Optimization
Parameters
Introduction
Parameters are project variables that your algorithm uses to define the value of internal variables like indicator arguments or the length of lookback windows. Parameters are stored outside of your algorithm code, but we inject the values of the parameters into your algorithm when you run a backtest, deploy a live algorithm, or launch an optimization job. To use parameters, set some parameters in your project and then load them into your algorithm.
Get Parameters
To get a parameter value into your algorithm, call the GetParameter
get_parameter
method of the algorithm class.
// Get the parameter string-value with this matching key. // Project parameters are useful for optimization or securing variables outside the source-code. var parameterValue = GetParameter("Parameter Name");
# Get the parameter string-value with this matching key. # Project parameters are useful for optimization or securing variables outside the source-code. parameter_value = self.get_parameter("Parameter Name")
The GetParameter
get_parameter
method returns a string by default. If you provide a default parameter value, the method returns the parameter value as the same data type as the default value. If there are no parameters in your project that match the name you pass to the method and you provide a default value to the method, it returns the default value. The following table describes the arguments the GetParameter
get_parameter
method accepts:
Argument | Data Type | Description | Default Value |
---|---|---|---|
name | string str | The name of the parameter to get | |
defaultValue | string/int/double/decimal | The default value to return | null |
default_value | str/int/double | The default value to return | None |
The following example algorithm gets the values of parameters of each data type:
// Example to get the values of parameters of each data type: namespace QuantConnect.Algorithm.CSharp { public class ParameterizedAlgorithm : QCAlgorithm { public override void Initialize() { // Get the parameter value and return an integer. var intParameterValue = GetParameter("intParameterName", 100); // Get the parameter value and return a double. var doubleParameterValue = GetParameter("doubleParameterName", 0.95); // Get the parameter value and return a decimal. var decimalParameterValue = GetParameter("decimalParameterName", 0.05m); // Get the parameter value as a string. var stringParameterValue = GetParameter("parameterName", "defaultStringValue") // Cast the string to an integer. var castedParameterValue = Convert.ToInt32(stringParameterValue); } } }
# Example to get the values of parameters of each data type: class ParameterizedAlgorithm(QCAlgorithm): def initialize(self) -> None: # Get the parameter value and return an integer. int_parameter_value = self.get_parameter("int_parameter_name", 100) # Get the parameter value and return a double. float_parameter_value = self.get_parameter("float_parameter_name", 0.95) # Get the parameter value as a string. string_parameter_value = self.get_parameter("parameter_name", "default_string_value") # Cast the string to an integer. parameter_value = int(string_parameter_value)
Alternatively, you can use the Parameter(name)
attribute on top of class fields or properties to set their values. If there are no parameters in your project that match the name you pass to the attribute and you provide a default value to the method, it returns the default value.
// Use the Parameter attribute to set field values externally, enabling flexible configuration with default fallback. namespace QuantConnect.Algorithm.CSharp { public class ParameterizedAlgorithm : QCAlgorithm { [Parameter("intParameterName")] public int IntParameterValue = 100; [Parameter("doubleParameterName")] public double DoubleParameterValue = 0.95; [Parameter("decimalParameterName")] public decimal DecimalParameterValue = 0.05m; } }
The parameter values are sent to your algorithm when you deploy the algorithm, so it's not possible to change the parameter values while the algorithm runs.
Overfitting
Overfitting occurs when a function is fit too closely fit to a limited set of training data. Overfitting can occur in your trading algorithms if you have many parameters or select parameters values that worked very well in the past but are sensitive to small changes in their values. In these cases, your algorithm will likely be fine-tuned to fit the detail and noise of the historical data to the extent that it negatively impacts the live performance of your algorithm. The following image shows examples of underfit, optimally-fit, and overfit functions:
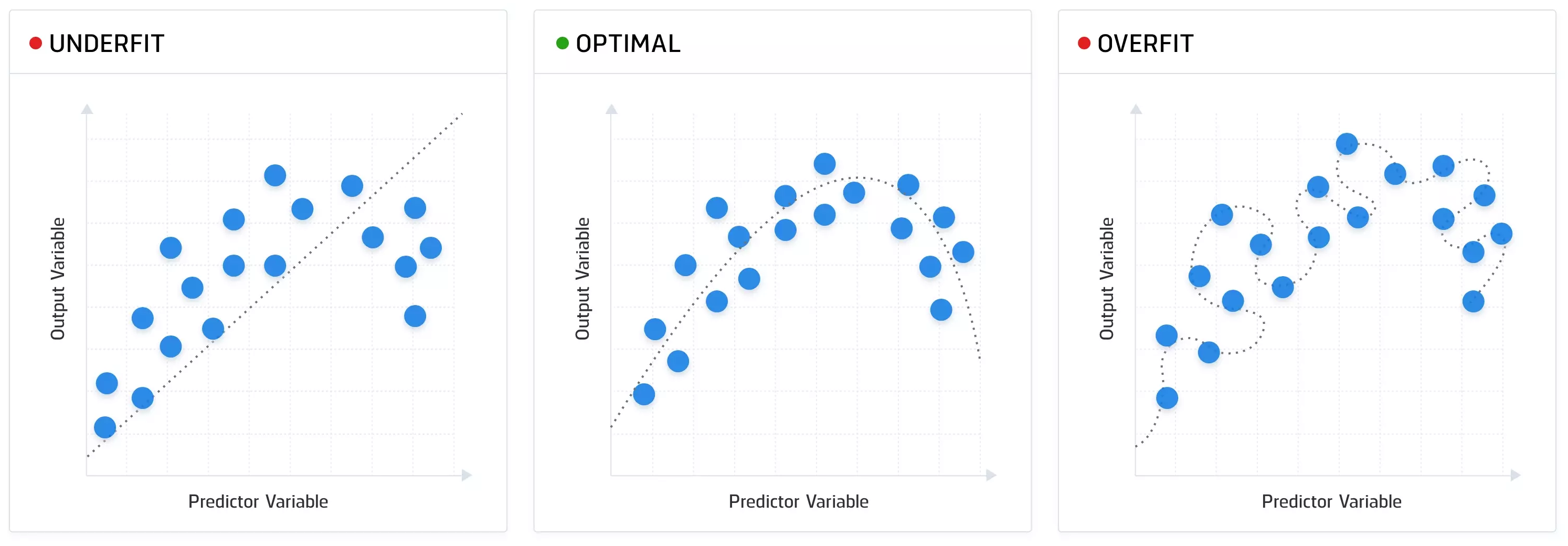
An algorithm that is dynamic and generalizes to new data is more likely to survive across different market conditions and apply to other markets.
Look-Ahead Bias
Look-ahead bias occurs when an algorithm makes decisions using data that would not have yet been available. For instance, in optimization jobs, you optimize a set of parameters over a historical backtesting period. After the optimizer finds the optimal parameter values, the backtest period becomes part of the in-sample data. If you run a backtest over the same period using the optimal parameters, look-ahead bias has seeped into your research. In reality, it would not be possible to know the optimal parameters during the testing period until after the testing period is over. To avoid issues with look-ahead bias, optimize on older historical data and test the optimal parameter values on recent historical data. Alternatively, apply walk forward optimization to optimize the parameters on smaller batches of history.
Live Trading Considerations
To update parameters in live mode, add a Schedule Event that downloads a remote file and uses its contents to update the parameter values.
private Dictionary_parameters = new(); public override void Initialize() { if (LiveMode) { Schedule.On( DateRules.EveryDay(), TimeRules.Every(TimeSpan.FromMinutes(1)), ()=> { var content = Download(urlToRemoteFile); // Convert content to _parameters }); } }
def initialize(self): self.parameters = { } if self.live_mode: def download_parameters(): content = self.download(url_to_remote_file) # Convert content to self.parameters self.schedule.on(self.date_rules.every_day(), self.time_rules.every(timedelta(minutes=1)), download_parameters)
Examples
The following example algorithm demonstrates loading parameter values with the GetParameter
get_parameter
method:
// Initialize a parameterized EMA-Cross algorithm. namespace QuantConnect.Algorithm.CSharp { public class ParameterizedAlgorithm : QCAlgorithm { private ExponentialMovingAverage _fast; private ExponentialMovingAverage _slow; public override void Initialize() { SetStartDate(2020, 1, 1); SetCash(100000); AddEquity("SPY"); // Get and store the EMA settings to local parameters. var fastPeriod = GetParameter("ema-fast", 100); var slowPeriod = GetParameter("ema-slow", 200); _fast = EMA("SPY", fastPeriod); _slow = EMA("SPY", slowPeriod); } } }
# Initialize a parameterized EMA-Cross algorithm. class ParameterizedAlgorithm(QCAlgorithm): def initialize(self) -> None: self.set_start_date(2020, 1, 1) self.set_cash(100000) self.add_equity("SPY") # Get and store the EMA settings to local parameters. fast_period = self.get_parameter("ema-fast", 100) slow_period = self.get_parameter("ema-slow", 200) self._fast = self.ema("SPY", fast_period) self._slow = self.ema("SPY", slow_period)
The following example algorithm demonstrates loading parameter values with the Parameter
attribute:
// Example algorithm for loading parameter values with the Parameter attribute. namespace QuantConnect.Algorithm.CSharp { public class ParameterizedAlgorithm : QCAlgorithm { [Parameter("ema-fast")] public int FastPeriod = 100; [Parameter("ema-slow")] public int SlowPeriod = 200; private ExponentialMovingAverage _fast; private ExponentialMovingAverage _slow; public override void Initialize() { SetStartDate(2020, 1, 1); SetCash(100000); AddEquity("SPY"); _fast = EMA("SPY", FastPeriod); _slow = EMA("SPY", SlowPeriod); } } }