About US Future Options
The US Future Options dataset by AlgoSeek provides Option data on US Future contracts, including prices, strikes, and expires. The data covers 16 Monthly Future contracts, starts in January 2012, and is delivered on a minute frequency. This dataset is created by monitoring the trading activity on the CME, CBOT, NYMEX, and COMEX markets.
This dataset depends on the US Futures dataset because the US Futures dataset contains data on the underlying Futures contracts. This dataset also depends on the US Futures Security Master because the US Futures Security Master dataset contains information to construct continuous Futures.
About AlgoSeek
AlgoSeek is a leading historical intraday US market data provider offering the most comprehensive and detailed market data and analytics products in the financial industry covering equities, futures, options, cash forex, and cryptocurrencies. AlgoSeek data is built for quantitative trading and machine learning. For more information about AlgoSeek, visit algoseek.com.
About QuantConnect
QuantConnect was founded in 2012 to serve quants everywhere with the best possible algorithmic trading technology. Seeking to disrupt a notoriously closed-source industry, QuantConnect takes a radically open-source approach to algorithmic trading. Through the QuantConnect web platform, more than 50,000 quants are served every month.
Algorithm Example
from AlgorithmImports import *
class FutureOptionExampleAlgorithm(QCAlgorithm):
def initialize(self) -> None:
# Subscribe the underlying since the updated price is needed for filtering
self.underlying = self.add_future(Futures.Indices.SP_500_E_MINI,
extended_market_hours=True,
data_mapping_mode=DataMappingMode.OPEN_INTEREST,
data_normalization_mode=DataNormalizationMode.BACKWARDS_RATIO,
contract_depth_offset=0)
# Filter the underlying continuous Futures to narrow the FOP spectrum
self.underlying.set_filter(0, 182)
# Filter for the current-week-expiring calls to formulate a covered call that expires at the end of week
self.add_future_option(self.underlying.symbol, lambda u: u.include_weeklys().calls_only().expiration(0, 5))
def on_data(self, slice: Slice) -> None:
# Create canonical symbol for the mapped future contract, since option chains are mapped by canonical symbol
symbol = Symbol.create_canonical_option(self.underlying.mapped)
# Get option chain data for the mapped future, as both the underlying and FOP have the highest liquidity among all other contracts
chain = slice.option_chains.get(symbol)
if not self.portfolio.invested and chain:
# Obtain the ATM call that expires at the end of week, such that both underlying and the FOP expires the same time
expiry = max(x.expiry for x in chain)
atm_call = sorted([x for x in chain if x.expiry == expiry],
key=lambda x: abs(x.strike - x.underlying_last_price))[0]
# Use abstraction method to order a covered call to avoid manual error
option_strategy = OptionStrategies.covered_call(symbol, atm_call.strike,expiry)
self.buy(option_strategy, 1)
def on_securities_changed(self, changes: SecurityChanges) -> None:
for security in changes.added_securities:
if security.type == SecurityType.FUTURE_OPTION:
# Historical data
history = self.history(security.symbol, 10, Resolution.MINUTE)
self.debug(f"We got {len(history)} from our history request for {security.symbol}")
Example Applications
The US Future Options dataset enables you to accurately design Future Option strategies. Examples include the following strategies:
- Selling out of the money Future Option contracts to collect the premium that the Option buyer pays
- Buying put Options to hedge against downward price movement in Future contracts you bought
- Exploiting arbitrage opportunities that arise when the price of Option contracts deviate from their theoretical value
Pricing
Cloud Access
Free access to US Future Options from CME, COMEX, CBOT, and NYMEX on the QuantConnect Cloud platform for research, backtest, and live trading. Futures Options are available in minute resolution.
Explore Other Datasets
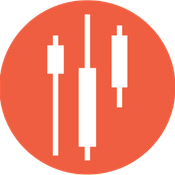
Upcoming Splits
Dataset by EOD Historical Data
US Futures
Dataset by AlgoSeek
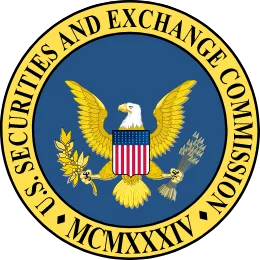
US SEC Filings
Dataset by Securities and Exchange Commission