Popular Libraries
Keras
Get Historical Data
Get some historical market data to train and test the model. For example, to get data for the SPY ETF during 2020 and 2021, run:
qb = QuantBook() symbol = qb.add_equity("SPY", Resolution.DAILY).symbol history = qb.history(symbol, datetime(2020, 1, 1), datetime(2022, 1, 1)).loc[symbol]
Prepare Data
You need some historical data to prepare the data for the model. If you have historical data, manipulate it to train and test the model. In this example, use the following features and labels:
Data Category | Description |
---|---|
Features | Daily percent change of the open, high, low, close, and volume of the SPY over the last 5 days |
Labels | Daily percent return of the SPY over the next day |
The following image shows the time difference between the features and labels:
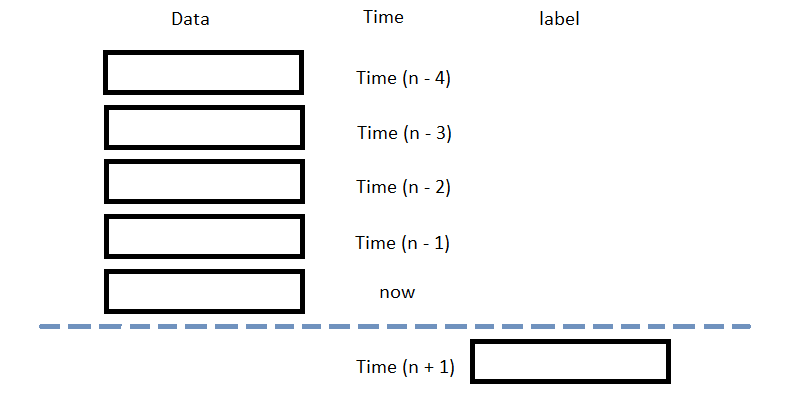
Follow these steps to prepare the data:
- Call the
pct_change
anddropna
methods. - Loop through the
daily_pct_change
DataFrame and collect the features and labels. - Convert the lists of features and labels into
numpy
arrays. - Split the data into training and testing periods.
daily_pct_change = history.pct_change().dropna()
n_steps = 5
features = [] labels = [] for i in range(len(daily_pct_change)-n_steps): features.append(daily_pct_change.iloc[i:i+n_steps].values) labels.append(daily_pct_change['close'].iloc[i+n_steps])
features = np.array(features) labels = np.array(labels)
train_length = int(len(features) * 0.7) X_train = features[:train_length] X_test = features[train_length:] y_train = labels[:train_length] y_test = labels[train_length:]
Train Models
You need to prepare the historical data for training before you train the model. If you have prepared the data, build and train the model. In this example, build a neural network model that predicts the future return of the SPY. Follow these steps to create the model:
- Call the
Sequential
constructor with a list of layers. - Call the
compile
method with a loss function, an optimizer, and a list of metrics to monitor. - Call the
fit
method with the features and labels of the training dataset and a number of epochs.
model = Sequential([Dense(10, input_shape=(5,5), activation='relu'), Dense(10, activation='relu'), Flatten(), Dense(1)])
Set the input_shape
of the first layer to (5, 5)
because each sample contains the percent change of 5 factors (percent change of the open, high, low, close, and volume) over the previous 5 days. Call the Flatten
constructor because the input is 2-dimensional but the output is just a single value.
model.compile(loss='mse', optimizer=RMSprop(0.001), metrics=['mae', 'mse'])
model.fit(X_train, y_train, epochs=5)
Test Models
You need to build and train the model before you test its performance. If you have trained the model, test it on the out-of-sample data. Follow these steps to test the model:
- Call the
predict
method with the features of the testing period. - Plot the actual and predicted labels of the testing period.
y_hat = model.predict(X_test)
results = pd.DataFrame({'y': y_test.flatten(), 'y_hat': y_hat.flatten()}) results.plot(title='Model Performance: predicted vs actual %change in closing price')
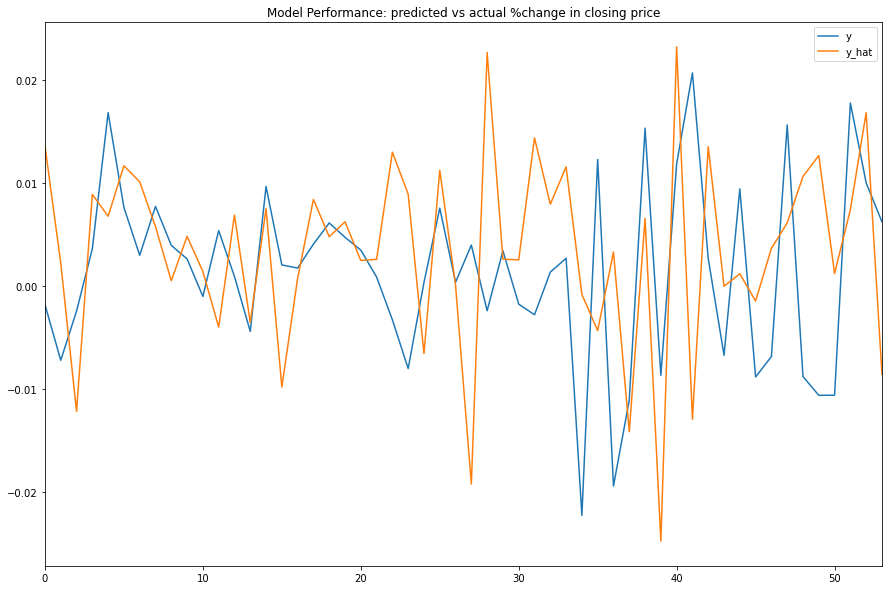
Store Models
You can save and load keras
models using the Object Store.
Save Models
Follow these steps to save models in the Object Store:
- Set the key name of the model to be stored in the Object Store.
- Call the
GetFilePath
get_file_path
method with the key. - Call the
save
method the file path.
The key must end with a .keras extension for the native Keras format (recommended) or a .h5 extension.
model_key = "model.keras"
file_name = qb.object_store.get_file_path(model_key)
This method returns the file path where the model will be stored.
model.save(file_name)
Load Models
You must save a model into the Object Store before you can load it from the Object Store. If you saved a model, follow these steps to load it:
- Call the
ContainsKey
contains_key
method with the model key. - Call the
GetFilePath
get_file_path
method with the key name. - Call the
load_model
method with the file path.
qb.object_store.contains_key(model_key)
This method returns a boolean that represents if the model_key
is in the Object Store. If the Object Store does not contain the model_key
, save the model using the model_key
before you proceed.
file_name = qb.object_store.get_file_path(model_key)
This method returns the path where the model is stored.
loaded_model = load_model(file_name)
This method returns the saved model.
Examples
The following examples demonstrate some common practices for using the Keras library.
Example 1: Predict Next Return
The following research notebook uses Keras
machine learning model to predict the next day's return by the previous 5 days' daily returns.
# Import the Keras library. from tensorflow.keras import utils, models from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Dense, Flatten from tensorflow.keras.optimizers import RMSprop from tensorflow.keras.saving import load_model # Instantiate the QuantBook for researching. qb = QuantBook() # Request the daily SPY history with the date range to be studied. symbol = qb.add_equity("SPY", Resolution.DAILY).symbol history = qb.history(symbol, datetime(2020, 1, 1), datetime(2022, 1, 1)).loc[symbol] # Obtain the daily returns to be the features and labels. daily_returns = history['close'].pct_change()[1:] # We use the previous 5 day returns as the features to be studied. # Get the 1-day forward return as the labels for the machine to learn. n_steps = 5 features = [] labels = [] for i in range(len(daily_returns)-n_steps): features.append(daily_returns.iloc[i:i+n_steps].values) labels.append(daily_returns.iloc[i+n_steps]) # Split the data as a training set and test set for validation. In this example, we use 70% of the data points to train the model and test with the rest. features = np.array(features) labels = np.array(labels) train_length = int(len(features) * 0.7) X_train = features[:train_length] X_test = features[train_length:] y_train = labels[:train_length] y_test = labels[train_length:] # Call the Sequential constructor with a list of layers to create the model. model = Sequential([Dense(10, input_shape=(5,5), activation='relu'), Dense(10, activation='relu'), Flatten(), Dense(1)]) # Call the compile method with a loss function, an optimizer, and a list of metrics to monitor to set how should the model be fitted. model.compile(loss='mse', optimizer=RMSprop(0.001), metrics=['mae', 'mse']) # Call the fit method with the features and labels of the training dataset and a number of epochs to fit the model. model.fit(X_train, y_train, epochs=5) # Call the predict method with the features of the testing period. y_hat = model.predict(X_test) # Plot the actual and predicted labels of the testing period. results = pd.DataFrame({'y': y_test.flatten(), 'y_hat': y_hat.flatten()}) results.plot(title='Model Performance: predicted vs actual %change in closing price') # Store the model in the object store to allow accessing the model in the next research session or in the algorithm for trading. model_key = "model.keras" file_name = qb.object_store.get_file_path(model_key) model.save(file_name)