Future Options
Handling Data
Introduction
LEAN passes the data you request to the OnData
on_data
method so you can make trading decisions. The default OnData
on_data
method accepts a Slice
object, but you can define additional OnData
on_data
methods that accept different data types. For example, if you define an OnData
on_data
method that accepts a TradeBar
argument, it only receives TradeBar
objects. The Slice
object that the OnData
on_data
method receives groups all the data together at a single moment in time. To access the Slice
outside of the OnData
on_data
method, use the CurrentSlice
current_slice
property of your algorithm.
All the data formats use DataDictionary
objects to group data by Symbol
and provide easy access to information. The plural of the type denotes the collection of objects. For instance, the TradeBars
DataDictionary
is made up of TradeBar
objects. To access individual data points in the dictionary, you can index the dictionary with the Futures Option contract ticker or Symbol
symbol
, but we recommend you use the Symbol
symbol
.
To view the resolutions that are available for Future Options data, see Resolutions.
Trades
TradeBar
objects are price bars that consolidate individual trades from the exchanges. They contain the open, high, low, close, and volume of trading activity over a period of time.
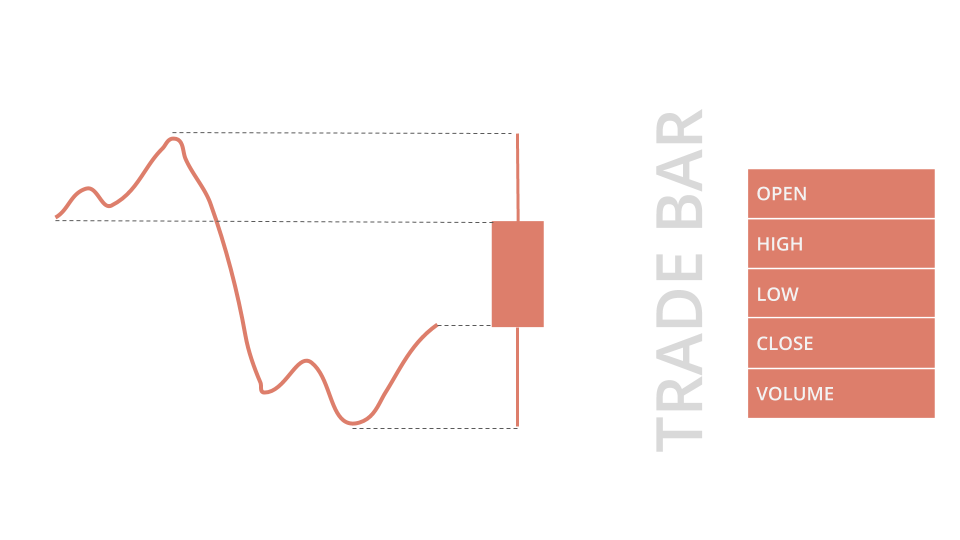
TradeBar
objects have the following properties:
To get the TradeBar
objects in the Slice
, index the Slice
or index the Bars
bars
property of the Slice
with the Option contract Symbol
symbol
. If the Option contract doesn't actively trade or you are in the same time step as when you added the Option contract subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your Option contract before you index the Slice
with the Option contract Symbol
symbol
.
public override void OnData(Slice slice) { if (slice.Bars.ContainsKey(_optionContractSymbol)) { var tradeBar = slice.Bars[_optionContractSymbol]; } }
def on_data(self, slice: Slice) -> None: trade_bar = slice.bars.get(self._option_contract_symbol) # None if not found
You can also iterate through the TradeBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the TradeBar
objects.
public override void OnData(Slice slice) { foreach (var kvp in slice.Bars) { var symbol = kvp.Key; var tradeBar = kvp.Value; var closePrice = tradeBar.Close; } }
def on_data(self, slice: Slice) -> None: for symbol, trade_bar in slice.bars.items(): close_price = trade_bar.close
Quotes
QuoteBar
objects are bars that consolidate NBBO quotes from the exchanges. They contain the open, high, low, and close prices of the bid and ask. The Open
open
, High
high
, Low
low
, and Close
close
properties of the QuoteBar
object are the mean of the respective bid and ask prices. If the bid or ask portion of the QuoteBar
has no data, the Open
open
, High
high
, Low
low
, and Close
close
properties of the QuoteBar
copy the values of either the Bid
bid
or Ask
ask
instead of taking their mean.
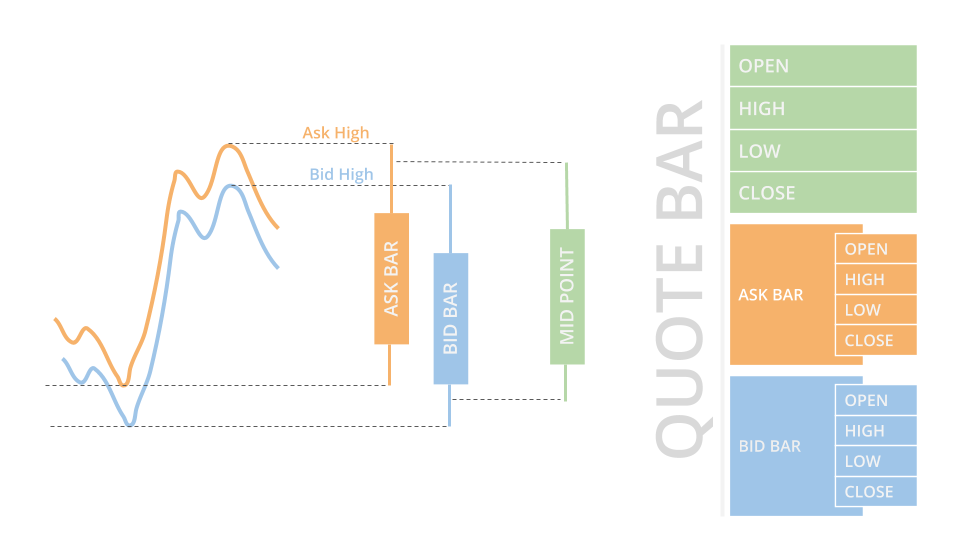
QuoteBar
objects have the following properties:
To get the QuoteBar
objects in the Slice
, index the QuoteBars
property of the Slice
with the Option contract Symbol
symbol
. If the Option contract doesn't actively get quotes or you are in the same time step as when you added the Option contract subscription, the Slice
may not contain data for your Symbol
symbol
. To avoid issues, check if the Slice
contains data for your Option contract before you index the Slice
with the Option contract Symbol
symbol
.
public override void OnData(Slice slice) { if (slice.QuoteBars.ContainsKey(_optionContractSymbol)) { var quoteBar = slice.QuoteBars[_optionContractSymbol]; } }
def on_data(self, slice: Slice) -> None: quote_bar = slice.quote_bars.get(self._option_contract_symbol) # None if not found
You can also iterate through the QuoteBars
dictionary. The keys of the dictionary are the Symbol
objects and the values are the QuoteBar
objects.
public override void OnData(Slice slice) { foreach (var kvp in slice.QuoteBars) { var symbol = kvp.Key; var quoteBar = kvp.Value; var askPrice = quoteBar.Ask.Close; } }
def on_data(self, slice: Slice) -> None: for symbol, quote_bar in slice.quote_bars.items(): ask_price = quote_bar.ask.close
QuoteBar
objects let LEAN incorporate spread costs into your simulated trade fills to make backtest results more realistic.
Futures Chains
FuturesChain
objects represent an entire chain of contracts for a single underlying Future. They have the following properties:
To get the FuturesChain
, index the FuturesChains
futures_chains
property of the Slice
with the continuous contract Symbol
.
public override void OnData(Slice slice) { if (slice.FuturesChains.TryGetValue(_futureContractSymbol.Canonical, out var chain)) { var contracts = chain.Contracts; } }
def on_data(self, slice: Slice) -> None: chain = slice.futures_chains.get(self._future_contract_symbol.canonical) if chain: contracts = chain.contracts
You can also loop through the FuturesChains
futures_chains
property to get each FuturesChain
.
public override void OnData(Slice slice) { foreach (var kvp in slice.FuturesChains) { var continuousContractSymbol = kvp.Key; var chain = kvp.Value; var contracts = chain.Contracts; } } public void OnData(FuturesChains futuresChains) { foreach (var kvp in futuresChains) { var continuousContractSymbol = kvp.Key; var chain = kvp.Value; var contracts = chain.Contracts; } }
def on_data(self, slice: Slice) -> None: for continuous_contract_symbol, chain in slice.futures_chains.items(): contracts = chain.contracts
Futures Contracts
FuturesContract
objects represent the data of a single Futures contract in the market. They have the following properties:
To get the Futures contracts in the Slice
, use the Contracts
contracts
property of the FuturesChain
.
public override void OnData(Slice slice) { if (slice.FuturesChains.TryGetValue(_futureContractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_futureContractSymbol, out var contract)) { var price = contract.LastPrice; } } } public void OnData(FuturesChains futuresChains) { if (futuresChains.TryGetValue(_futureContractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_futureContractSymbol, out var contract)) { var price = contract.LastPrice; } } }
def on_data(self, slice: Slice) -> None: chain = slice.FuturesChains.get(self._future_contract_symbol.Canonical) if chain: contract = chain.Contracts.get(self._future_contract_symbol) if contract: price = contract.LastPrice
Option Chains
OptionChain
objects represent an entire chain of Option contracts for a single underlying security. They have the following properties:
To get the OptionChain
, index the OptionChains
option_chains
property of the Slice
with the canonical Symbol
.
public override void OnData(Slice slice) { if (slice.OptionChains.TryGetValue(_optionContractSymbol.Canonical, out var chain)) { var contracts = chain.Contracts; } }
def on_data(self, slice: Slice) -> None: chain = slice.option_chains.get(self._option_contract_symbol.Canonical) if chain: contracts = chain.contracts
You can also loop through the OptionChains
option_chains
property to get each OptionChain
.
public override void OnData(Slice slice) { foreach (var kvp in slice.OptionChains) { var canonicalFOPSymbol = kvp.Key; var chain = kvp.Value; var contracts = chain.Contracts; } }
def on_data(self, slice: Slice) -> None: for canonical_fop_symbol, chain in slice.option_chains.items(): contracts = chain.contracts
Option Contracts
OptionContract
objects represent the data of a single Option contract in the market. They have the following properties:
To get the Option contracts in the Slice
, use the Contracts
contracts
property of the OptionChain
.
public override void OnData(Slice slice) { if (slice.OptionChains.TryGetValue(_optionContractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_optionContractSymbol, out var contract)) { var price = contract.Price; } } }
def on_data(self, slice: Slice) -> None: chain = slice.option_chains.get(self._option_contract_symbol.canonical) if chain: contract = chain.contracts.get(self._option_contract_symbol) if contract: price = contract.price
You can also iterate through the FuturesChains
futures_chains
first.
public override void OnData(Slice slice) { foreach (var kvp in slice.FuturesChains) { var continuousContractSymbol = kvp.Key; var futuresChain = kvp.Value; // Select a Future Contract and create its canonical FOP Symbol var futuresContract = futuresChain.First(); var canonicalFOPSymbol = QuantConnect.Symbol.CreateCanonicalOption(futuresContract.Symbol); if (slice.OptionChains.TryGetValue(canonicalFOPSymbol, out var optionChain)) { if (optionChain.Contracts.TryGetValue(_optionContractSymbol, out var optionContract)) { var price = optionContract.Price; } } } }
def on_data(self, slice: Slice) -> None: for continuous_future_symbol, futures_chain in slice.futures_chains.items(): # Select a Future Contract and create its canonical FOP Symbol futures_contract = [contract for contract in futures_chain][0] canonical_fop_symbol = Symbol.create_canonical_option(futures_contract.symbol) option_chain = slice.option_chains.get(canonical_fop_symbol) if option_chain: option_contract = option_chain.contracts.get(self._option_contract_symbol) if option_contract: price = option_contract.price
Greeks and Implied Volatility
To get the Greeks and implied volatility of an Option contract, use the Greeks
greeks
and implied_volatility
members.
public override void OnData(Slice slice) { if (slice.OptionChains.TryGetValue(_optionContractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_optionContractSymbol, out var contract)) { var delta = contract.Greeks.Delta; var iv = contract.ImpliedVolatility; } } }
def on_data(self, slice: Slice) -> None: chain = slice.option_chains.get(self._option_contract_symbol.canonical) if chain: contract = chain.contracts.get(self._option_contract_symbol) if contract: delta = contract.greeks.delta iv = contract.implied_volatility
LEAN only calculates Greeks and implied volatility when you request them because they are expensive operations. If you invoke the Greeks
greeks
property, the Greeks aren't calculated. However, if you invoke the Greeks.Delta
greeks.delta
, LEAN calculates the delta. To avoid unecessary computation in your algorithm, only request the Greeks and implied volatility when you need them. For more information about the Greeks and implied volatility, see Options Pricing.
Open Interest
Open interest is the number of outstanding contracts that haven't been settled. It provides a measure of investor interest and the market liquidity, so it's a popular metric to use for contract selection. Open interest is calculated once per day. To get the latest open interest value, use the OpenInterest
open_interest
property of the Option
or OptionContract
Optioncontract
.
public override void OnData(Slice slice) { if (slice.OptionChains.TryGetValue(_contractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_contractSymbol, out var contract)) { var openInterest = contract.OpenInterest; } } } public void OnData(OptionChains optionChains) { if (optionChains.TryGetValue(_contractSymbol.Canonical, out var chain)) { if (chain.Contracts.TryGetValue(_contractSymbol, out var contract)) { var openInterest = contract.OpenInterest; } } }
def on_data(self, slice: Slice) -> None: chain = slice.OptionChains.get(self._contract_symbol.canonical) if chain: contract = chain.contracts.get(self._contract_symbol) if contract: open_interest = contract.open_interest