Hi!
I'm new to QuantConnect and new to Python.
How can I create a simple backtesting for let's say one stock (e.g. 'BAC') where investment is made on Sept. 27 2017 and sell is made on Dec. 21 2017 ? Investment: 10.000 USD.
I've reviewed the documentation, but I have not found examples for this case. Code is not straightforward, so I would be happy for some help.
Jing Wu
You could use schedule event. For example
# schedule an event to fire at a specific date/time self.Schedule.On(self.DateRules.On(2017, 9, 27), self.TimeRules.At(10, 0), Action(self.buy)) self.Schedule.On(self.DateRules.On(2017, 12, 21), self.TimeRules.At(10, 0), Action(self.sell)) def buy(self): # place buy order def sell(self): # place sell order
Forum TD
an error occours at the 4.th line
"def buy(self):"
Build Error: File: main.py Line:25 Column:0 - IndentationError: expected an indented block
Quant Trader
can you share your algo? than we can have a better look. Did you walk through the tutorials with the sample algo? You can build on it. Takes a couple of days before you will feel comfortable.
Forum TD
Yes, I went through the tutorial, but tht sytax is compliated and they do not provide the why's. But this is another story.
Here it is my code: I took the predefined Basic Template and inserted only the four lines from @Jing Wu. And there is the build error:
Could not find a part of the path
import numpy as np ### <summary> ### Basic template algorithm simply initializes the date range and cash. This is a skeleton ### framework you can use for designing an algorithm. ### </summary> class BasicTemplateAlgorithm(QCAlgorithm): '''Basic template algorithm simply initializes the date range and cash''' def Initialize(self): '''Initialise the data and resolution required, as well as the cash and start-end dates for your algorithm. All algorithms must initialized.''' self.SetStartDate(2017,1, 1) #Set Start Date self.SetEndDate(2017,12,31) #Set End Date self.SetCash(100000) #Set Strategy Cash # Set Benchmark SPY self.SetBenchmark("SPY") # Find more symbols here: http://quantconnect.com/data self.AddEquity("BAC", Resolution.Daily) # Hmmmm.... what's this? What's going on here? self.Debug("numpy test >>> print numpy.pi: " + str(np.pi)) def OnData(self, data): '''OnData event is the primary entry point for your algorithm. Each new data point will be pumped in here. Arguments: data: Slice object keyed by symbol containing the stock data ''' if not self.Portfolio.Invested: self.SetHoldings("SPY", 1) self.Schedule.On(self.DateRules.On(2017, 9, 27), self.TimeRules.At(10, 0), Action(self.buy)) self.Schedule.On(self.DateRules.On(2017, 12, 21), self.TimeRules.At(10, 0), Action(self.sell)) # This line produces an error: Could not find a part of the path '/QuantConnect.Compiler/bin/.../bin/cache/__pycache__'. def buy(self): # place buy order def sell(self): # place sell order
Jing Wu
<1> self.Debug() statement could print the information in the console
<2> if you are going to trade "SPY" you need to subscribe the "SPY" data with self.AddEquity("SPY")
<3> self.Schedule.On() function should be in Initialize(self) method. You could customize your own buy and sell function to place orders, for example
def OnData(self, data):
# do nothing
pass
def buy(self):
# place buy order
self.SetHoldings("SPY", 1)
def sell(self):
# place sell order
self.SetHoldings("SPY", -1)
Forum TD
This is exactly what I was looking for. Thank you very much.
Just one question: When I add a second buy and second sell, there is no error message, but the results show that the sell on Nov. 9th and the Buy on Nov. 28th won't be recognized in backtesting.
# Find more symbols here: http://quantconnect.com/data self.AddEquity("BAC") self.Schedule.On(self.DateRules.On(2017, 9, 27), self.TimeRules.At(10, 0), Action(self.buy)) self.Schedule.On(self.DateRules.On(2017, 11, 9), self.TimeRules.At(10, 0), Action(self.sell)) self.Schedule.On(self.DateRules.On(2017, 11, 28), self.TimeRules.At(10, 0), Action(self.buy)) self.Schedule.On(self.DateRules.On(2018, 2, 5), self.TimeRules.At(10, 0), Action(self.sell))
Jing Wu
I got four trades with your schedule event functions, not sure why you have such problem. By the way, you should add equity "SPY" in initialize().
Forum TD
See my code:
import numpy as np ### <summary> ### Basic template algorithm simply initializes the date range and cash. This is a skeleton ### framework you can use for designing an algorithm. ### </summary> class BasicTemplateAlgorithm(QCAlgorithm): '''Basic template algorithm simply initializes the date range and cash''' def Initialize(self): '''Initialise the data and resolution required, as well as the cash and start-end dates for your algorithm. All algorithms must initialized.''' self.SetStartDate(2017,1, 1) #Set Start Date self.SetEndDate(2017,12,31) #Set End Date self.SetCash(100000) #Set Strategy Cash # Set Benchmark SPY self.SetBenchmark("SPY") # Find more symbols here: http://quantconnect.com/data self.AddEquity("BAC") self.Schedule.On(self.DateRules.On(2017, 2, 1), self.TimeRules.At(10, 0), Action(self.buy)) self.Schedule.On(self.DateRules.On(2017, 3, 31), self.TimeRules.At(10, 0), Action(self.sell)) self.Schedule.On(self.DateRules.On(2017, 11, 1), self.TimeRules.At(10, 0), Action(self.buy)) self.Schedule.On(self.DateRules.On(2017, 12, 31), self.TimeRules.At(10, 0), Action(self.sell)) def OnData(self, data): pass def buy(self): # place buy order self.SetHoldings("BAC", 1) def sell(self): # place sell order self.SetHoldings("BAC", -1)
I've defined four trades. But the result is wrong:
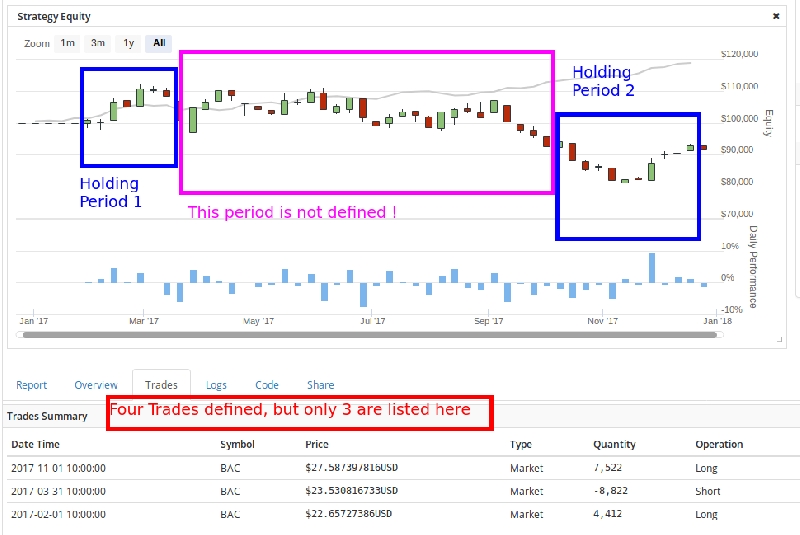
Jared Broad
- Please assume with 50,000 users and 70+ open source contributors that its your lack of understanding vs saying the "result is wrong".
- When seeking support for a backtest; please attach the backtest don't copy and paste the code.
- The 31st December 2017 is a Sunday.
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Forum TD
I thought, the highlited part in the screenshot would indicate the mis-calculation at first sight.
To be more precise: I‘ve defined two holding periods: the first one is February and March 2017 and the second holding period is November and December 2017. Between April and September, there is no holding period. But the backtesting does not care about that.
@Jared: Thanks for the hint that I have to check for weekends for myself and for the hint to paste the backtesting and not the code: Here is my backtest attached.
Quant Trader
I believe setholdings is for 0-100 percent. maybe -1 is not understood. Try self.Liquidate("BAC") instead.
Jared Broad
Ahh thank you for clarifying Quant Trader !
Forum TD -- Liquidate or SetHoldings(0). SetHoldings(-1) will make your algorithm go short.
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Forum TD
Fantastic! That's excellent! SetHoldings(1) and SetHoldings(0) provides perfect results for me.
Many thanks!
Pierre Pohly
I dont understand how u can open a short sell position? SetHoldings(-1) is not working and Sell(200) will also just sell your open positions. So how can u open a sell position?Â
Derek Melchin
Hi Pierre,
To request a short position using `SetHoldings`, we need to pass the symbol as the first argument. For example,
SetHoldings("TSLA", -1);
If this doesn't solve the issue, can you attach a backtest so I can investigate further?
Best,
Derek Melchin
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Forum TD
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
To unlock posting to the community forums please complete at least 30% of Boot Camp.
You can continue your Boot Camp training progress from the terminal. We hope to see you in the community soon!