I can't seem to access the Top Of Book (Aka, level 1) data at a given instant with my code.
The logs show that for each Bid/AskSize quote I receive, either the BidSize field or the Ask Size field is left empty. The tick only contains the most recent order and doesn't show the current best orders. Does anyone know how I can fix this and get access to the current Top Of Book data?
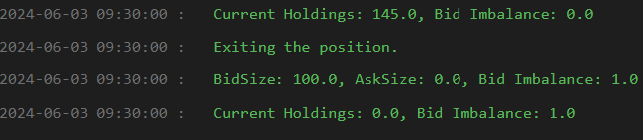
from AlgorithmImports import *
class BidAskImbalanceAlgorithm(QCAlgorithm):
def Initialize(self):
self.SetStartDate(2024, 6, 1) # Set Start Date
self.SetEndDate(2024, 6, 10) # Set End Date
self.SetCash(100000) # Set Strategy Cash
self.symbol = self.AddEquity("AMD", Resolution.Tick).Symbol
self.target_percentage = 0.25
self.imbalance_threshold = 0.7
def OnData(self, data):
if self.symbol not in data.Ticks:
return
ticks = data.Ticks[self.symbol]
for tick in ticks:
if tick.TickType != TickType.Quote:
continue
bid_size = tick.BidSize
ask_size = tick.AskSize
total_size = bid_size + ask_size
if total_size == 0:
self.Debug(f"Total size is zero. Bid: {bid_size}, Ask: {ask_size}")
continue
bid_imbalance = bid_size / total_size
self.Debug(f"BidSize: {bid_size}, AskSize: {ask_size}, Bid Imbalance: {bid_imbalance}")
holdings = self.Portfolio[self.symbol].Quantity
self.Debug(f"Current Holdings: {holdings}, Bid Imbalance: {bid_imbalance}")
if bid_imbalance > self.imbalance_threshold and holdings <= 0:
self.Debug("Entering a long position.")
self.SetHoldings(self.symbol, self.target_percentage)
elif bid_imbalance < self.imbalance_threshold and holdings > 0:
self.Debug("Exiting the position.")
self.Liquidate(self.symbol)
Mia Alissi
Sources: Handling Data - QuantConnect.com, Using Data - QuantConnect.com, US Equities - QuantConnect.com, Data Preparation - QuantConnect.com, US Equities - QuantConnect.com
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Caleb Eldredge
I don't understand Mia.
Caleb Eldredge
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
To unlock posting to the community forums please complete at least 30% of Boot Camp.
You can continue your Boot Camp training progress from the terminal. We hope to see you in the community soon!