Contents
Algorithm Framework
Overview
Introduction
Since QuantConnect was formed in 2013, we've seen more than 2 million algorithms created by the community. Although they all seek to achieve different results, they can all be abstracted into the same core features. The Algorithm Framework is an attempt by QuantConnect to provide this well-defined structure to the community, encouraging good design and making your work more reusable.
These core "modules" – Universe Selection, Alpha Creation, Portfolio Construction, Execution and Risk Management – form the basis of all investment strategies.
- 1. Universe Selection - Select your assets.
- 2. Alpha Creation - Generate trading signals.
- 3. Portfolio Construction - Determine position size targets.
- 4. Execution - Place trades to reach your position sizes.
- 5. Risk Management - Manage the market risks.
In the following sections, we'll go into each of these modules in more detail.
The Algorithm Wizard
QuantConnect's algorithm wizard offers a growing number of premade framework modules. When you select a module in the wizard, you can see the full implementation in the second tab. Here are two examples of how one line of code can call for a premade universe class.
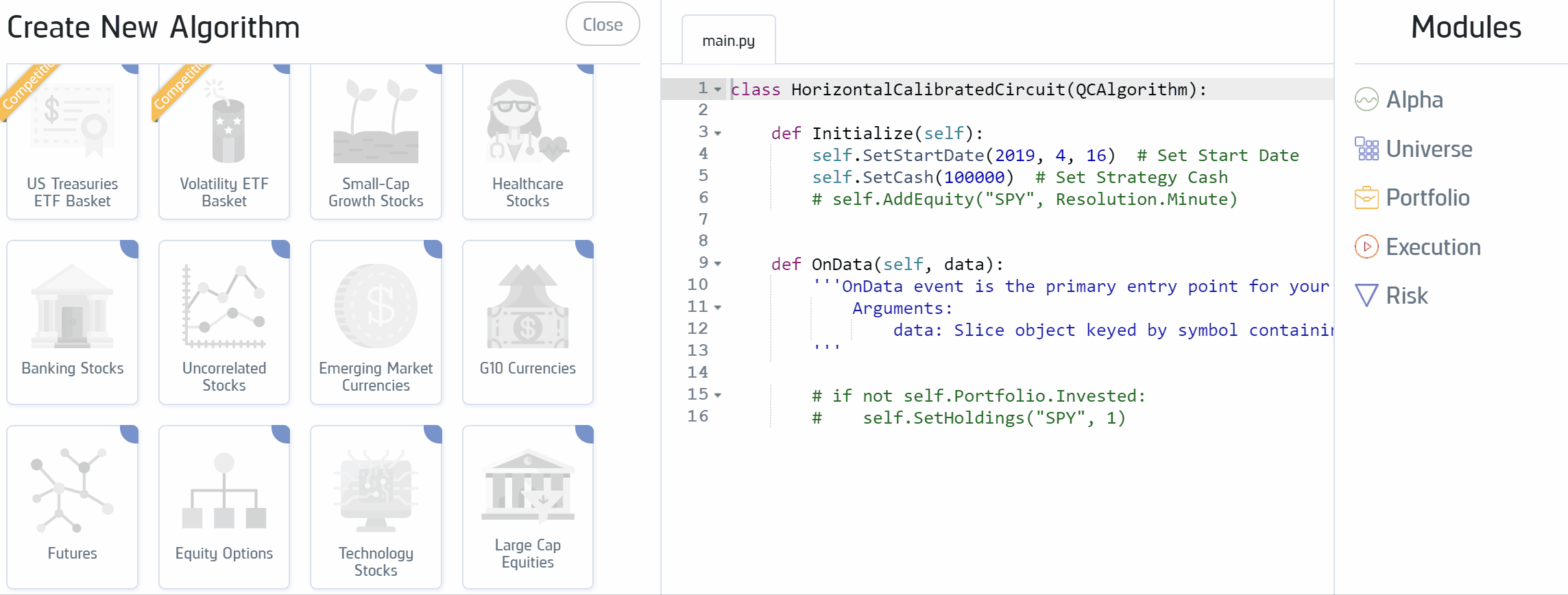
Example: A Universe of Technology Stocks
The Technology Universe Selection Model selects the most liquid stocks in the Nasdaq Stock Exchange. Through a combination of coarse and fine filtering on MorningStar data, it sorts all available symbols on dollar-volume and returns a sorted list of 1000 most liquid symbols.
def Initialize(self): # Pass in a universe class of technology stocks self.SetUniverseSelection(TechnologyUniverseModule())
Example: A Universe of Banking Stocks
The Banking Stock Universe Selection Model selects the most liquid stocks in the Morningstar Bank Industry. This comprises all public US Equities classified as Banks and provides an industry-specific Universe for banking stocks.
def Initialize(self): # Pass in a universe class of banking stocks self.SetUniverseSelection(BankingIndustryStocks())
Important Terminology
Term | Definition |
---|---|
Universe Selection Model | Framework component for selecting assets for your algorithm. |
Alpha Creation Model | Framework component that generates trade signals on the assets in your universe for your algorithm. |
Insight | A trade signal generated by an Alpha Model. An Insight indicates the Direction, Magnitude, Confidence, and Period of your signal. |
Portfolio Construction Model | Framework component that constructs a target portfolio from Insight signals created by the Alpha Model. |
Portfolio Target | A target quantity of holdings (e.g. shares, contracts) the algorithm desires to reach. This target is created by the Portfolio Construction Model. |
Execution Model | Framework component responsible for fulfilling the Portfolio Targets set by the Portfolio Construction Model. Execute trades to reach the desired portfolio make up. |
Risk Model | Framework component that manages ongoing market and portfolio risk, ensuring the algorithm remains in target parameters. |
Framework Advantages
The Algorithm Framework is now the recommended way to design strategies in QuantConnect. Following this well-defined structure gives many advantages over conventional design:
Pluggable Algorithm Modules
By using the algorithm framework, your code will be able to instantly utilize all modules built within the framework. The modules clip together in well-defined ways, which enable them to be shared and swapped interchangeably.
Focus On Your Strengths
By writing code in modules, you can focus on your strengths. If you are great at building universes, you can build universe modules. If you have risk-management experience, you could write reusable risk monitoring technology.
Reduce Development By Using Community Modules
Easily share modules you've made between algorithms or pull in ones produced by the community. The strict separation of duties makes the Algorithm Framework perfect for reusing code between strategies.
System Architecture
The Algorithm Framework is built into the QCAlgorithm class giving your strategy access to all the normal methods you use for algorithm development. You should be able to copy and paste your algorithms across without any changes. No separate class is required.
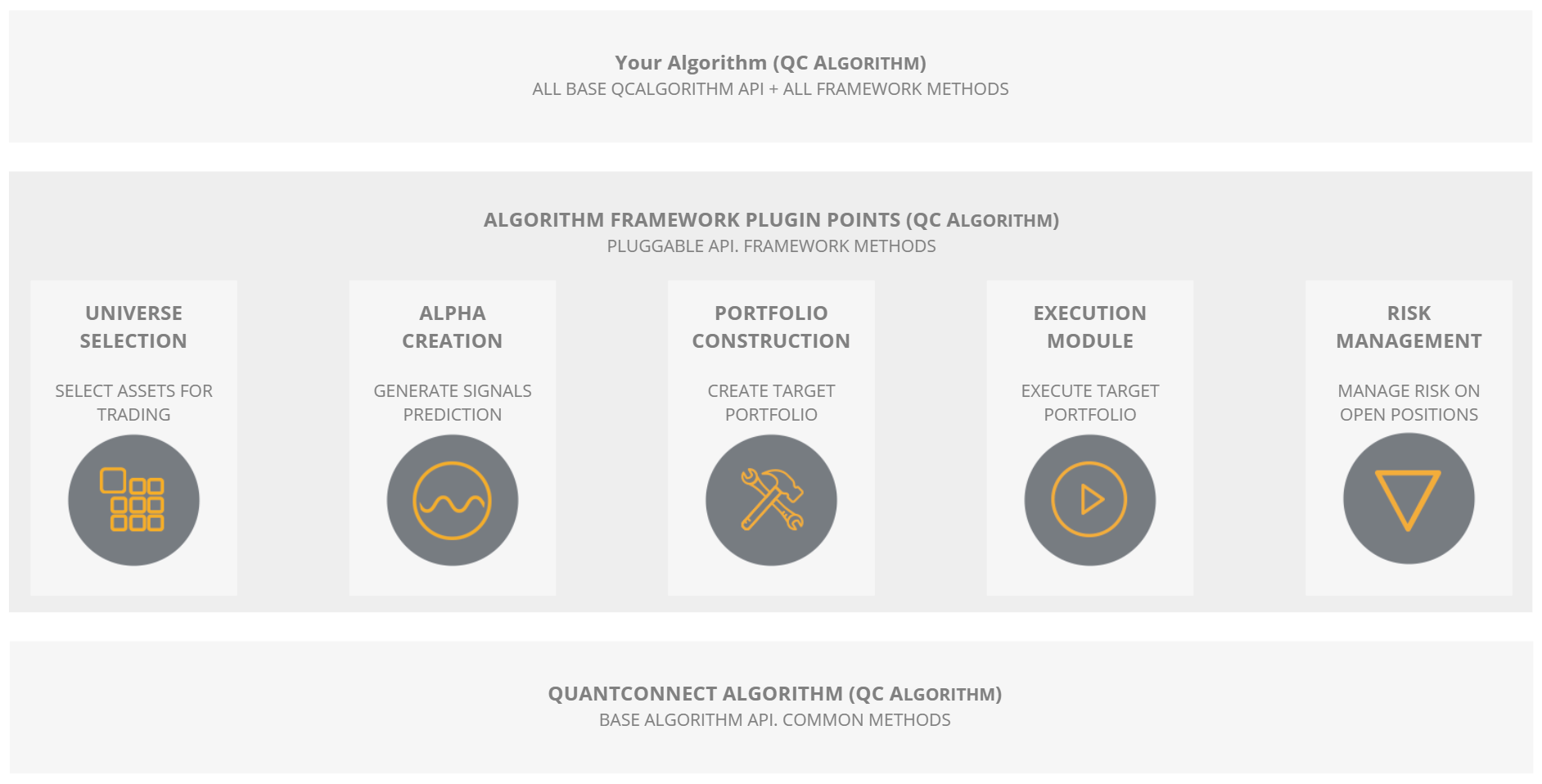
The framework data output of each module flows into the next predictably. Assets selected by the Universe Selection Model are fed into your Alpha Model to generate trade signals. The trade signals (Insights) are converted into Portfolio Targets by the Portfolio Construction Model. The Portfolio Targets hold a target share quantity we'd like the algorithm to hold. To execute these targets efficiently, the Execution module fills the targets over time. Finally, the Risk Management Model ensures our targets are still within safe risk parameters and adjusts the portfolio targets if required.
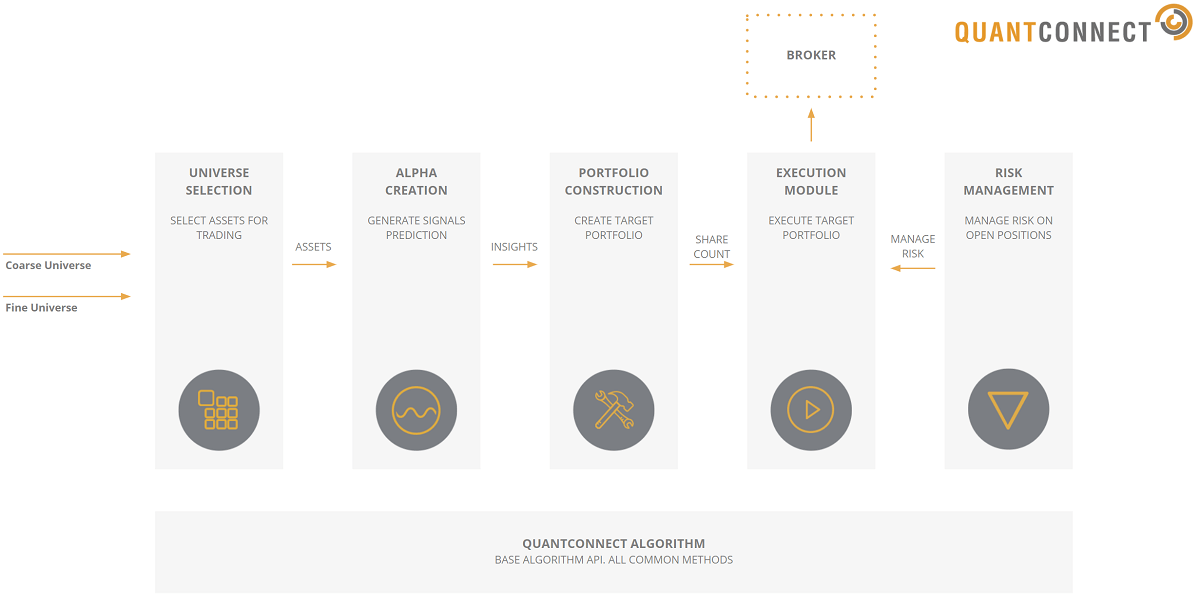
public class MyFrameworkAlgorithm : QCAlgorithm { public void Initialize() { // Setup Requested Modules } }
class MyFrameworkAlgorithm(QCAlgorithm): def Initialize(self): # Setup Requested Modules
For simple strategies, this may seem like overkill to abstract out your algorithm concepts; however, even simple strategies can benefit from reusing the ecosystem of modules available in QuantConnect. Imagine pairing your EMA-cross with a better execution system, or simply plugging in an open-source trailing stop risk management model.