Option Strategies
Protective Put
Introduction
A Protective Put consists of buying a long position in a stock and a long position in put Options for the same amount of stock. Protective puts aim to hedge the long position of a stock with a long ATM or slightly OTM put Option. At any time for American Options or at expiration for European Options, if the stock moves above the strike price, the Option contract becomes worthless but the long stock position acquires an unrealized gain. If the underlying price moves below the strike, you can exercise the Options contract, which sells your underlying position at the put Option strike price.
Implementation
Follow these steps to implement the protective put strategy:
- In the
initialize
method, set the start date, end date, starting cash, and Options universe. - In the
on_data
method, select the Option contract. - In the
on_data
method, place the orders.
def initialize(self) -> None: self.set_start_date(2014, 1, 1) self.set_end_date(2014, 3, 1) self.set_cash(100000) self.universe_settings.asynchronous = True option = self.add_option("IBM") self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().naked_put(30, 0))
The naked_put
filter narrows the universe down to just the one contract you need to form a protective put.
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return chain = slice.option_chains.get(self._symbol) if not chain: return # Find ATM put with the farthest expiry expiry = max([x.expiry for x in chain]) put_contracts = sorted([x for x in chain if x.right == OptionRight.PUT and x.expiry == expiry], key=lambda x: abs(chain.underlying.price - x.strike)) if not put_contracts: return atm_put = put_contracts[0]
Approach A: Call the OptionStrategies.protective_put
method with the details of each leg and then pass the result to the buy
method.
protective_put = OptionStrategies.protective_put(self._symbol, atm_put.strike, expiry) self.buy(protective_put, 1)
Approach B: Create a list of Leg
objects and then call the combo_market_order, combo_limit_order, or combo_leg_limit_order method.
legs = [ Leg.create(atm_put.symbol, 1), Leg.create(chain.underlying.symbol, chain.underlying.symbol_properties.contract_multiplier) ] self.combo_market_order(legs, 1)
Strategy Payoff
The payoff of the strategy is
PKT=(K−ST)+PT=(ST−S0+PKT−PK0)×m−fee wherePKT=Put value at time TST=Underlying asset price at time TK=Put strike pricePT=Payout total at time TS0=Underlying asset price when the trade openedPK0=Put price when the trade opened (credit received)m=Contract multiplierT=Time of expirationThe following chart shows the payoff at expiration:
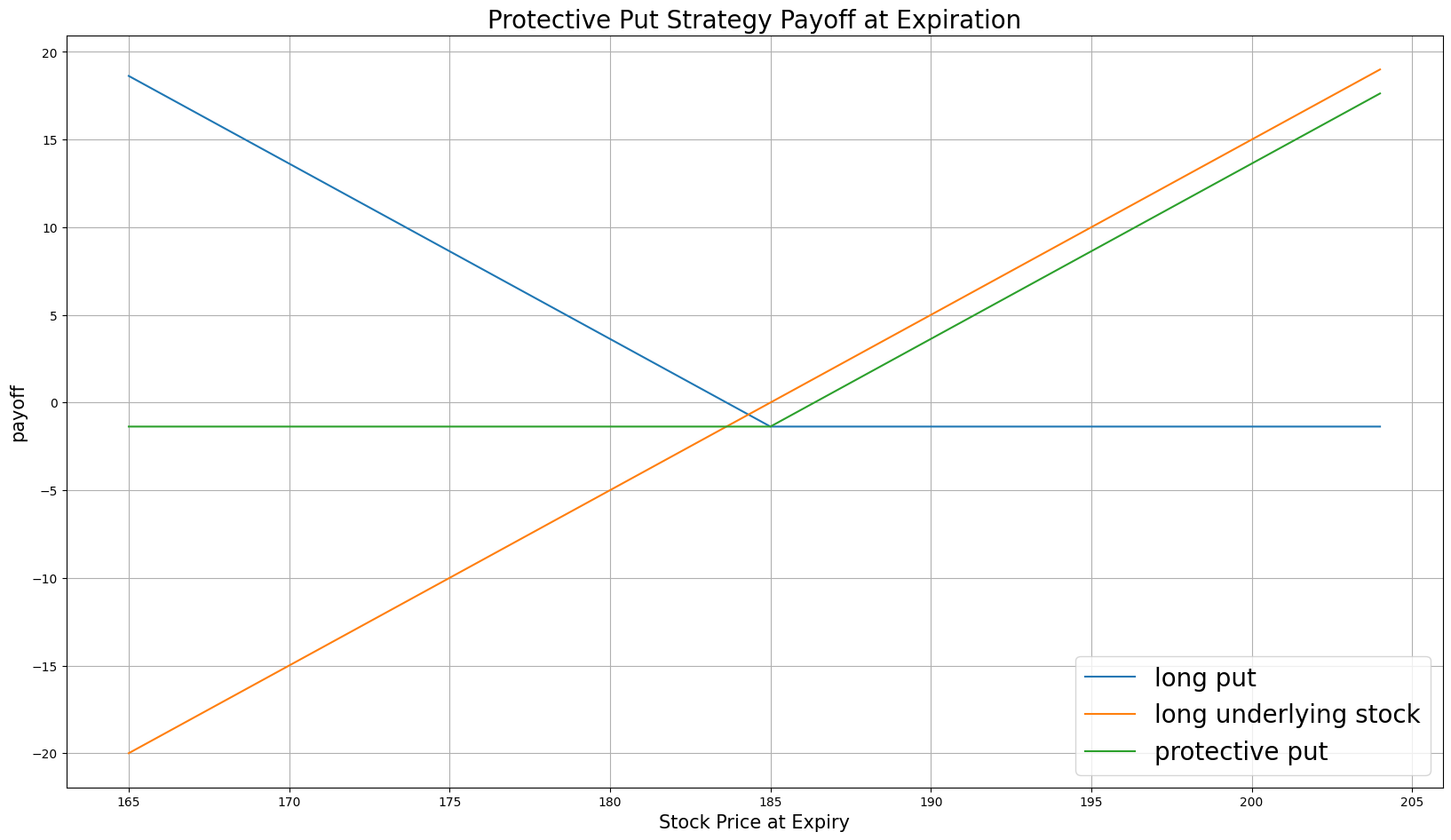
The maximum profit is ST−S0−PK0, which occurs when the underlying price is above the S0+PK0.
The maximum loss is PK0, which occurs when the underlying price drops.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
Put | 1.53 | 185.00 |
Underlying Equity at start of the trade | 187.07 | - |
Underlying Equity at expiration | 190.01 | - |
Therefore, the payoff is
PKT=(K−ST)+=(185−190.1)+=0PT=(ST−S0+PKT−PK0)×m−fee=(190.01−187.07+0−1.53)×m−fee=1.41×100−2=139So, the strategy gains $139.
The following algorithm implements a protective put Option strategy:
class ProtectivePutAlgorithm(QCAlgorithm): def Initialize(self) -> None: self.SetStartDate(2014, 1, 1) self.SetEndDate(2014, 3, 1) self.SetCash(100000) option = self.AddOption("IBM") self.symbol = option.Symbol option.set_filter(lambda universe: universe.include_weeklys().naked_put(30, 0)) self.put = None # use the underlying equity as the benchmark self.SetBenchmark(self.symbol.Underlying) def OnData(self, slice: Slice) -> None: if self.put and self.Portfolio[self.put].Invested: return chain = slice.OptionChains.get(self.symbol) if not chain: return # Find ATM put with the farthest expiry expiry = max([x.Expiry for x in chain]) put_contracts = sorted([x for x in chain if x.Right == OptionRight.Put and x.Expiry == expiry], key=lambda x: abs(chain.Underlying.Price - x.Strike)) if not put_contracts: return atm_put = put_contracts[0] protective_put = OptionStrategies.ProtectivePut(self.symbol, atm_put.Strike, expiry) self.Buy(protective_put, 1) self.put = atm_put.Symbol