I have SPX index options trade data only. I'm trying to import the options first through an initialize function and then through OnData and neither one is returning the data.
from clr import AddReference
AddReference("System")
AddReference("QuantConnect.Algorithm")
AddReference("QuantConnect.Common")
from System import *
from System.Reflection import *
from decimal import Decimal
from enum import Enum
import calendar
from datetime import timedelta
import pandas as pd
import numpy as np
from QuantConnect import *
from QuantConnect.Parameters import *
from QuantConnect.Benchmarks import *
from QuantConnect.Brokerages import *
from QuantConnect.Util import *
from QuantConnect.Interfaces import *
from QuantConnect.Algorithm import *
from QuantConnect.Algorithm.Framework import *
from QuantConnect.Algorithm.Framework.Selection import *
from QuantConnect.Algorithm.Framework.Alphas import *
from QuantConnect.Algorithm.Framework.Portfolio import *
from QuantConnect.Algorithm.Framework.Execution import *
from QuantConnect.Algorithm.Framework.Risk import *
from QuantConnect.Indicators import *
from QuantConnect.Data import *
from QuantConnect.Data.Consolidators import *
from QuantConnect.Data.Custom import *
from QuantConnect.Data.Fundamental import *
from QuantConnect.Data.Market import *
from QuantConnect.Data.UniverseSelection import *
from QuantConnect.Notifications import *
from QuantConnect.Orders import *
from QuantConnect.Orders.Fees import *
from QuantConnect.Orders.Fills import *
from QuantConnect.Orders.Slippage import *
from QuantConnect.Scheduling import *
from QuantConnect.Securities import *
from QuantConnect.Securities.Equity import *
from QuantConnect.Securities.Forex import *
from QuantConnect.Securities.Interfaces import *
from datetime import date, datetime, timedelta
from QuantConnect.Python import *
from QuantConnect.Storage import *
QCAlgorithmFramework = QCAlgorithm
QCAlgorithmFrameworkBridge = QCAlgorithm
from QuantConnect.Securities.Option import OptionPriceModels
class SPXOptions(QCAlgorithm):
def Initialize(self):
self.bot = BOT()
self.SetStartDate(
self.bot.StartTime.year, self.bot.StartTime.month, self.bot.StartTime.day
) # Set Start Date
self.SetEndDate(
self.bot.EndTime.year, self.bot.EndTime.month, self.bot.EndTime.day
) # Set End Date
self.SetCash(self.bot.InitialCash) # Set Strategy Cash
self.bot.CurrentDate = self.bot.StartTime.date()
self.next_date = None
self.spx = self.AddIndex("SPX", Resolution.Minute).Symbol
self.contracts = []
self.Schedule.On(
self.DateRules.EveryDay(self._option.Symbol.Underlying),
self.TimeRules.AfterMarketOpen(self._option.Symbol.Underlying, 5),
Action(self.init_option_contracts),
)
def init_option_contracts(self):
contracts = list(
self.OptionChainProvider.GetOptionContractList(self.spx, self.Time)
)
price = self.Securities[self.spx].Price
self.Debug(self.Time)
lower_strike_bound = quantize(price - (self.bot.StrikePercent * price))
upper_strike_bound = quantize(price + (self.bot.StrikePercent * price))
puts = [i for i in contracts if i.ID.OptionRight == OptionRight.Put]
self.contracts = [
self.AddIndexOptionContract(put, Resolution.Minute) for put in puts
]
return
def option_filter_function(self, slice):
price = self.Securities[self.spx].Price
contracts = []
for kvp in slice.OptionChains:
if kvp.Key == self._option.Symbol:
contracts = [x for x in kvp.Value]
if len(contracts) < 5:
# self.Debug(f'{self.Time} :: not enough contracts: {len(contracts)}')
return
times_to_expiry = [(i.Expiry - self.Time).days for i in contracts]
self.Debug(f"{self.Time}\n{np.unique(times_to_expiry)}")
return
return
def OnData(self, slice):
self.bot.CurrentDate = self.Time.date()
self.bot.CurrentTime = self.Time.time()
if self.Portfolio.Invested:
return
if self.IsWarmingUp:
return
if not self.IsMarketOpen(self.spx):
return
# if len(self.contracts) < 1:
# self.Debug(f"{self.Time} - no optionchainprovider contracts yet")
# return
self.option_filter_function(slice)
return
The data structure looks like the following, within the local data folder:
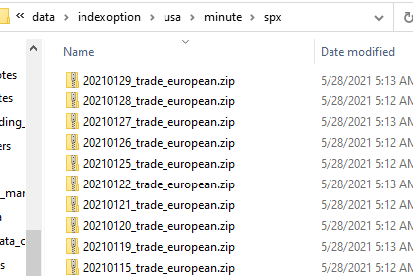
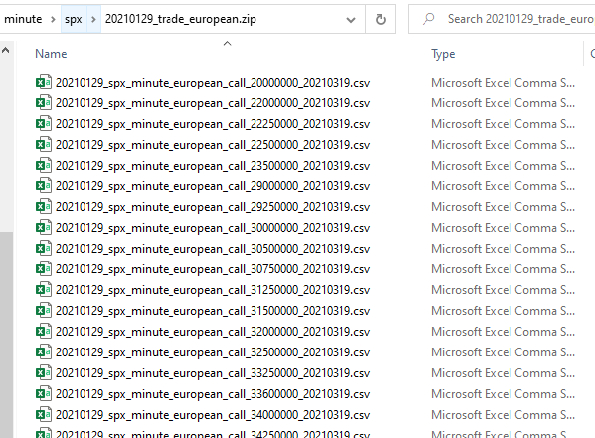
an example csv file within the zip looks like:
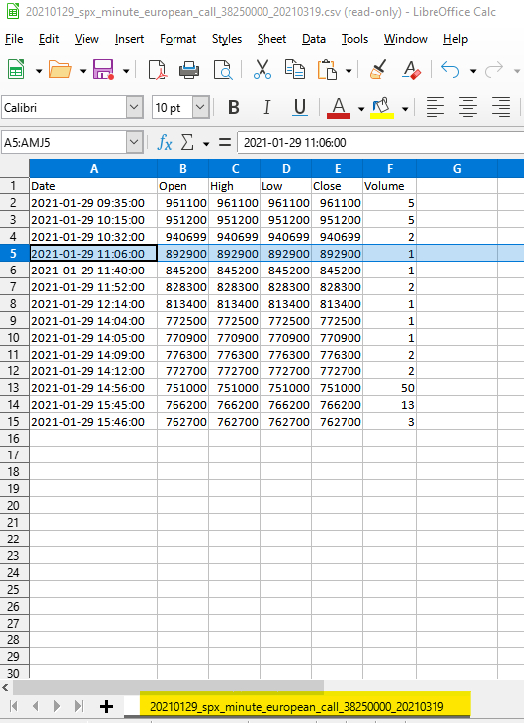
Not sure why the Option Data won't import. Please advise.
Derek Melchin
Hi Brian,
To subscribe to index options, we need to have data for the underlying index in place. Does the local file structure include data for SPX in Lean/Data/index/usa/minute/spx/?
Best,
Derek Melchin
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Brian Christopher
Derek Melchin yes.
Derek Melchin
Hi Brian,
We apologize for the delay. The issue may be from how the data files are formatted. The screenshot above of file "20210129_spx_minute_european_call_38250000_20210319.csv" has column headers and the timestamp is in the following format: yyyy-mm-dd hh-mm-ss. In contrast, the sample index option data in our GitHub repo doesn't have any headers and the timestamp is in the format of milliseconds since midnight.
We were able to run a local backtest using the sample index option data and place orders without error. See the attached backtest for reference.
Best,
Derek Melchin
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Brian Christopher
Hi @derek-melchin ,
I ran the exact same script as you did using the example data except I used only the IndexOption trade data (as that is all I have) and I received the following error:
I previously had asked the support team if the indexoption quote data was required but I wasn't given a clear answer. Please advise.
Louis Szeto
Hi Brian
Sorry for the long wait. The above error message is most likely related to not having the right date/time order.
Best,
Louis Szeto
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Brian Christopher
Louis Szeto I don't think that's it as I used the QC example data not my own.
Louis Szeto
Hi Brian
We are not able to reproduce your problem. Could you please attach a backtest that would reproduce your problem for further investigation?
Best,
Louis Szeto
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Brian Christopher
Louis Szeto I used the exact same code as Derek Melchin
This is the file folder setup using your original example data without OI and Quote data. The error is gone now, but the Index Option does not trade.
Louis Szeto
Hi Brian
May we ask what other files are inside your spx directory? If 20210114_quote_european.zip is not available, no trade will be placed.
Best,
Louis Szeto
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
Brian Christopher
Louis Szeto thanks. that answers my question. I need the quote data also. We only have the trade data at the moment.
Brian Christopher
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
To unlock posting to the community forums please complete at least 30% of Boot Camp.
You can continue your Boot Camp training progress from the terminal. We hope to see you in the community soon!