I have an alpha model that sets the insight period with a `timedelta`.
Using a timedelta of 1 day, the flattening of positions happens (after 1 day) as expected.
However, when using a timedelta of 1 day - 1 second, the algorithm holds the position for a longer-than-expected period of time (shown below).
Looking at the debug, it looks like that the insights' `CloseTimeUtc` is incorrectly generated despite the `generatedTimeUtc` and `Period` look correct during runtime. What's going on here?
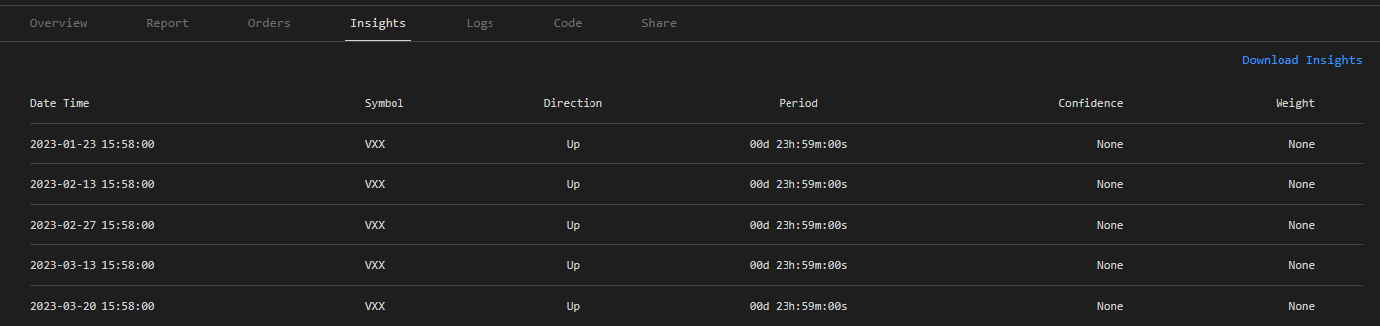
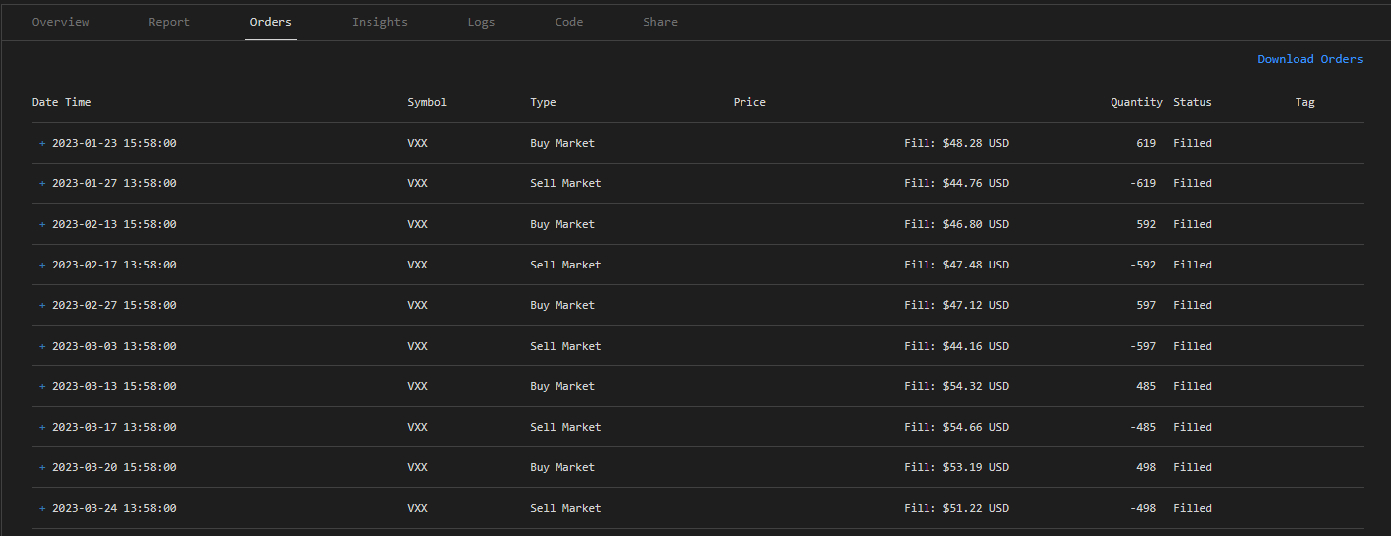
Below is a minimum reproduceable example:
from AlgorithmImports import *
from Portfolio.EqualWeightingPortfolioConstructionModel import EqualWeightingPortfolioConstructionModel
import functools
import pandas as pd
class InternFundReplica(QCAlgorithm):
def Initialize(self):
self.SetStartDate(2023, 1, 23)
self.SetCash(30000)
# params
tickers = ['VXX']
symbols = {ticker: Symbol.Create(ticker, SecurityType.Equity, Market.USA) for ticker in tickers}
# Algorithmic Framework
self.UniverseSettings.Resolution = Resolution.Minute
self.AddUniverseSelection(ManualUniverseSelectionModel(list(symbols.values())))
self.AddAlpha(TurnAroundTuesdayAlpha('turnaround_tuesday_alpha', 1.0))
self.SetPortfolioConstruction(EqualWeightingPortfolioConstructionModel())
self.SetExecution(ImmediateExecutionModel())
def OnData(self, data: Slice):
pass
class TurnAroundTuesdayAlpha(AlphaModel):
"""
If Monday is down, hold for tuesday
"""
def __init__(self, model_name: str, allocation: float):
super().__init__()
def Update(self, algorithm, data):
return []
def OnSecuritiesChanged(self, algorithm, changes):
for security in changes.AddedSecurities:
algorithm.Schedule.On(
algorithm.DateRules.Every(DayOfWeek.Monday),
algorithm.TimeRules.BeforeMarketClose(security.Symbol, 2),
functools.partial(self.monday_before_close, algorithm, security.Symbol)
)
def monday_before_close(self, algorithm, symbol):
trade_bars = list(algorithm.History[TradeBar](symbol, timedelta(days = 1), Resolution.Minute, extendedMarketHours = False))
todays_bars = [b for b in trade_bars if b.Time.date() == algorithm.Time.date()]
if len(todays_bars) > 0:
day_open = todays_bars[0].Open
latest_close = trade_bars[-1].Close
if latest_close < day_open * 0.995:
algorithm.EmitInsights(
Insight.Price(
symbol, timedelta(days = 1) - timedelta(seconds = 1),
direction = InsightDirection.Up
)
)
Thanks!
Mia Alissi
Based on the information provided in the extracts, it seems that the issue you're experiencing might be related to how the insight period is handled in the algorithm.
In QuantConnect, the insight period is a timedelta that shows how long your insight is valid for, and after that period, the position should be automatically closed. However, the actual behavior might vary depending on the resolution of your data and other factors.
One thing to note is that Python timedeltas can’t be specified to a specific number of months or years. So, if you're trying to set the insight period to a specific number of days minus one second, it might not behave as expected.
Also, an Up/Down insight will be liquidated if followed by a Flat insight with a duration equal to or exceeding the duration of the previous Up/Down insight.
If you need more fine control over your positions, you might want to consider using
SetHoldings
instead of emitting insights. This gives you more control over the precise points for market entry/exits.Unfortunately, without more information or the ability to run and debug your code, it's hard to provide a more specific answer. I would recommend checking the QuantConnect documentation and forums for more information on how insights and insight periods work.
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Robert Lee
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
To unlock posting to the community forums please complete at least 30% of Boot Camp.
You can continue your Boot Camp training progress from the terminal. We hope to see you in the community soon!