Option Strategies
Long Box Spread
Introduction
A long Box Spread is the combination of a bull call spread and a bear put spread. It consist of buying an ITM call at strike A, selling an OTM put at strike A, buying an ITM put at strike B<A, and selling an OTM call at strike B, where all of the contracts have the same expiry date. This strategy serves as an delta-neutral arbitration from Option mispricing. Note that it only attains a true profit when the risk-free return is greater than the risk-free interest rate.
Implementation
Follow these steps to implement the long box spread strategy:
- In the
initialize
method, set the start date, set the end date, subscribe to the underlying Equity, and create an Option universe. - In the
on_data
method, select the expiry and strikes of the contracts in the strategy legs. - In the
on_data
method, select the contracts and place the order.
def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 30) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().box_spread(30, 5))
The box_spread
filter narrows the universe down to just the four contracts you need to form a box spread.
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select an expiry date and ITM & OTM strike prices expiry = max([x.expiry for x in chain]) contracts = [x for x in chain if x.expiry == expiry] lower_strike = min([x.strike for x in contracts]) higher_strike = max([x.strike for x in contracts])
Approach A: Call the OptionStrategies.box_spread
method with the details of each leg and then pass the result to the buy
method.
box_spread = OptionStrategies.box_spread(self._symbol, higher_strike, lower_strike, expiry) self.buy(box_spread, 1)
Approach B: Create a list of Leg
objects and then call the combo_market_order, combo_limit_order, or combo_leg_limit_order method.
# Select the call and put contracts itm_call = next(filter(lambda x: x.right == OptionRight.CALL and x.strike == lower_strike, contracts)) otm_call = next(filter(lambda x: x.right == OptionRight.CALL and x.strike == higher_strike, contracts)) itm_put = next(filter(lambda x: x.right == OptionRight.PUT and x.strike == higher_strike, contracts)) otm_put = next(filter(lambda x: x.right == OptionRight.PUT and x.strike == lower_strike, contracts)) legs = [ Leg.create(itm_call.symbol, 1), Leg.create(itm_put.symbol, 1), Leg.create(otm_call.symbol, -1), Leg.create(otm_put.symbol, -1), ] self.combo_market_order(legs, 1)
Strategy Payoff
This is a fixed payoff, delta-neutral strategy. The payoff is
CITMT=(ST−K−)+COTMT=(ST−K+)+PITMT=(K+−ST)+POTMT=(K−−ST)+PayoffT=(CITMT−CITMT0+PITMT−PITMT0−COTMT+COTMT0−POTMT+POTMT0)×m−fee=(K+−K−+COTMT0+POTMT0−CITMT0−PITMT0)×m−feeThe following chart shows the payoff at expiration:
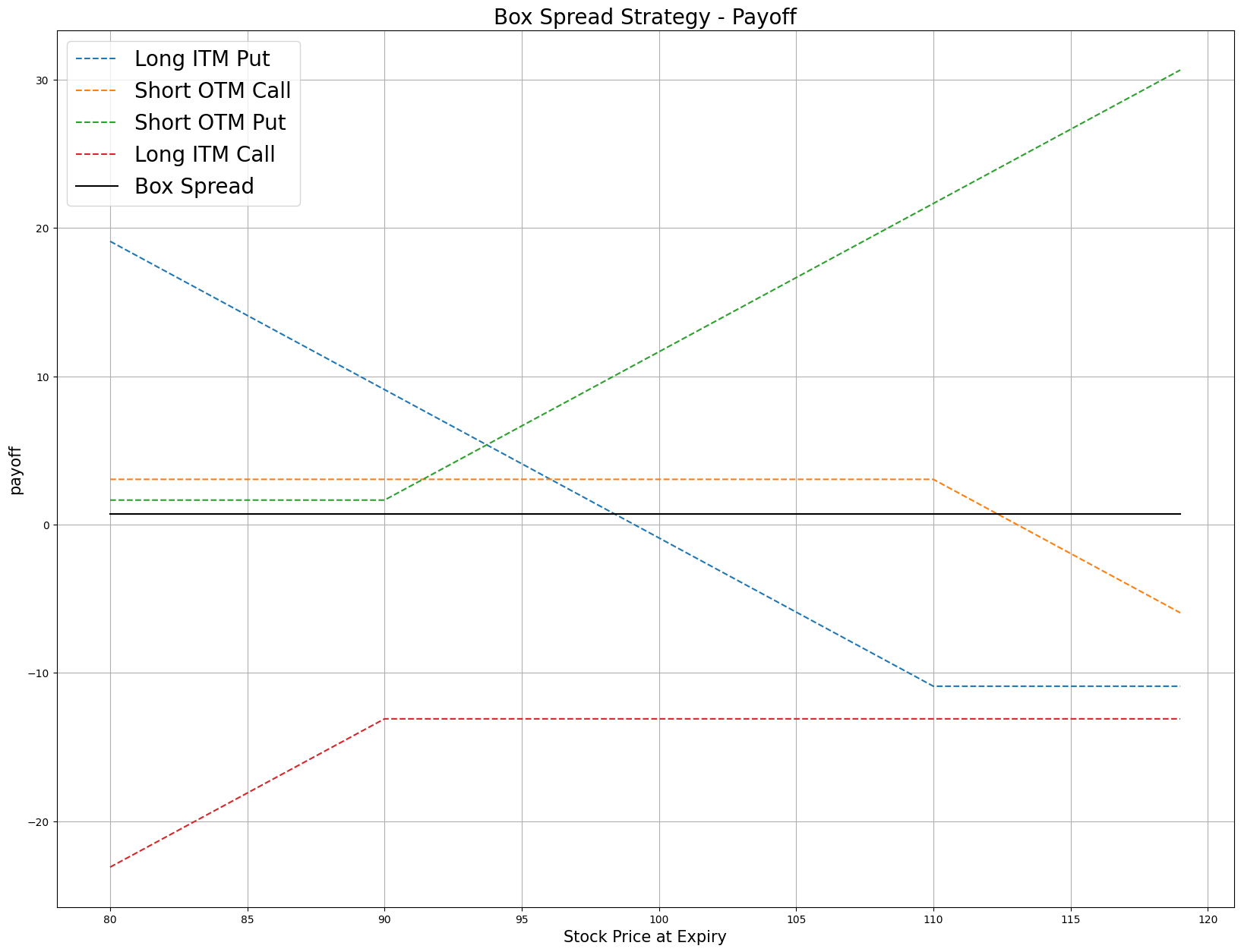
The payoff is only dependent on the strike price and the initial asset prices.
If the Option is American Option, there is a risk of early assignment on the contracts you sell.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
ITM Call | 27.30 | 810.00 |
ITM Put | 28.00 | 857.50 |
OTM Call | 1.05 | 857.50 |
OTM Put | 1.50 | 810.00 |
Underlying Equity at expiration | 843.25 | - |
Therefore, the payoff is
PayoffT=(K+−K−+COTM0+POTM0−CITM0−PITM0)×m−fee=(857.50−810.00+1.05+1.50−27.30−28.00)×100−1.00×4=−529.00So, the strategy loses $529.
The following algorithm implements a long box spread Option strategy:
class BoxSpreadStrategy(QCAlgorithm): def initialize(self) -> None: self.set_start_date(2017, 4, 1) self.set_end_date(2017, 4, 30) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.Symbol option.set_filter(lambda universe: universe.include_weeklys().box_spread(30, 5)) def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Select an expiry date expiry = max([x.expiry for x in chain]) # Select the strike prices of the contracts contracts = [x for x in chain if x.expiry == expiry] higher_strike = max([x.strike for x in contracts]) lower_strike = min([x.strike for x in contracts]) box_spread = OptionStrategies.box_spread(self._symbol, higher_strike, lower_strike, expiry) self.buy(box_spread, 1) def on_end_of_day(self, symbol: Symbol) -> None: if symbol == self._symbol.underlying: self.Log(f"{self.time}::{symbol}::{self.securities[symbol].price}")