Charting
Seaborn
Import Libraries
Follow these steps to import the libraries that you need:
- Import the
seaborn
andmatplotlib
libraries. - Import, and then call, the
register_matplotlib_converters
method.
import seaborn as sns import matplotlib.pyplot as plt
from pandas.plotting import register_matplotlib_converters register_matplotlib_converters()
Get Historical Data
Get some historical market data to produce the plots. For example, to get data for a bank sector ETF and some banking companies over 2021, run:
qb = QuantBook() tickers = ["XLF", # Financial Select Sector SPDR Fund "COF", # Capital One Financial Corporation "GS", # Goldman Sachs Group, Inc. "JPM", # J P Morgan Chase & Co "WFC"] # Wells Fargo & Company symbols = [qb.add_equity(ticker, Resolution.DAILY).symbol for ticker in tickers] history = qb.history(symbols, datetime(2021, 1, 1), datetime(2022, 1, 1))
Create Candlestick Chart
Seaborn does not currently support candlestick charts. Use one of the other plotting libraries to create candlestick charts.
Create Line Chart
You must import the plotting libraries and get some historical data to create line charts.
In this example, you create a line chart that shows the closing price for one of the banking securities. Follow these steps to create the chart:
- Select a
Symbol
. - Slice the
history
DataFrame with thesymbol
and then select the close column. - Call the
DataFrame
constructor with thedata Series
and then call thereset_index
method. - Call the
lineplot
method with thedata Series
and the column name of each axis. - In the same cell that you called the
lineplot
method, call theset
method with the y-axis label and a title.
symbol = symbols[0]
data = history.loc[symbol]['close']
data = pd.DataFrame(data).reset_index()
plot = sns.lineplot(data=data, x='time', y='close')
plot.set(ylabel="price", title=f"{symbol} Price Over Time");
The Jupyter Notebook displays the line chart.
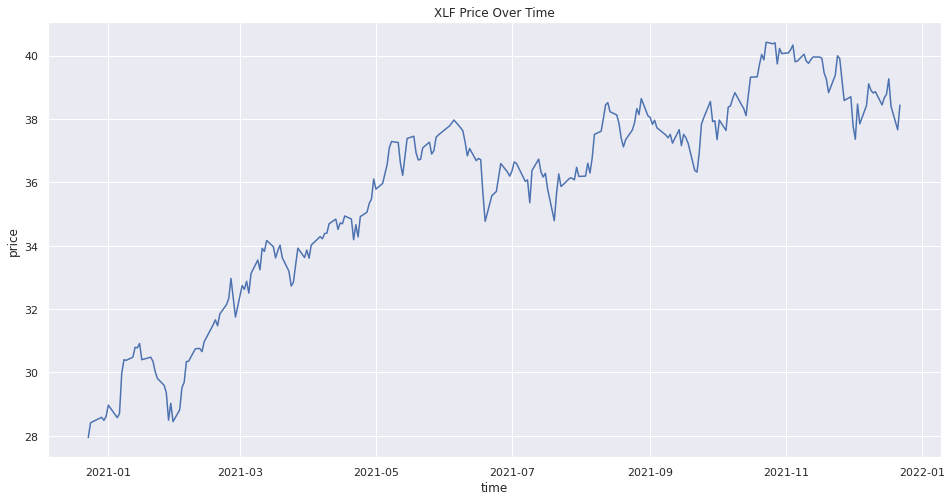
Create Scatter Plot
You must import the plotting libraries and get some historical data to create scatter plots.
In this example, you create a scatter plot that shows the relationship between the daily returns of two banking securities. Follow these steps to create the scatter plot:
- Select 2
Symbol
s. - Select the close column of the
history
DataFrame, call theunstack
method, and then select thesymbol1
andsymbol2
columns. - Call the
pct_change
method and then call thedropna
method. - Call the
regplot
method with thedaily_returns DataFrame
and the column names. - In the same cell that you called the
regplot
method, call theset
method with the axis labels and a title.
For example, to select the Symbol
s of the first 2 bank stocks, run:
symbol1 = symbols[1] symbol2 = symbols[2]
close_prices = history['close'].unstack(0)[[symbol1, symbol2]]
daily_returns = close_prices.pct_change().dropna()
plot = sns.regplot(data=daily_returns, x=daily_returns.columns[0], y=daily_returns.columns[1])
plot.set(xlabel=f'{daily_returns.columns[0]} % Returns', ylabel=f'{daily_returns.columns[1]} % Returns', title=f'{symbol1} vs {symbol2} Daily % Returns');
The Jupyter Notebook displays the scatter plot.
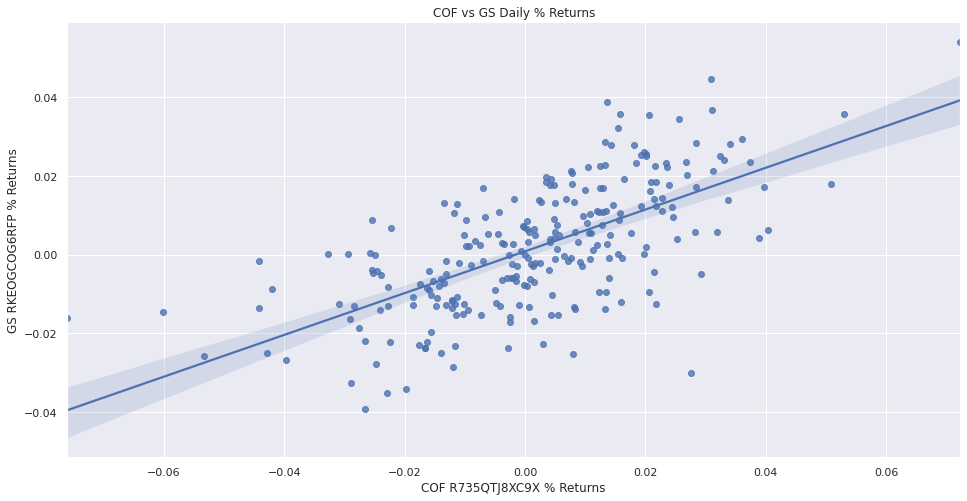
Create Histogram
You must import the plotting libraries and get some historical data to create histograms.
In this example, you create a histogram that shows the distribution of the daily percent returns of the bank sector ETF. Follow these steps to create the histogram:
- Select the
Symbol
. - Slice the
history
DataFrame with thesymbol
and then select the close column. - Call the
pct_change
method and then call thedropna
method. - Call the
DataFrame
constructor with thedaily_returns Series
and then call thereset_index
method. - Call the
histplot
method with thedaily_returns
, the close column name, and the number of bins. - In the same cell that you called the
histplot
method, call theset
method with the axis labels and a title.
symbol = symbols[0]
data = history.loc[symbol]['close']
daily_returns = data.pct_change().dropna()
daily_returns = pd.DataFrame(daily_returns).reset_index()
plot = sns.histplot(daily_returns, x='close', bins=20)
plot.set(xlabel='Return', ylabel='Frequency', title=f'{symbol} Daily Return of Close Price Distribution');
The Jupyter Notebook displays the histogram.
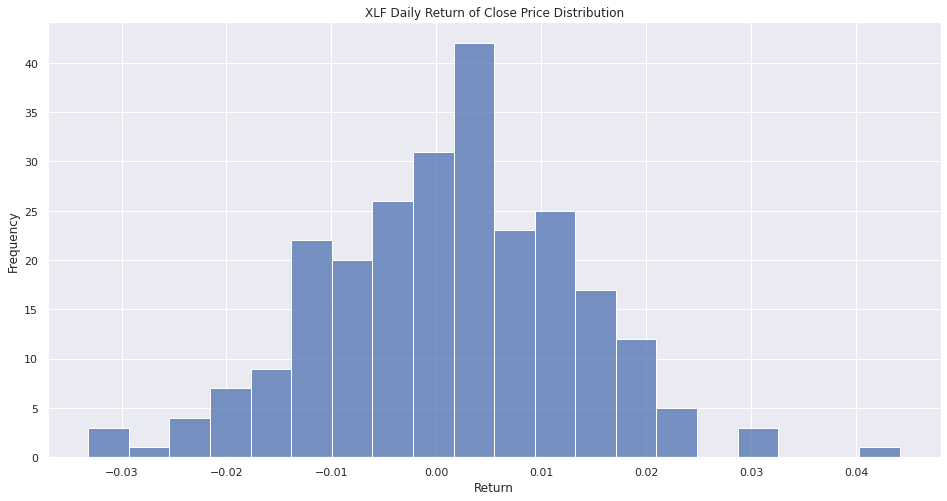
Create Bar Chart
You must import the plotting libraries and get some historical data to create bar charts.
In this example, you create a bar chart that shows the average daily percent return of the banking securities. Follow these steps to create the bar chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method and then multiply by 100. - Call the
mean
method. - Call the
DataFrame
constructor with theavg_daily_returns
Series
and then call thereset_index
method. - Call
barplot
method with theavg_daily_returns
Series
and the axes column names. - In the same cell that you called the
barplot
method, call theset
method with the axis labels and a title. - In the same cell that you called the
set
method, call thetick_params
method to rotate the x-axis labels.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change() * 100
avg_daily_returns = daily_returns.mean()
avg_daily_returns = pd.DataFrame(avg_daily_returns, columns=["avg_daily_ret"]).reset_index()
plot = sns.barplot(data=avg_daily_returns, x='symbol', y='avg_daily_ret')
plot.set(xlabel='Tickers', ylabel='%', title='Banking Stocks Average Daily % Returns')
plot.tick_params(axis='x', rotation=90)
The Jupyter Notebook displays the bar chart.
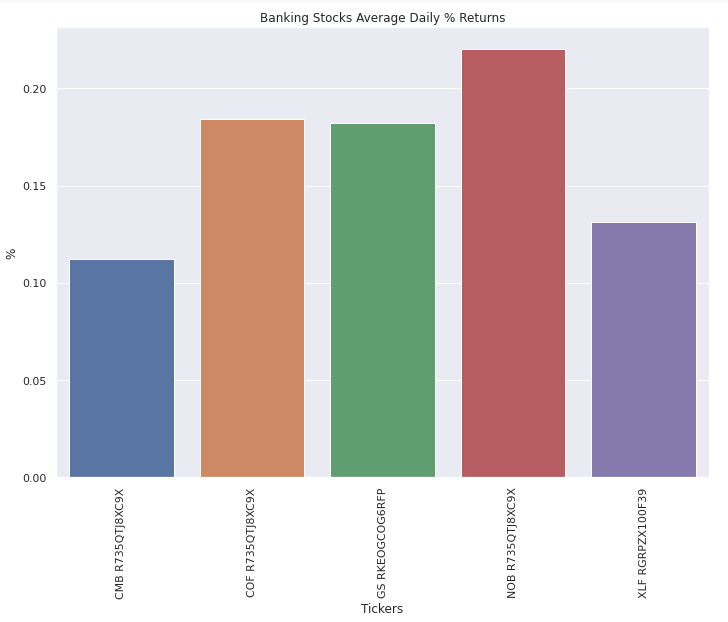
Create Heat Map
You must import the plotting libraries and get some historical data to create heat maps.
In this example, you create a heat map that shows the correlation between the daily returns of the banking securities. Follow these steps to create the heat map:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call the
corr
method. - Call the
heatmap
method with thecorr_matrix
and the annotation argument enabled. - In the same cell that you called the
heatmap
method, call theset
method with a title.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
corr_matrix = daily_returns.corr()
plot = sns.heatmap(corr_matrix, annot=True)
plot.set(title='Bank Stocks and Bank Sector ETF Correlation Coefficients');
The Jupyter Notebook displays the heat map.
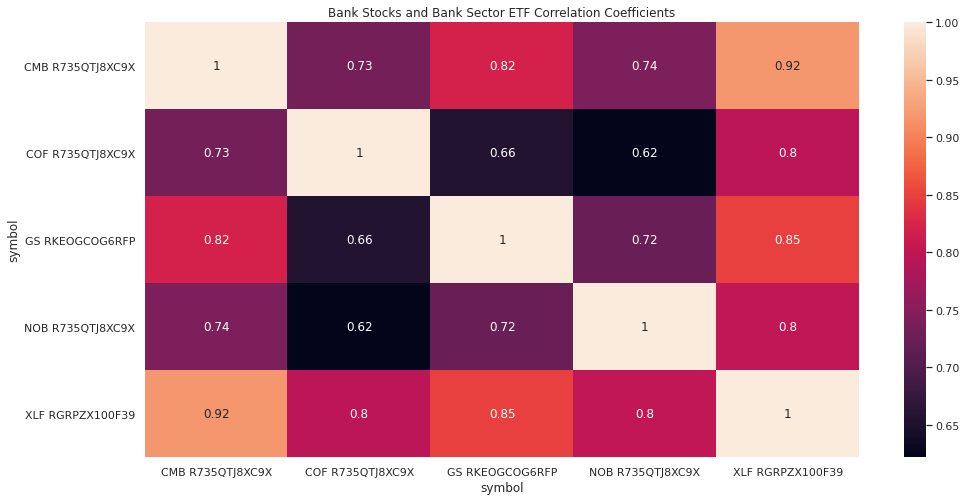
Create Pie Chart
You must import the plotting libraries and get some historical data to create pie charts.
In this example, you create a pie chart that shows the weights of the banking securities in a portfolio if you allocate to them based on their inverse volatility. Follow these steps to create the pie chart:
- Select the close column and then call the
unstack
method. - Call the
pct_change
method. - Call
var
method and then take the inverse. - Call the
color_palette
method with a palette name and then truncate the returned colors to so that you have one color for each security. - Call the
pie
method with the security weights, labels, and colors. - In the same cell that you called the
pie
method, call thetitle
method with a title.
close_prices = history['close'].unstack(level=0)
daily_returns = close_prices.pct_change()
inverse_variance = 1 / daily_returns.var()
colors = sns.color_palette('pastel')[:len(inverse_variance.index)]
plt.pie(inverse_variance, labels=inverse_variance.index, colors=colors, autopct='%1.1f%%')
plt.title(title='Banking Stocks and Bank Sector ETF Allocation');
The Jupyter Notebook displays the pie chart.
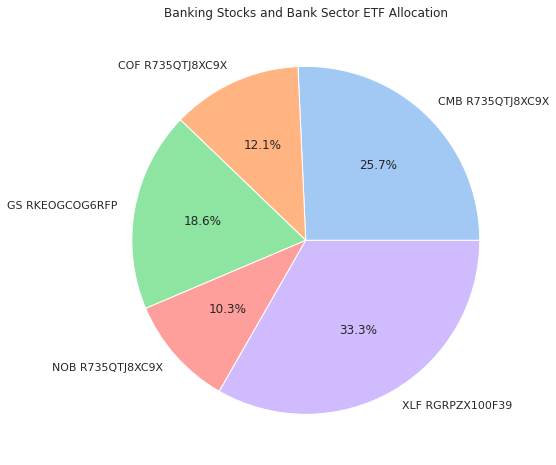