from QuantConnect import Resolution
from QuantConnect.Algorithm import QCAlgorithm
from clr import AddReference
AddReference("System")
AddReference("QuantConnect.Algorithm")
AddReference("QuantConnect.Indicators")
AddReference("QuantConnect.Common")
from System import *
from QuantConnect import *
from QuantConnect.Algorithm import *
from QuantConnect.Indicators import *
from QuantConnect.Data.Custom import *
from QuantConnect.Python import *
from datetime import datetime, timedelta
from QuantConnect.Data import SubscriptionDataSource
class Malgorithm(QCAlgorithm):
def Initialize(self):
self.SetStartDate(1999, 1, 1) # Set Start Date
self.SetEndDate(2004, 1, 1)
self.SetCash(100000) # Set Strategy Cash
# self.AddEquity("SPY", Resolution.Minute)
self.spx = self.AddData(SPX, "SPX", Resolution.Daily).Symbol
def OnData(self, data):
'''OnData event is the primary entry point for your algorithm. Each new data point will be pumped in here.
Arguments:
data: Slice object keyed by symbol containing the stock data
'''
# if not data.ContainsKey("SPX"): return
if not self.Portfolio.Invested:
self.SetHoldings(self.spx, 1)
self.SetHoldings(self.spx, -0.5)
self.Debug("Buying SPX ")
self.Debug("Time: {0} {1}".format(datetime.now(), "-----------------------------------------"))
class SPX(PythonData):
''' S&p 500 index data'''
def GetSource(self, config, date, isLive):
source = "C:\\Users\\anonymouse\\Documents\\CliLean\\P1\\sp500.csv"
print("Hello, backtesting logs!")
return SubscriptionDataSource(source, SubscriptionTransportMedium.RemoteFile);
def Reader(self, config, line, date, isLive):
# If first character is not digit, pass
if not (line.strip() and line[0].isdigit()): return None
data = line.split(',')
spx = SPX()
spx.Symbol = config.Symbol
spx.Time = datetime.strptime(data[0], "%Y-%m-%d") + timedelta(days=1) # Make sure we only get this data AFTER trading day - don't want forward bias.
spx.Value = decimal.Decimal(data[2])
return spx
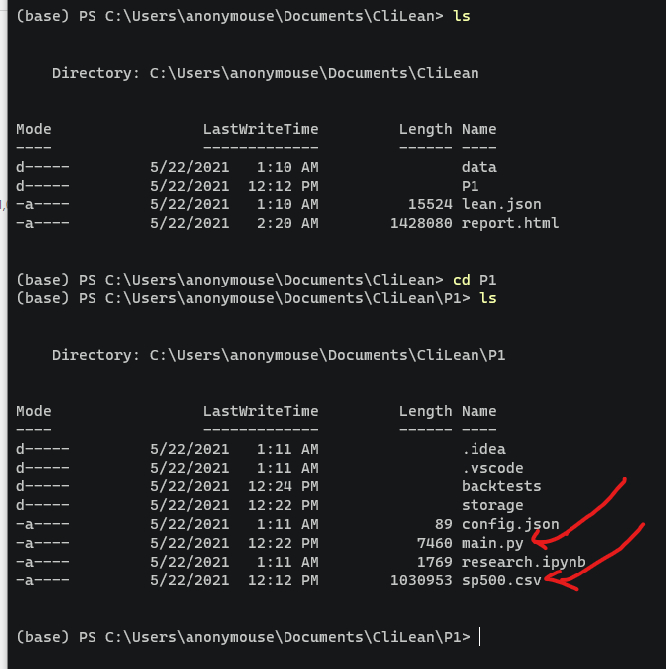
(base) PS C:\Users\anonymouse\Documents\CliLean> lean backtest "P1"
20210522 16:28:24.510 TRACE:: Config.GetValue(): debug-mode - Using default value: False
20210522 16:28:24.512 TRACE:: Config.Get(): Configuration key not found. Key: plugin-directory - Using default value:
20210522 16:28:24.559 TRACE:: Python for .NET Assembly: Python.Runtime, Version=2.0.1.0, Culture=neutral,
PublicKeyToken=5000fea6cba702dd
20210522 16:28:24.566 TRACE:: Python for .NET Assembly: nPython, Version=3.0.0.0, Culture=neutral, PublicKeyToken=null
20210522 16:28:24.593 TRACE:: Config.Get(): Configuration key not found. Key: data-directory - Using default value:
../../../Data/
20210522 16:28:24.596 TRACE:: Config.Get(): Configuration key not found. Key: version-id - Using default value:
20210522 16:28:24.596 TRACE:: Config.Get(): Configuration key not found. Key: cache-location - Using default value:
/Lean/Data
20210522 16:28:24.597 TRACE:: Engine.Main(): LEAN ALGORITHMIC TRADING ENGINE v2.5.0.0 Mode: DEBUG (64bit)
20210522 16:28:24.600 TRACE:: Engine.Main(): Started 4:28 PM
20210522 16:28:24.603 TRACE:: Config.Get(): Configuration key not found. Key: lean-manager-type - Using default value:
LocalLeanManager
20210522 16:28:24.618 TRACE:: JobQueue.NextJob(): Selected /LeanCLI/main.py
20210522 16:28:24.670 TRACE:: Config.GetValue(): scheduled-event-leaky-bucket-capacity - Using default value: 120
20210522 16:28:24.670 TRACE:: Config.GetValue(): scheduled-event-leaky-bucket-time-interval-minutes - Using default
value: 1440
20210522 16:28:24.670 TRACE:: Config.GetValue(): scheduled-event-leaky-bucket-refill-amount - Using default value: 18
20210522 16:28:24.672 TRACE:: Config.Get(): Configuration key not found. Key: algorithm-id - Using default value: main
20210522 16:28:24.675 TRACE:: Config.GetValue(): job-project-id - Using default value: 0
20210522 16:28:24.676 TRACE:: Config.Get(): Configuration key not found. Key: data-permission-manager - Using default
value: DataPermissionManager
20210522 16:28:24.686 TRACE:: AlgorithmManager.CreateTokenBucket(): Initializing LeakyBucket: Capacity: 120
RefillAmount: 18 TimeInterval: 1440
20210522 16:28:24.687 TRACE:: Config.GetValue(): algorithm-manager-time-loop-maximum - Using default value: 20
20210522 16:28:24.693 TRACE:: TextSubscriptionDataSourceReader.SetCacheSize(): Setting cache size to 71582788 items
20210522 16:28:24.961 TRACE:: Config.GetValue(): algorithm-creation-timeout - Using default value: 90
20210522 16:28:24.965 TRACE:: PythonInitializer.Initialize(): start...
PythonEngine.Initialize(): Runtime.Initialize()...
Runtime.Initialize(): Py_Initialize...
Runtime.Initialize(): PyEval_InitThreads...
Runtime.Initialize(): Initialize types...
Runtime.Initialize(): Initialize types end.
Runtime.Initialize(): AssemblyManager.Initialize()...
Runtime.Initialize(): AssemblyManager.UpdatePath()...
PythonEngine.Initialize(): GetCLRModule()...
PythonEngine.Initialize(): clr GetManifestResourceStream...
20210522 16:28:25.869 TRACE:: PythonInitializer.Initialize(): ended
20210522 16:28:25.872 TRACE:: AlgorithmPythonWrapper(): Python version 3.6.8 |Anaconda, Inc.| (default, Dec 30 2018,
01:25:33)
[GCC 7.3.0]: Importing python module main
20210522 16:28:26.116 TRACE:: AlgorithmPythonWrapper(): main successfully imported.
20210522 16:28:26.119 TRACE:: AlgorithmPythonWrapper(): Creating IAlgorithm instance.
20210522 16:28:26.597 TRACE:: Config.GetValue(): mute-python-library-logging - Using default value: True
20210522 16:28:26.610 TRACE:: LocalObjectStore.Initialize(): Storage Root: /Storage/QCAlgorithm
20210522 16:28:26.623 TRACE:: BacktestingSetupHandler.Setup(): Setting up job: Plan: Free, UID: 0, PID: 0, Version:
2.5.0.0, Source: WebIDE
20210522 16:28:26.627 TRACE:: Config.Get(): Configuration key not found. Key: security-data-feeds - Using default value:
20210522 16:28:26.726 TRACE:: BaseSetupHandler.SetupCurrencyConversions():
Symbol Quantity Conversion = Value in USD
USD: $ 100000.00 @ 1.00 = $100000.0
-------------------------------------------------
CashBook Total Value: $100000.0
20210522 16:28:26.728 TRACE:: SetUp Backtesting: User: 0 ProjectId: 0 AlgoId: main
20210522 16:28:26.729 TRACE:: Dates: Start: 01/01/1999 End: 01/01/2004 Cash: ¤100,000.00
20210522 16:28:26.731 TRACE:: BacktestingResultHandler(): Sample Period Set: 657.72
20210522 16:28:26.731 TRACE:: Time.TradeableDates(): Security Count: 1
20210522 16:28:26.734 TRACE:: Config.GetValue(): forward-console-messages - Using default value: True
20210522 16:28:26.735 TRACE:: JOB HANDLERS:
20210522 16:28:26.735 TRACE:: DataFeed: QuantConnect.Lean.Engine.DataFeeds.FileSystemDataFeed
20210522 16:28:26.736 TRACE:: Setup: QuantConnect.Lean.Engine.Setup.ConsoleSetupHandler
20210522 16:28:26.736 TRACE:: RealTime: QuantConnect.Lean.Engine.RealTime.BacktestingRealTimeHandler
20210522 16:28:26.736 TRACE:: Results: QuantConnect.Lean.Engine.Results.BacktestingResultHandler
20210522 16:28:26.736 TRACE:: Transactions:
QuantConnect.Lean.Engine.TransactionHandlers.BacktestingTransactionHandler
20210522 16:28:26.737 TRACE:: Alpha: QuantConnect.Lean.Engine.Alphas.DefaultAlphaHandler
20210522 16:28:26.737 TRACE:: ObjectStore: QuantConnect.Lean.Engine.Storage.LocalObjectStore
20210522 16:28:26.737 TRACE:: History Provider:
QuantConnect.Lean.Engine.HistoricalData.SubscriptionDataReaderHistoryProvider
20210522 16:28:26.748 TRACE:: AlgorithmManager.Run(): Begin DataStream - Start: 1/1/1999 12:00:00 AM Stop: 1/1/2004
11:59:59 PM
20210522 16:28:26.771 TRACE:: Debug: Launching analysis for main with LEAN Engine v2.5.0.0
20210522 16:28:26.783 TRACE:: Config.GetValue(): data-feed-max-work-weight - Using default value: 400
20210522 16:28:26.783 TRACE:: Config.GetValue(): data-feed-workers-count - Using default value: 16
20210522 16:28:26.784 TRACE:: WeightedWorkScheduler(): will use 16 workers and MaxWorkWeight is 400
20210522 16:28:26.795 TRACE:: Config.GetValue(): show-missing-data-logs - Using default value: False
20210522 16:28:26.895 TRACE:: UniverseSelection.AddPendingInternalDataFeeds(): Adding internal benchmark data feed
SPY,SPY,Hour,TradeBar,Trade,Adjusted,Internal
20210522 16:28:27.283 TRACE:: Synchronizer.GetEnumerator(): Exited thread.
20210522 16:28:27.283 TRACE:: AlgorithmManager.Run(): Firing On End Of Algorithm...
20210522 16:28:27.285 TRACE:: Engine.Run(): Exiting Algorithm Manager
20210522 16:28:27.287 TRACE:: FileSystemDataFeed.Exit(): Start. Setting cancellation token...
20210522 16:28:27.289 TRACE:: FileSystemDataFeed.Exit(): Exit Finished.
20210522 16:28:27.289 TRACE:: DefaultAlphaHandler.Exit(): Exiting...
20210522 16:28:27.293 TRACE:: DefaultAlphaHandler.Exit(): Ended
20210522 16:28:27.293 TRACE:: BacktestingResultHandler.Exit(): starting...
20210522 16:28:27.293 TRACE:: BacktestingResultHandler.Exit(): Saving logs...
20210522 16:28:27.296 TRACE:: Debug: Algorithm Id:(main) completed in 0.55 seconds at 16k data points per second.
Processing total of 8,757 data points.
20210522 16:28:27.297 TRACE:: StopSafely(): waiting for 'Result Thread' thread to stop...
20210522 16:28:27.297 TRACE:: Debug: Your log was successfully created and can be retrieved from: /Results/main-log.txt
20210522 16:28:27.297 TRACE:: BacktestingResultHandler.Run(): Ending Thread...
20210522 16:28:27.595 TRACE:: Config.GetValue(): regression-update-statistics - Using default value: False
20210522 16:28:27.595 TRACE::
STATISTICS:: Total Trades 0
STATISTICS:: Average Win 0%
STATISTICS:: Average Loss 0%
STATISTICS:: Compounding Annual Return 0%
STATISTICS:: Drawdown 0%
STATISTICS:: Expectancy 0
STATISTICS:: Net Profit 0%
STATISTICS:: Sharpe Ratio 0
STATISTICS:: Probabilistic Sharpe Ratio 0%
STATISTICS:: Loss Rate 0%
STATISTICS:: Win Rate 0%
STATISTICS:: Profit-Loss Ratio 0
STATISTICS:: Alpha 0
STATISTICS:: Beta 0
STATISTICS:: Annual Standard Deviation 0
STATISTICS:: Annual Variance 0
STATISTICS:: Information Ratio -0.074
STATISTICS:: Tracking Error 0.213
STATISTICS:: Treynor Ratio 0
STATISTICS:: Estimated Strategy Capacity $0
STATISTICS:: Lowest Capacity Asset
STATISTICS:: Fitness Score 0
STATISTICS:: Kelly Criterion Estimate 0
STATISTICS:: Kelly Criterion Probability Value 0
STATISTICS:: Sortino Ratio 79228162514264337593543950335
STATISTICS:: Return Over Maximum Drawdown 79228162514264337593543950335
STATISTICS:: Portfolio Turnover 0
STATISTICS:: Total Insights Generated 0
STATISTICS:: Total Insights Closed 0
STATISTICS:: Total Insights Analysis Completed 0
STATISTICS:: Long Insight Count 0
STATISTICS:: Short Insight Count 0
STATISTICS:: Long/Short Ratio 100%
STATISTICS:: Estimated Monthly Alpha Value $0
STATISTICS:: Total Accumulated Estimated Alpha Value $0
STATISTICS:: Mean Population Estimated Insight Value $0
STATISTICS:: Mean Population Direction 0%
STATISTICS:: Mean Population Magnitude 0%
STATISTICS:: Rolling Averaged Population Direction 0%
STATISTICS:: Rolling Averaged Population Magnitude 0%
STATISTICS:: OrderListHash d41d8cd98f00b204e9800998ecf8427e
20210522 16:28:27.595 TRACE:: BacktestingResultHandler.SendAnalysisResult(): Processed final packet
20210522 16:28:27.596 TRACE:: Engine.Run(): Disconnecting from brokerage...
20210522 16:28:27.596 TRACE:: Engine.Run(): Disposing of setup handler...
20210522 16:28:27.597 TRACE:: Engine.Main(): Analysis Completed and Results Posted.
Engine.Main(): Analysis Complete.
20210522 16:28:27.597 TRACE:: Engine.Main(): Packet removed from queue: main
20210522 16:28:27.598 TRACE:: LeanEngineSystemHandlers.Dispose(): start...
20210522 16:28:27.598 TRACE:: LeanEngineSystemHandlers.Dispose(): Disposed of system handlers.
20210522 16:28:27.598 TRACE:: LeanEngineAlgorithmHandlers.Dispose(): start...
20210522 16:28:27.603 TRACE:: LeanEngineAlgorithmHandlers.Dispose(): Disposed of algorithm handlers.
20210522 16:28:27.604 TRACE:: Program.Main(): Exiting Lean...
Successfully ran 'P1' in the 'backtesting' environment and stored the output in 'P1\backtests\2021-05-22_12-28-23'
(base) PS C:\Users\anonymouse\Documents\CliLean>
Main-Log
1999-01-01 00:00:00 Launching analysis for main with LEAN Engine v2.5.0.0
2003-12-31 16:00:00 Algorithm Id:(main) completed in 0.55 seconds at 16k data points per second. Processing total of 8,757 data points.
Rushi Chaudhari
It doesn't work without Globals.DataFolder at line47. I think that was the issue and SubscriptionTransportMedium.LocalFile.
Rushi Chaudhari
The material on this website is provided for informational purposes only and does not constitute an offer to sell, a solicitation to buy, or a recommendation or endorsement for any security or strategy, nor does it constitute an offer to provide investment advisory services by QuantConnect. In addition, the material offers no opinion with respect to the suitability of any security or specific investment. QuantConnect makes no guarantees as to the accuracy or completeness of the views expressed in the website. The views are subject to change, and may have become unreliable for various reasons, including changes in market conditions or economic circumstances. All investments involve risk, including loss of principal. You should consult with an investment professional before making any investment decisions.
To unlock posting to the community forums please complete at least 30% of Boot Camp.
You can continue your Boot Camp training progress from the terminal. We hope to see you in the community soon!