Contents
Algorithm Framework
Execution
Execution
Introduction
The Execution Model is primarily concerned with efficiently executing trades. It seeks to find the optimal price to fill orders and manages the orders. It receives a PortfolioTarget array from the Portfolio Construction Model and uses it to place trades in the market, seeking to reach the units indicated in the Portfolio Target. To set your execution model, you should use the
SetExecution( IExecutionModel )
self.SetExecution( IExecutionModel )
method.
This should be done from your algorithm Initialize()
def Initialize()
method.
When the targets arrive at the Execution Model, they have already been risk-adjusted by the Risk Management Model. Like all models, the Execution Model only receives updates to the portfolio target share counts. You will not receive all the targets at once.
// Set your execution model in the Initialize() method SetExecution( new ImmediateExecutionModel() );
# Set your execution model in the def Initialize() method self.SetExecution( ImmediateExecutionModel() )
Execution Model Structure
Execution models have one required method to implement Execute(algorithm, targets). This is responsible for reaching the target portfolios as efficiently as possible. The PortfolioTarget objects are created by the Portfolio Construction Model and then adjusted by the Risk Management Module. The final risk-adjusted portfolio targets are delivered to your execution model for fulfillment.
// Basic Execution Model Scaffolding Structure Example class MyExecutionModel : ExecutionModel { // Fill the supplied portfolio targets efficiently. public override void Execute(QCAlgorithmFramework algorithm, IPortfolioTarget[] targets) { // NOP } // Optional: Securities changes event for handling new securities. public override void OnSecuritiesChanged(QCAlgorithmFramework algorithm, SecurityChanges changes) { } }
from clr import AddReference AddReference("QuantConnect.Algorithm.Framework") from QuantConnect.Algorithm.Framework.Execution import ExecutionModel # Execution Model scaffolding structure example class MyExecutionModel(ExecutionModel): # Fill the supplied portfolio targets efficiently def Execute(self, algorithm, targets): pass # Optional: Securities changes event for handling new securities. def OnSecuritiesChanged(self, algorithm, changes): pass
The PortfolioTarget class has the following properties available for use by the Execution Model. They can be accessed with their public properties target.Quantity
.
// Final target quantity for execution class PortfolioTarget : IPortfolioTarget { // Asset to be traded. Symbol Symbol; // Number of units to hold. decimal Quantity; }
# Final target quantity for execution class PortfolioTarget: self.Symbol # Asset to be traded (Symbol object) self.Quantity # Number of units to hold (Decimal)
Immediate Execution Model
The Immediate Execution Model uses market orders to immediately fill algorithm portfolio targets. It is the simplest Execution Model similar to simply placing Market Orders inline with your algorithm logic.
You can use this pre-made Execution Model by setting it in the Initialize method:
SetExecution( new ImmediateExecutionModel() );
self.SetExecution( ImmediateExecutionModel() )
It is implemented as demonstrated in the code snippet below:
// Issue market orders for the difference between holdings & targeted quantity public override void Execute(QCAlgorithmFramework algorithm, IPortfolioTarget[] targets) { foreach (var target in targets) { var existing = algorithm.Securities[target.Symbol].Holdings.Quantity + algorithm.Transactions.GetOpenOrders(target.Symbol).Sum(o => o.Quantity); var quantity = target.Quantity - existing; if (quantity != 0) { algorithm.MarketOrder(target.Symbol, quantity); } } }
# Issue market orders for the difference between holdings & targeted quantity def Execute(self, algorithm, targets): for target in targets: open_quantity = sum([x.Quantity for x in algorithm.Transactions.GetOpenOrders(target.Symbol)]) existing = algorithm.Securities[target.Symbol].Holdings.Quantity + open_quantity quantity = target.Quantity - existing if quantity != 0: algorithm.MarketOrder(target.Symbol, quantity)
You can view the complete C# implementation of this model in GitHub.You can view the complete Python implementation of this model in GitHub.
VWAP Execution Model
The VWAP Execution Model seeks for the average fill price of your position to match or be better than the volume weighted average price for the trading day. This is a best-effort algorithm, and no guarantee can be made that it will reach the VWAP.
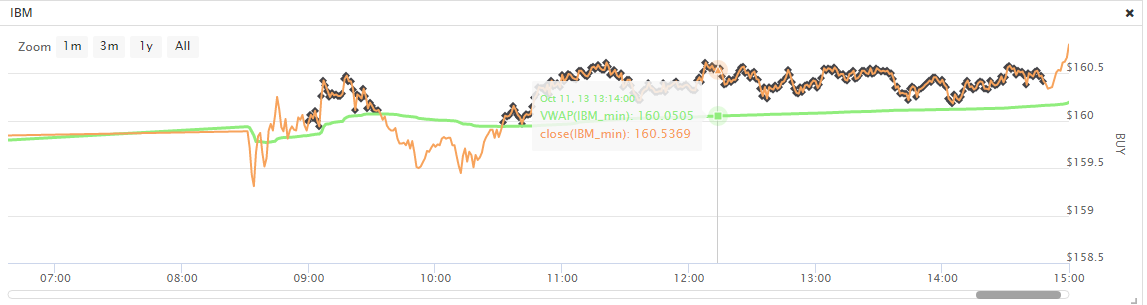
To use the pre-made Execution Model in your algorithm, you should set it in Initialize():
SetExecution( new VolumeWeightedAveragePriceExecutionModel() );
self.SetExecution( VolumeWeightedAveragePriceExecutionModel() )
You can view the complete C# implementation of this model in GitHub.You can view the complete Python implementation of this model in GitHub.
Standard Deviation Execution Model
The Standard Deviation Execution Model seeks to fill orders when the price is more than 2 standard deviations lower than the normal stock price for a given period. The intent is to find dips in the market to place trades. Unfortunately, in strongly trending markets, this can result in delayed trade placement as it might be a while before the next price dip.
To use the pre-made Execution Model in your algorithm, you should set it in Initialize():
SetExecution( new StandardDeviationExecutionModel() );
self.SetExecution( StandardDeviationExecutionModel() )
This model has the following optional parameters:
StandardDeviationExecutionModel( deviations = 2, period = 60, resolution=Resolution.Minute )
deviations
- Minimum deviations from mean before trading.period
- Period of the standard deviation indicator.resolution
- Resolution of the deviation indicators.
You can view the complete C# implementation of this model in GitHub.You can view the complete Python implementation of this model in GitHub.