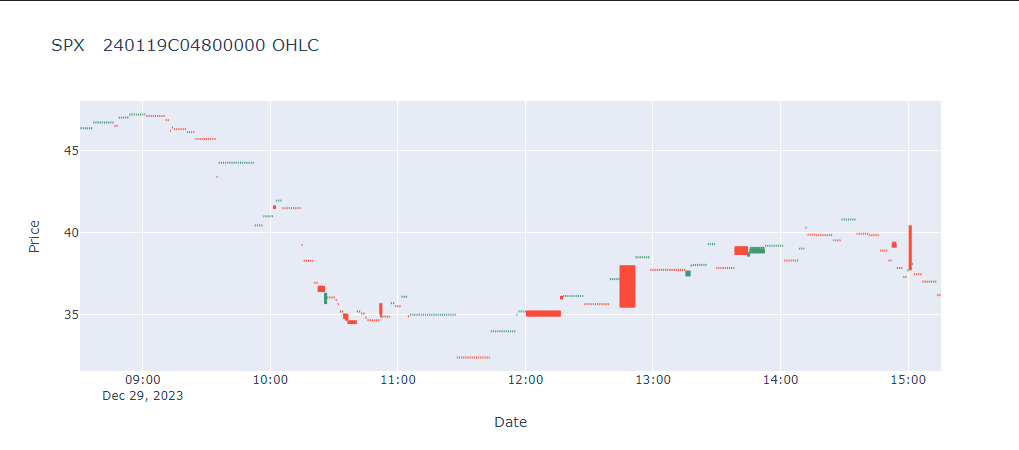
Example 2: Contract Open Interest History
The following notebook plots the historical open interest of a VIXW Index Option contract using Matplotlib :
# Get the VIX weekly Option chain for January 1, 2024. qb = QuantBook() underlying_symbol = qb.add_index("VIX").symbol qb.set_start_date(2024, 1, 1) chain = qb.option_chain( Symbol.create_canonical_option(underlying_symbol, "VIXW", Market.USA, "?VIXW"), flatten=True ).data_frame # Select a contract from the chain. strike_distance = (chain.strike - chain.underlyinglastprice).abs() target_strike_distance = strike_distance.min() chain = chain.loc[strike_distance[strike_distance == target_strike_distance].index] contract_symbol = chain.sort_values('openinterest').index[-1] # Add the target contract. qb.add_index_option_contract(contract_symbol, fill_forward=False) # Get the contract's open interest history. history = qb.history(OpenInterest, contract_symbol, timedelta(90)) history.index = history.index.droplevel([0, 1, 2]) history = history['openinterest'].unstack(0)[contract_symbol] # Plot the open interest history. history.plot(title=f'{contract_symbol.value} Open Interest') plt.show()
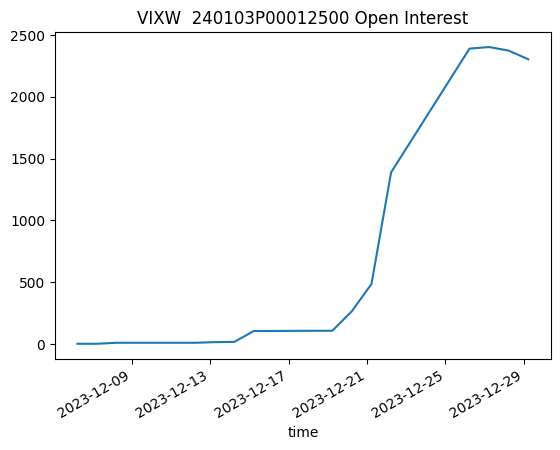