Option Strategies
Bull Call Spread
Introduction
Bull call spread, also known as long call spread, consists of buying an ITM call and selling an OTM call. Both calls have the same underlying Equity and the same expiration date. The OTM call serves as a hedge for the ITM call. The bull call spread profits from a rise in underlying asset price.
Implementation
Follow these steps to implement the bull call spread strategy:
- In the
Initialize
initialize
method, set the start date, end date, cash, and Option universe. - In the
OnData
on_data
method, select the expiration and strikes of the contracts in the strategy legs. - In the
OnData
on_data
method, call theOptionStrategies.BullCallSpread
method and then submit the order.
private Symbol _symbol; public override void Initialize() { SetStartDate(2017, 2, 1); SetEndDate(2017, 3, 5); SetCash(500000); UniverseSettings.Asynchronous = true; var option = AddOption("GOOG", Resolution.Minute); _symbol = option.Symbol; option.SetFilter(universe => universe.IncludeWeeklys().Strikes(-15, 15).Expiration(0, 31)); }
def initialize(self) -> None: self.set_start_date(2017, 2, 1) self.set_end_date(2017, 3, 5) self.set_cash(500000) self.universe_settings.asynchronous = True option = self.add_option("GOOG", Resolution.MINUTE) self._symbol = option.symbol option.set_filter(lambda universe: universe.include_weeklys().strikes(-15, 15).expiration(0, 31))
public override void OnData(Slice slice) { if (Portfolio.Invested) return; // Get the OptionChain var chain = slice.OptionChains.get(_symbol, null); if (chain.Count() == 0) return; // Get the furthest expiration date of the contracts var expiry = chain.OrderByDescending(x => x.Expiry).First().Expiry; // Select the call Option contracts with the furthest expiry var calls = chain.Where(x => x.Expiry == expiry && x.Right == OptionRight.Call); if (calls.Count() == 0) return; // Select the ITM and OTM contract strikes from the remaining contracts var putStrikes = calls.Select(x => x.Strike).OrderBy(x => x); var itmStrike = putStrikes.First(); var otmStrike = putStrikes.Last();
def on_data(self, slice: Slice) -> None: if self.portfolio.invested: return # Get the OptionChain chain = slice.option_chains.get(self._symbol, None) if not chain: return # Get the furthest expiration date of the contracts expiry = sorted(chain, key = lambda x: x.expiry, reverse=True)[0].expiry # Select the call Option contracts with the furthest expiry calls = [i for i in chain if i.expiry == expiry and i.right == OptionRight.CALL] if len(calls) == 0: return # Select the ITM and OTM contract strike prices from the remaining contracts call_strikes = sorted([x.strike for x in calls]) itm_strike = call_strikes[0] otm_strike = call_strikes[-1]
var optionStrategy = OptionStrategies.BullCallSpread(_symbol, itmStrike, otmStrike, expiry); Buy(optionStrategy, 1);
option_strategy = OptionStrategies.bull_call_spread(self._symbol, itm_strike, otm_strike, expiry) self.buy(option_strategy, 1)
Option strategies synchronously execute by default. To asynchronously execute Option strategies, set the asynchronous
argument to False
false
. You can also provide a tag and order properties to the
Buy
method.
Buy(optionStrategy, quantity, asynchronous, tag, orderProperties);
self.Buy(option_strategy, quantity, asynchronous, tag, order_properties)
Strategy Payoff
The bull call spread is a limited-reward-limited-risk strategy. The payoff is
$$ \begin{array}{rcll} C^{OTM}_T & = & (S_T - K^{OTM})^{+}\\ C^{ITM}_T & = & (S_T - K^{ITM})^{+}\\ P_T & = & (C^{ITM}_T - C^{OTM}_T + C^{OTM}_0 - C^{ITM}_0)\times m - fee\\ \end{array} $$ $$ \begin{array}{rcll} \textrm{where} & C^{OTM}_T & = & \textrm{OTM call value at time T}\\ & C^{ITM}_T & = & \textrm{ITM call value at time T}\\ & S_T & = & \textrm{Underlying asset price at time T}\\ & K^{OTM} & = & \textrm{OTM call strike price}\\ & K^{ITM} & = & \textrm{ITM call strike price}\\ & P_T & = & \textrm{Payout total at time T}\\ & C^{ITM}_0 & = & \textrm{ITM call value at position opening (debit paid)}\\ & C^{OTM}_0 & = & \textrm{OTM call value at position opening (credit received)}\\ & m & = & \textrm{Contract multiplier}\\ & T & = & \textrm{Time of expiration} \end{array} $$The following chart shows the payoff at expiration:
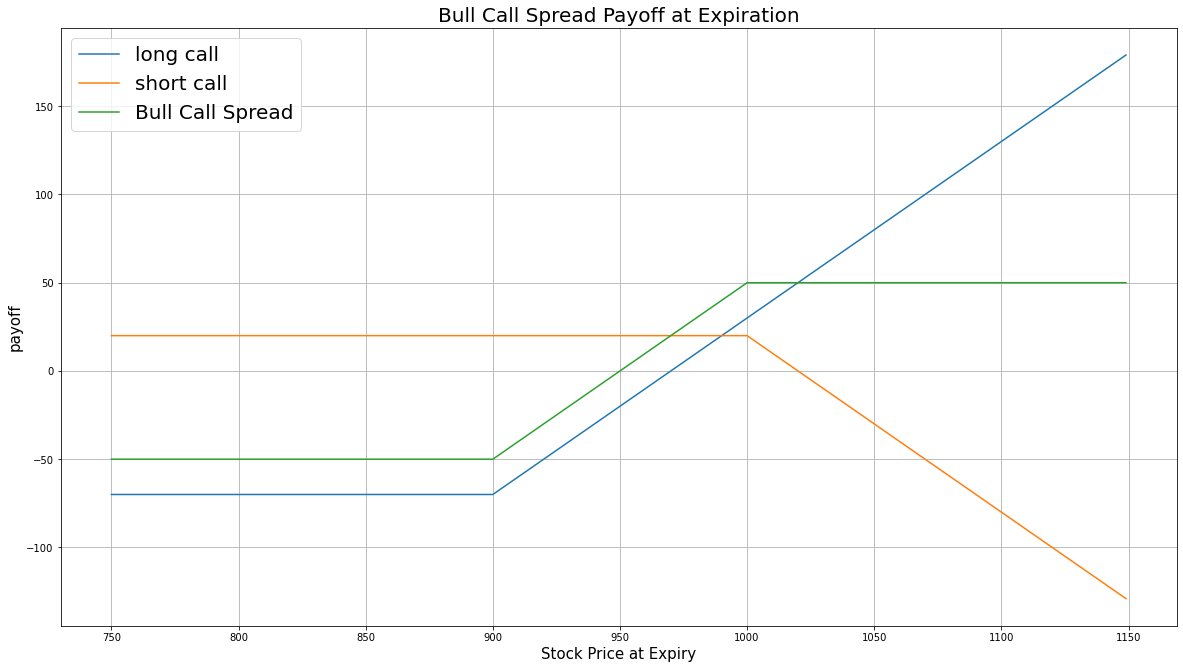
The maximum profit is $K^{OTM} - K^{ITM} + C^{OTM}_0 - C^{ITM}_0$. If the underlying price increases to exceed both strikes at expiration, both calls are worth $(S_T - K)$ at expiration.
The maximum loss is the net debit you paid to open the position, $C^{OTM}_0 - C^{ITM}_0$.
If the Option is American Option, there is a risk of early assignment on the sold contract.
Example
The following table shows the price details of the assets in the algorithm:
Asset | Price ($) | Strike ($) |
---|---|---|
OTM call | 3.00 | 835.00 |
ITM call | 41.00 | 767.50 |
Underlying Equity at expiration | 829.08 | - |
Therefore, the payoff is
$$ \begin{array}{rcll} C^{OTM}_T & = & (S_T - K^{OTM})^{+}\\ & = & (829.08-835.00)^{+}\\ & = & 0\\ C^{ITM}_T & = & (S_T - K^{ITM})^{+}\\ & = & (829.08-767.50)^{+}\\ & = & 61.58\\ P_T & = & (C^{ITM}_T - C^{OTM}_T + C^{OTM}_0 - C^{ITM}_0)\times m - fee\\ & = & (61.58-0+3.00-41.00)\times100-1.00\times2\\ & = & 2356\\ \end{array} $$So, the strategy profits $2,356.
The following algorithm implements a bull call spread strategy: