Futures Options
Universes
Create Subscriptions
Follow these steps to subscribe to a Futures Options universe:
- Load the assembly files and data types in their own cell.
- Import the data types.
- Create a
QuantBook
. - Add the underlying Future.
#load "../Initialize.csx"
#load "../QuantConnect.csx" #r "../Microsoft.Data.Analysis.dll" using QuantConnect; using QuantConnect.Data; using QuantConnect.Algorithm; using QuantConnect.Research; using QuantConnect.Indicators; using QuantConnect.Securities; using QuantConnect.Data.UniverseSelection; using Microsoft.Data.Analysis;
var qb = new QuantBook();
qb = QuantBook()
var future = qb.AddFuture(Futures.Indices.SP500EMini);
future = qb.add_future(Futures.Indices.SP_500_E_MINI)
To view the available underlying Futures in the US Future Options dataset, see Supported Assets.
Price History
The contract filter determines which Future Option contracts are in your universe each trading day. The default filter selects the contracts with the following characteristics:
- Standard type (weeklies and non-standard contracts are not available)
- Within 1 strike price of the underlying asset price
- Expire within 35 days
To get the prices and volumes for all of the Future Option contracts that pass your filter during a specific period of time, get the underlying Future contract and then call the OptionHistory
option_history
method with the Future contract's Symbol
object, a start DateTime
datetime
, and an end DateTime
datetime
.
start_date = datetime(2024, 1, 1) # Select an underlying Futures contract. For example, get the front-month contract. futures_contract = sorted( qb.future_chain_provider.get_future_contract_list(future.symbol, start_date), key=lambda symbol: symbol.id.date )[0] # Get the Options data for the selected Futures contract. option_history = qb.option_history( futures_contract, start_date, futures_contract.id.date, Resolution.HOUR, fill_forward=False, extended_market_hours=False )
var startDate = new DateTime(2024, 1, 1); // Select an underlying Futures contract. For example, get the front-month contract. var futuresContract = qb.FutureChainProvider.GetFutureContractList(future.Symbol, startDate) .OrderBy(symbol => symbol.ID.Date) .Last(); // Get the Options data for the selected Futures contract. var optionHistory = qb.OptionHistory( futuresContract, startDate, futuresContract.ID.Date, Resolution.Hour, fillForward: false, extendedMarketHours: false );
To convert the OptionHistory
object to a DataFrame
that contains the trade and quote information of each contract and the underlying, use the data_frame
property.
option_history.data_frame
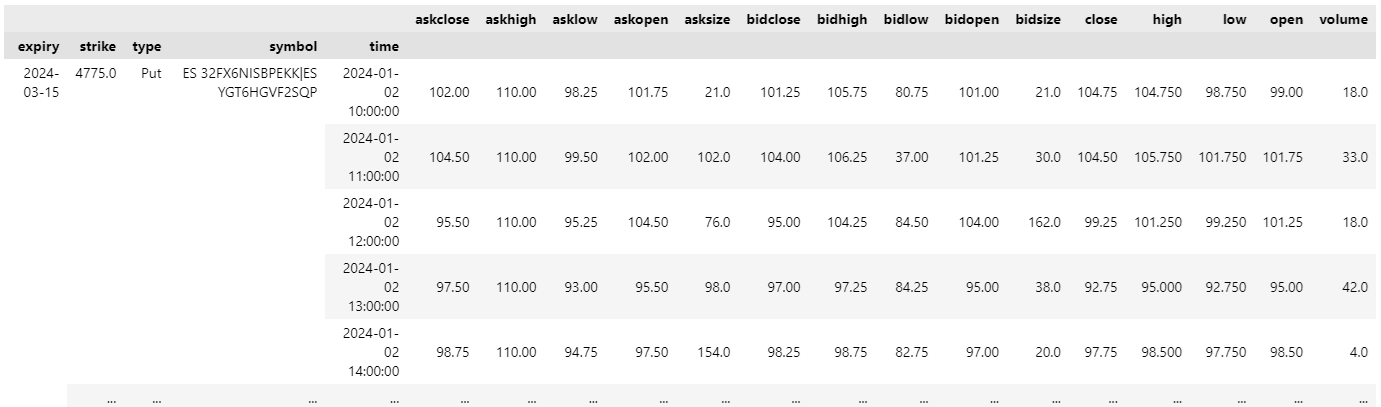
To get the expiration dates of all the contracts in an OptionHistory
object, call the GetExpiryDates
get_expiry_dates
method.
option_history.get_expiry_dates()

To get the strike prices of all the contracts in an OptionHistory
object, call the GetStrikes
get_strikes
method.
option_history.get_strikes()
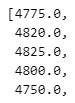