Option Strategies
Bull Call Ladder
Introduction
Bull call ladder, also known as long call ladder, is a combination of a bull call spread and a short call with a higher strike price than the 2 legs of the call spread. All calls have the same underlying Equity and expiration date. This strategy profits from low volatility of the underlying asset. For instance, the underlying price stays similar to its current price.
Implementation
Follow these steps to implement the bull call ladder strategy:
- In the
initialize
method, set the start date, end date, cash, and Option universe. - In the
on_data
method, select the expiration and strikes of the contracts in the strategy legs. - In the
on_data
method, select the contracts and place the orders.
def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 22) self.set_cash(1000000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().call_ladder(30, 5, 0, -5))
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select the call Option contracts with the furthest expiry expiry = max([x.expiry for x in chain]) calls = [i for i in chain if i.expiry == expiry and i.right == OptionRight.CALL] if not calls: return # Select the strike prices from the remaining contracts strikes = sorted(set(x.strike for x in calls)) if len(strikes) < 3: return low_strike = strikes[0] middle_strike = strikes[1] high_strike = strikes[2]
Approach A: Call the OptionStrategies.bull_call_ladder
method with the details of each leg and then pass the result to the buy
method.
option_strategy = OptionStrategies.bull_call_ladder(self._symbol, low_strike, middle_strike, high_strike, expiry) self.buy(option_strategy, 1)
Approach B: Create a list of Leg
objects and then call the combo_market_order, combo_limit_order, or combo_leg_limit_order method.
low_strike_call = next(filter(lambda x: x.strike == low_strike, calls)) middle_strike_call = next(filter(lambda x: x.strike == middle_strike, calls)) high_strike_call = next(filter(lambda x: x.strike == high_strike, calls)) legs = [ Leg.create(low_strike_call.symbol, 1), Leg.create(middle_strike_call.symbol, -1), Leg.create(high_strike_call.symbol, -1) ] self.combo_market_order(legs, 1)
Strategy Payoff
The bull call spread is an limited-profit strategy. The payoff is
ClowT=(ST−Klow)+CmidT=(ST−Kmid)+ChighT=(ST−Khigh)+PayoffT=(ClowT−Clow0+Cmid0−CmidT+Chigh0−ChighT)×m−feeThe following chart shows the payoff at expiration:
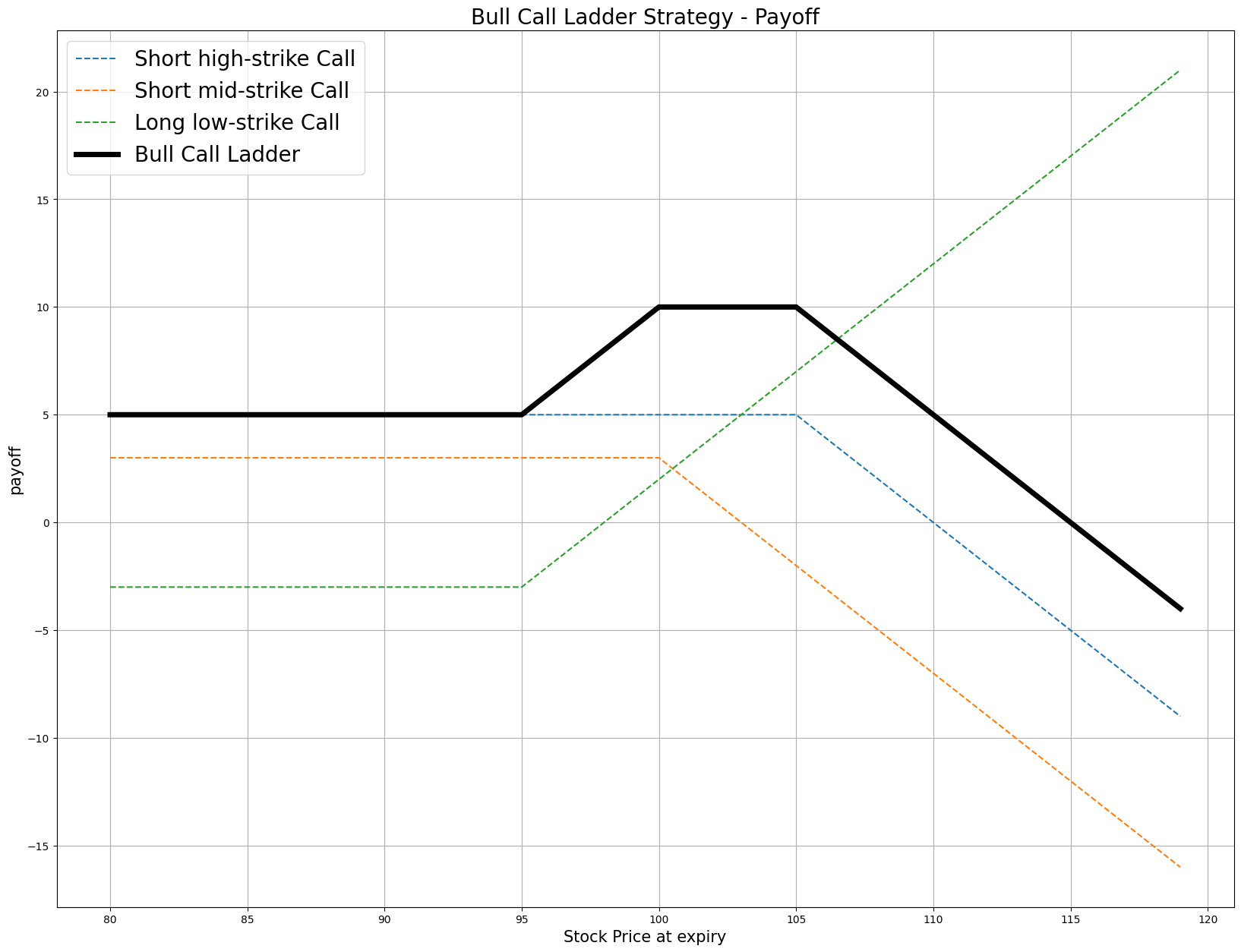
The maximum profit is Kmid−Klow−Clow0+Cmid0+Chigh0, which occurs when the underlying price is between the two higher strike prices.
The maximum loss is unlimited, which occurs when the underlying price increases indefinitely.
If the Option is American Option, there is a risk of early assignment on the contract you sell.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
Lower-Strike call | 17.10 | 822.50 |
Middle-strike call | 12.00 | 825.00 |
Higher-strike call | 10.90 | 827.50 |
Underlying Equity at expiration | 843.25 | - |
Therefore, the payoff is
ClowT=(ST−Klow)+=(843.25−822.50)+=20.75CmidT=(ST−Kmid)+=(843.25−825.00)+=18.25ChighT=(ST−Khigh)+=(843.25−827.50)+=15.75PayoffT=(ClowT−Clow0+Cmid0−CmidT+Chigh0−ChighT)×m−fee=(20.75−17.10+12.00−18.25+10.90−15.75)×100−1.00×3=−748So, the strategy loses $748.
The following algorithm implements a bull call ladder Option strategy:
class BullCallLadderOptionStrategy(QCAlgorithm): def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 23) self.set_cash(100000) option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol # set our strike/expiry filter for this option chain option.set_filter(lambda x: x.include_weeklys().call_ladder(30, 5, 0, -5)) def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select the call Option contracts with the furthest expiry expiry = max([x.expiry for x in chain]) calls = [i for i in chain if i.expiry == expiry and i.right == OptionRight.CALL] if not calls: return # Select the strike prices from the remaining contracts strikes = sorted(set(x.strike for x in calls)) if len(strikes) < 3: return low_strike = strikes[0] middle_strike = strikes[1] high_strike = strikes[2] option_strategy = OptionStrategies.bull_call_ladder(self._symbol, low_strike, middle_strike, high_strike, expiry) self.buy(option_strategy, 1)